1. 数据结构转换
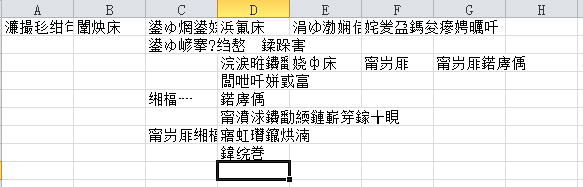
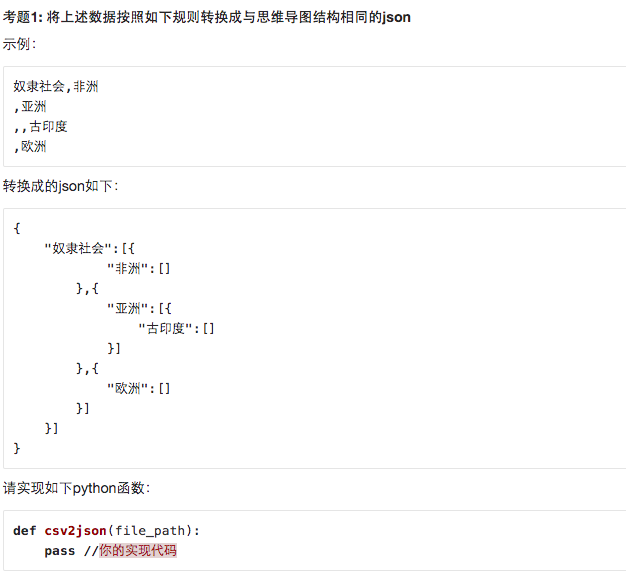
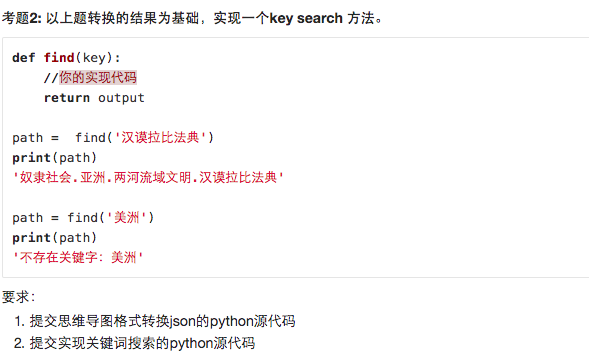
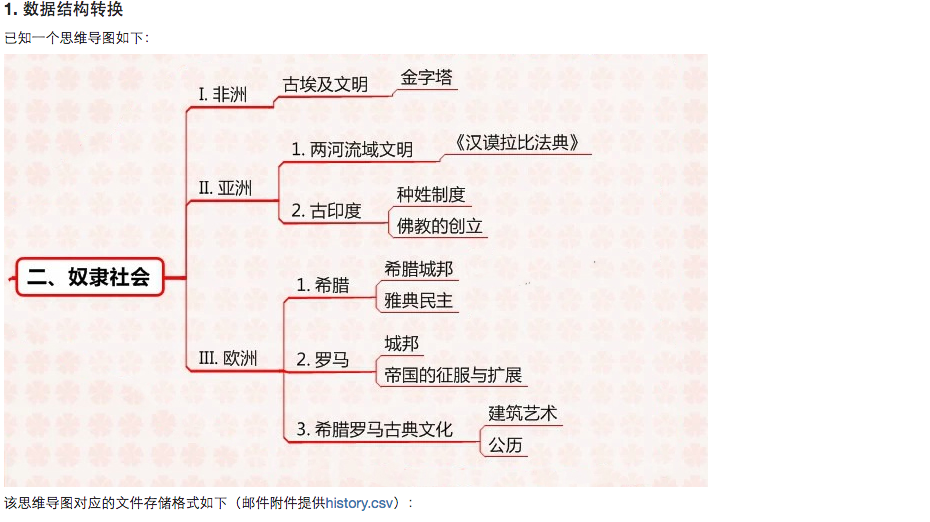
# -*- coding: utf-8 -*-
import csv
import json
def cvs2json(file_path):
with open(file_path, 'rb') as csvfile:
reader = csv.reader(csvfile)
reader_list = list(reader)
row_length = len(reader_list)
col_length = len(reader_list[0])
data = {}
for row_index in range(row_length):
sub = data
for col_index in range(col_length):
value = reader_list[row_index][col_index]
if value != '':
if value not in sub:
if col_index < col_length - 1:
sub[value] = [{}]
else:
sub[value] = []
if len(sub[value]) > 0:
sub = sub[value][0]
else:
tmp_row_index = row_index
while reader_list[tmp_row_index][col_index] == '':
tmp_row_index -= 1
sub = sub[reader_list[tmp_row_index][col_index]][0]
return json.dumps(data, encoding="UTF-8", ensure_ascii=False,
indent=4, separators=(',', ': '))
# -*- coding: utf-8 -*-
def find(obj_data, key, res):
def _inner(obj_data, key, res):
found = False
for i in obj_data:
if key not in res:
res.append(i)
if i == key:
found = True
return found
if not found and len(obj_data[i]) > 0:
for j in obj_data[i]:
found = _inner(j, key, res)
if not found:
res.pop()
return found
_inner(obj_data, key, res)
if len(res) == 0:
return u'不存在关键字:{0}'.format(key)
return '.'.join(res)
2. 用户权限验证系统
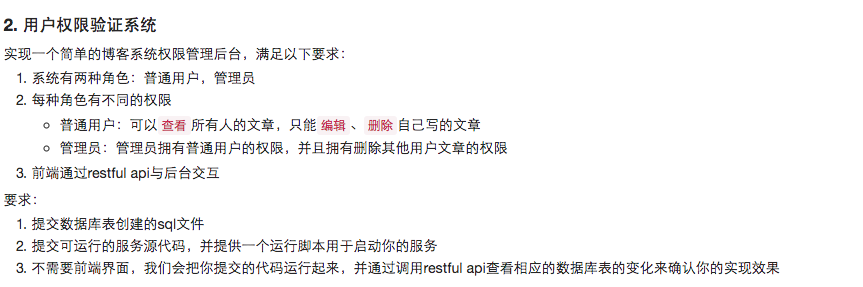