第一种哦通过ConfigurationProperties 指定一个yaml文件
新建Pojo实体包
@Component//组件
public class Dog {
@Value("狗子")
private String name;
@Value("11")
private Integer age;
}
@Component
@ConfigurationProperties(prefix = "person")//他的前缀为person
public class Person {
private String name;
private Integer age;
private Boolean happy;
private Date birth;
private Map<String,Object> maps;
private List<Object> lists;
private Dog dog;
}
新建application.yaml
yaml语法学习教程:https://www.runoob.com/w3cnote/yaml-intro.html
person:
name: 小狗
age: 12
happy: false
birth: 2022/11/02
maps: {k:v,k1:v1}
lists:
- code
- music
- readBook
- watch
dog:
name: 旺财
age: 12
在通过Person类上设置一个ConfigurationProperties
1.他是SpringBoot包下的
2.如果在实体类上定义这个注解,这个类上会让你打开一个连接
https://docs.spring.io/spring-boot/docs/2.1.9.RELEASE/reference/html/configuration-metadata.html#configuration-metadata-annotation-processor
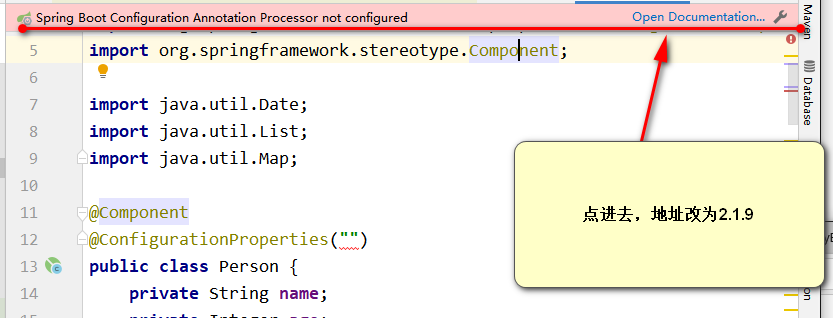
测试
@SpringBootTest
class Springboot01HelloworldApplicationTests {
@Autowired
private Person person;
@Test
void contextLoads() {
System.out.println(person);
}
}
结果
// Person{
// name='小狗',
// age=12,
// happy=false,
// birth=Wed Nov 02 00:00:00 CST 2022,
// maps=null,
// lists=null,
// dog=Dog{name='旺财', age=12}}
第二种指定配置文件
新建一个properties
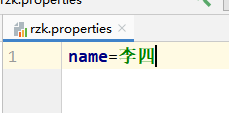
Person
@Component
@PropertySource(value = "classpath:rzk.properties")//指定配置文件
public class Person {
@Value("${name}")//赋值
private String name;
private Integer age;
private Boolean happy;
private Date birth;
private Map<String,Object> maps;
private List<Object> lists;
private Dog dog;
}
测试结果
Person{name='李四', age=null, happy=null, birth=null, maps=null, lists=null, dog=null}
@ConfigurationProperties作用
/**
* @ConfigurationProperties作用:
* 将配置文件中配置的每一个属性的值,映射到这个组件中;
* 告诉SpringBoot将本类中的所有属性和配置文件中相关的配置进行绑定
* 参数 prefix = "person" ,将配置文件中的person下面所有属性一一对应
*
* 只有这个组件是容器中的组件,才能使用容器提供的@ConfigurationProperties功能
*/
Thymeleaf使用foreach
导入依赖
<!--Thymeleaf-->
<dependency>
<groupId>org.thymeleaf</groupId>
<artifactId>thymeleaf-spring5</artifactId>
</dependency>
<dependency>
<groupId>org.thymeleaf.extras</groupId>
<artifactId>thymeleaf-extras-java8time</artifactId>
</dependency>
新建一个test.html
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<h1 th:text="${msg}"></h1>
<h1 th:each="user : ${users}" th:text="${user}"></h1>
</body>
</html>
controller
@Controller
public class HelloController {
@RequestMapping("/hello")
public String hello(Model model){
model.addAttribute("users", Arrays.asList("李四","赵五","王七"));
return "test";
}
}
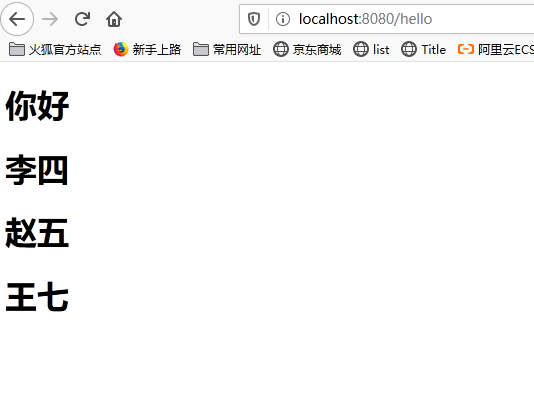