一、快速入门步骤
1.导入jar包
下载struts2的jar包 struts-2.3.15.1-all 版本.
ps:在struts2开发,一般情况下最少导入的jar包,去apps下的struts2-blank示例程序中copy
2.创建index.jsp页面
创建hello.jsp页面。。。
3.对struts2框架进行配置
3.1.web.xml文件中配置前端控制器(核心控制器)-----就是一个Filter
目的:是为了让struts2框架可以运行。
<filter> <filter-name>struts2</filter-name> <filter-class>org.apache.struts2.dispatcher.ng.filter.StrutsPrepareAndExecuteFilter</filter-class> </filter> <filter-mapping> <filter-name>struts2</filter-name> <url-pattern>/*</url-pattern> </filter-mapping>
3.2.创建一个struts.xml配置文件 ,这个是struts2框架配置文件。
目的:是为了struts2框架流程可以执行。
名称:struts.xml
位置:src下(classes下)
4.创建一个HelloAction类
要求,在HelloAction类中创建一个返回值是String类型的方法,注意,无参数。
public String say(){
return "good";
}
5.在struts.xml文件中配置HelloAction
<package name="default" namespace="/" extends="struts-default"> <action name="hello" class="cn.itcast.action.HelloAction" method="say"> <result name="good">/hello.jsp</result> </action> </package>
6.在index.jsp中添加连接,测试
<a href="${pageContext.request.contextPath}/hello">第一次使用struts2</a>
在地址栏中输入:http://localhost/struts2_day01/index.jsp 访问连接,就可以看到
HelloAction类中的say方法执行了,也跳转到了hello.jsp
二、入门流程分析图:
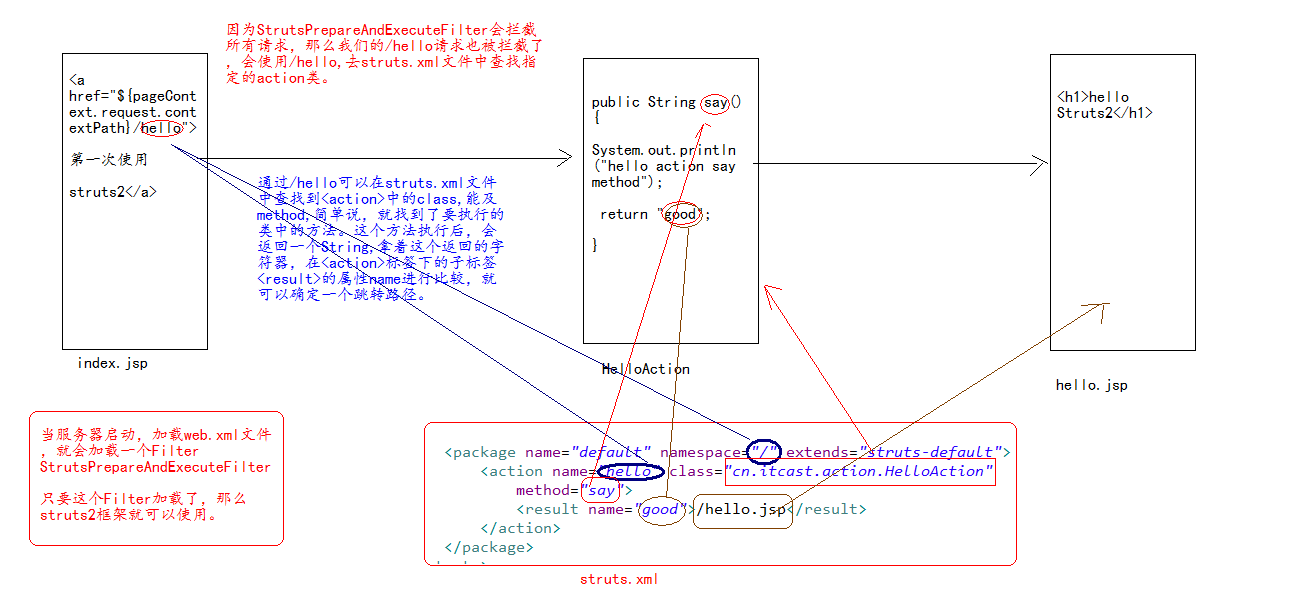
三、模仿struts2前端控制器—— StrutsPrepareAndExecuteFilter
1、web.xml

<?xml version="1.0" encoding="UTF-8"?> <web-app version="2.5" xmlns="http://java.sun.com/xml/ns/javaee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd"> <filter> <filter-name>struts</filter-name> <filter-class>cn.itcast.filter.StrutsFilter</filter-class> </filter> <filter-mapping> <filter-name>struts</filter-name> <url-pattern>/*</url-pattern> </filter-mapping> <welcome-file-list> <welcome-file>index.jsp</welcome-file> </welcome-file-list> </web-app>
2、StrutsFilter.java

package cn.itcast.filter; import java.io.File; import java.io.IOException; import java.lang.reflect.InvocationTargetException; import java.lang.reflect.Method; import javax.servlet.Filter; import javax.servlet.FilterChain; import javax.servlet.FilterConfig; import javax.servlet.ServletException; import javax.servlet.ServletRequest; import javax.servlet.ServletResponse; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import org.dom4j.Document; import org.dom4j.DocumentException; import org.dom4j.Element; import org.dom4j.io.SAXReader; public class StrutsFilter implements Filter { public void init(FilterConfig filterConfig) throws ServletException { } public void doFilter(ServletRequest req, ServletResponse resp, FilterChain chain) throws IOException, ServletException { // 1.