forEach()
作用:
forEach()显示的指定一个处理函数,在这个函数里,会依次遍历数组中的元素,可以对每个元素进行处理,请注意方法对数组的每个元素执行一次给定的函数
类似最普通的for(var i=0; i<arr1.length; i++){},只不过用forEach后不用写的那么啰嗦。
特别注意的是:forEach只可按需对调用的该数组每个元素进行处理,改造,是可对原数组的按需改变,回调函数并不返回新数组,回调函数handleFunc显示的返回值也不生成新数组
适应场景:
需要对调用数组进行相关值处理,改变这个数组或只拿调用数组做执行判断依据时使用
语法、代码示例:
指定一个function对数组arr1进行改造处理
1 const arr1 = ['a', 'b', 'c']; 2 const handleFunc = (item,index, arr) => { 3 console.log(item) 4 console.log(index) 5 console.log(arr) 6 // 其它处理逻辑 7 } 8 const arr2 = arr1.forEach(handleFunc); 9 console.log(arr2) // undefined
代码输出:
可以看到arr2值为 undefined arr1.forEach()不返回新数组或原数组
filter()
作用:
方法会创建并返回一个新数组,这个新数组的数据为filter中指定的回调函数handleFunc中设定的符合指定条件的原数组新的子集。
适应场景:
现有一个数组数据A,项目中某个功能需要对数组A的数据进行依次判断,返回符合条件的新数组 这样的需要就非常适合用到filter()来处理。
语法、代码示例:
代码演示A:
为了便于比较filter跟forEach的差异,这里只对forEach的演示代码做2处简单的更改或增加。可以明显的感觉到filter与forEach的不同。
1 const arr1 = ['a', 'b', 'c']; 2 const handleFunc = (item,index, arr) => { 3 console.log(item) 4 console.log(index) 5 console.log(arr) 6 // 其它处理逻辑 7 8 return item === 'a' // 改动1 + 9 } 10 const arr2 = arr1.filter(handleFunc); // 改动2:forEach改为filter 11 12 console.log(arr2) // ["a"]
代码输出:
特别注意:filter的返回值是一个新的数组,而这个新数组就是filter()指定的回调函数handleFunc的显示返回值(即对数组单项数据符合自定义条件的累加)
如果回调函数handleFunc不显示的指定返回数据,那么filter()的返回值为空数组[]。
代码演示B:
为了更好的加深filter印象我们再来一个demo,项目中有个需求:当用户选择小于100元以下商品时,只显示价格小于100的商品,那么就需要我们针对现有商品数据过滤掉大于100的数据。
1 const arr1 = [ 2 { 3 id: 1001, 4 title: '商品A', 5 price: 80 6 }, 7 { 8 id: 1002, 9 title: '商品B', 10 price: 90 11 }, 12 { 13 id: 1003, 14 title: '商品C', 15 price: 101 16 }, 17 { 18 id: 1004, 19 title: '商品D', 20 price: 118 21 } 22 ]; 23 const handleFunc = (item,index, arr) => { 24 return item.price < 100 // 需要返回的数据要符合这个条件,自动返回所有单项数据符合这个条件的累加数组 25 } 26 const arr2 = arr1.filter(handleFunc); 27 28 console.log(arr2); // 返回handleFunc 返回的符合条件的新数组数据
代码输出:
小结:filter的方便在于相较于过去没有filter的时候我们处理类似的问题,需要写普通的for循环做遍历判断处理,每个人写可能还会有各不同,而filter通过指定规则的handleFunc,
固定的使用方式,我们只需关注符合条件的逻辑判断条件的书写,大大减少了各色代码的书写,使得工程师关注点更专注。
some()
作用:
有没有符合自定义条件的至少1个的数据(也就是只要有一个数据符合条件那么 some的结果就返回true)