强转 HttpServletRequest request = (HttpServletRequest) req; HttpServletResponse response = (HttpServletResponse) resp; // 2.操作 // 2.1 得到请求资源路径 String uri = request.getRequestURI(); String contextPath = request.getContextPath(); String path = uri.substring(contextPath.length() + 1); // System.out.println(path); // hello // 2.2 使用path去struts.xml文件中查找某一个<action name=path>这个标签 SAXReader reader = new SAXReader(); try { // 得到struts.xml文件的document对象。 Document document = reader.read(new File(this.getClass() .getResource("/struts.xml").getPath())); Element actionElement = (Element) document .selectSingleNode("//action[@name='" + path + "']"); // 查找<action // name='hello'>这样的标签 if (actionElement != null) { // 得到<action>标签上的class属性以及method属性 String className = actionElement.attributeValue("class"); // 得到了action类的名称 String methodName = actionElement.attributeValue("method");// 得到action类中的方法名称。 // 2.3通过反射,得到Class对象,得到Method对象 Class actionClass = Class.forName(className); Method method = actionClass.getDeclaredMethod(methodName); // 2.4 让method执行. String returnValue = (String) method.invoke(actionClass .newInstance()); // 是让action类中的方法执行,并获取方法的返回值。 // 2.5 // 使用returnValue去action下查找其子元素result的name属性值,与returnValue做对比。 Element resultElement = actionElement.element("result"); String nameValue = resultElement.attributeValue("name"); if (returnValue.equals(nameValue)) { // 2.6得到了要跳转的路径。 String skipPath = resultElement.getText(); // System.out.println(skipPath); request.getRequestDispatcher(skipPath).forward(request, response); return; } } } catch (DocumentException e) { e.printStackTrace(); } catch (ClassNotFoundException e) { e.printStackTrace(); } catch (NoSuchMethodException e) { e.printStackTrace(); } catch (SecurityException e) { e.printStackTrace(); } catch (IllegalAccessException e) { // TODO Auto-generated catch block e.printStackTrace(); } catch (IllegalArgumentException e) { // TODO Auto-generated catch block e.printStackTrace(); } catch (InvocationTargetException e) { // TODO Auto-generated catch block e.printStackTrace(); } catch (InstantiationException e) { // TODO Auto-generated catch block e.printStackTrace(); } // 3.放行 chain.doFilter(request, response); } public void destroy() { } }