适应场景:
语法、代码示例:
相较于filter的“代码演示B” 只做了一处更改,就是,将filter改为some,其它完全不变,同样可以直观感受它们的区别。
1 const arr1 = [ 2 { 3 id: 1001, 4 title: '商品A', 5 price: 80 6 }, 7 { 8 id: 1002, 9 title: '商品B', 10 price: 90 11 }, 12 { 13 id: 1003, 14 title: '商品C', 15 price: 101 16 }, 17 { 18 id: 1004, 19 title: '商品D', 20 price: 118 21 } 22 ]; 23 const handleFunc = (item,index, arr) => { 24 return item.price < 100 // 也是要跟filter一样要回调函数要显示的返回,或用es6箭头函数 对单个表达式的默认返回 25 } 26 console.log(arr1.some(handleFunc)); // 修改 使用some方法
代码输出:
小结:就是只要有一个条件符合,那么回调函数结果就是true,否则就是false,值得注意的是回调函数必须显示的返回值 否则不论是有没有条件满足,
some的返回结果都是false
every()
作用:
指定一个回调函数给every,这个回调函数中判断数组每一项是否都满足自定义条件,注意是都满足,只有都满足条件最终every返回值则为true,否则,
但凡有一个不满足条件都返回false
通俗的讲:
看看这组商品价格都小于100吗,哦不,我看还有2个商品要大于100(此时every返回值为false)
语法、代码示例:
同样,相校于some的演示代码,我们只改了一处,即some方法改为every,其他代码完全与some演示代码一样,很容易感受出异同。
需求:以下商品价格都小于100吗
1 const arr1 = [ 2 { 3 id: 1001, 4 title: '商品A', 5 price: 80 6 }, 7 { 8 id: 1002, 9 title: '商品B', 10 price: 90 11 }, 12 { 13 id: 1003, 14 title: '商品C', 15 price: 101 16 }, 17 { 18 id: 1004, 19 title: '商品D', 20 price: 118 21 } 22 ]; 23 const handleFunc = (item,index, arr) => { 24 return item.price < 100 25 } 26 console.log(arr1.every(handleFunc)); // some改为every
小结:就是必须每一项条件都符合,那么回调函数结果就是true,否则就是false,值得注意的是回调函数也必须显示的返回值 否则不论即使条件都满足,
every的返回结果都是false
提示:
当然以上代码我们还可以这么写
1 const handleFunc = (item,index, arr) => { 2 return item.price < 100 3 }
我们改为一行写,也不是啥不得了的写法,就是ES6的简写,就是当返回的表达式只有单行时,可以省略掉大括号,以及箭头函数可以省略最后的return,因为这么写默认跟上加return效果一致:
1 const handleFunc = (item,index, arr) => item.price < 100
find()
作用:
find的回调函数会从上往下遍历数组的每一项是否符合diy的条件,看谁先符合就把哪个数组单项数据返回。一个符合条件的数组项都没有则返回undefined。
应用场景:
需要获取数组中越近符合条件数据的场景。
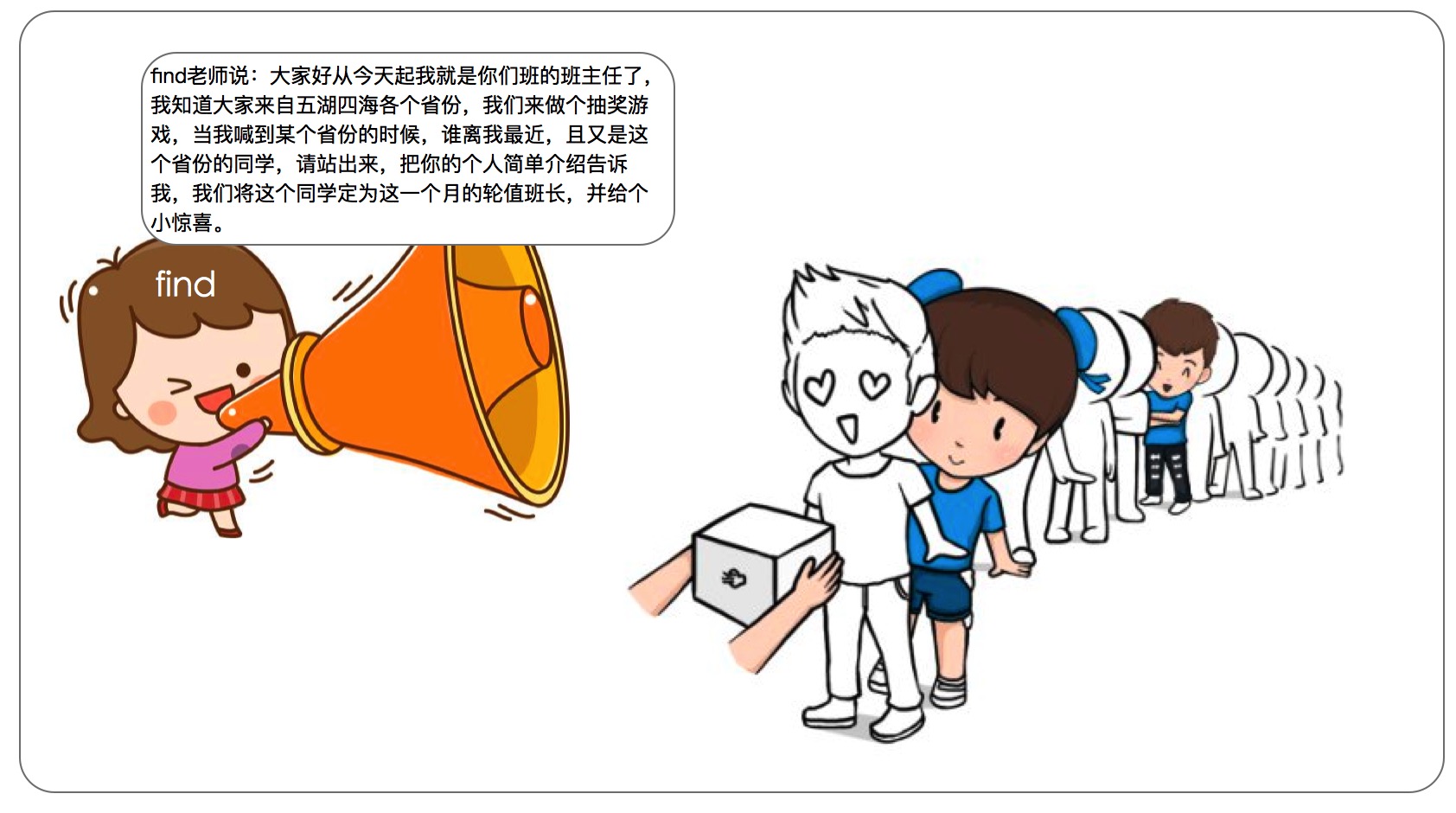
1 const arr1 = [ 2 { 3 id: 1001, 4 title: '商品A', 5 price: 80 6 }, 7 { 8 id: 1002, 9 title: '商品B', 10 price: 90 11 }, 12 { 13 id: 1003, 14 title: '商品C', 15 price: 101 16 }, 17 { 18 id: 1004, 19 title: '商品D', 20 price: 118 21 } 22 ]; 23 const handleFunc = (item,index, arr) => item.price < 100 24 console.log(arr1.find(handleFunc)); // 使用find方法,返回符合条件的最近数组单项的数据,没有符合则默认返回undefined
findIndex()
作用:
使用findIndex方法,返回符合条件的最近数组单项的数据的索引,没有符合则默认返回-1
跟find方法使用一样的demo,仅仅改为findIndex方法,很明显感知到跟find方法的区别
1 const arr1 = [ 2 { 3 id: 1001, 4 title: '商品A', 5 price: 80 6 }, 7 { 8 id: 1002, 9 title: '商品B', 10 price: 90 11 }, 12 { 13 id: 1003, 14 title: '商品C', 15 price: 101 16 }, 17 { 18 id: 1004, 19 title: '商品D', 20 price: 118 21 } 22 ]; 23 const handleFunc = (item,index, arr) => item.price < 100 24 console.log(arr1.findIndex(handleFunc)); // 使用findIndex方法,返回符合条件的最近数组单项的数据的索引,没有符合则默认返回-1
map()
作用:
对目标数据进行处理,并返回一个处理后的新数组
应用场景:
需要对原数组进行处理,根据diy处理规则返回新的数组数据
演示C:
1 const arr1 = [11,22,33]; 2 const handleFunc = (item,index, arr) => item * 100 3 console.log(arr1.map(handleFunc));
演示D:如果操作的目标数组每个元素都是对象型的,那么处理完毕后返回的新数组的单项数据项只包括return 时参与运算或逻辑判断的key
1 const arr1 = [ 2 { 3 id: 1001, 4 title: '商品A', 5 price: 80 6 }, 7 { 8 id: 1002, 9 title: '商品B', 10 price: 90 11 }, 12 { 13 id: 1003, 14 title: '商品C', 15 price: 101 16 }, 17 { 18 id: 1004, 19 title: '商品D', 20 price: 118 21 } 22 ]; 23 const handleFunc = (item,index, arr) => item.price * 100 24 console.log(arr1.map(handleFunc));
演示E:如果想在map处理后返回原数组项所有的key,可以这样
1 const arr1 = [ 2 { 3 id: 1001, 4 title: '商品A', 5 price: 80 6 }, 7 { 8 id: 1002, 9 title: '商品B', 10 price: 90 11 }, 12 { 13 id: 1003, 14 title: '商品C', 15 price: 101 16 }, 17 { 18 id: 1004, 19 title: '商品D', 20 price: 118 21 } 22 ]; 23 const handleFunc = (item,index, arr) => { 24 return { 25 id: item.id, // 不修改 26 title: item.title += '你好', // 修改标题 当然key title也可以改 27 price: item.price * 100 // 修改价格 28 } 29 } 30 console.log(arr1.map(handleFunc));
reduce()
作用:
可以对数组的每一项元素执行求和,回调函数有自己的固定写法,结果为数组每一项的总和。
适应场景:
计算数组每一项求和的情况
代码演示F:
1 const arr1 = [1, 2, 3, 4]; 2 const reducer = (accumulator, currentValue) => accumulator + currentValue; 3 4 // 1 + 2 + 3 + 4 5 console.log(arr1.reduce(reducer)); // 输出 10
代码演示G:
当然也可以这么写(只是上面的写法从参数变量语义上你能感觉到它的机制)
1 const arr1 = [1, 2, 3, 4]; 2 const reducer = (a, b) => a + b; 3 4 // 1 + 2 + 3 + 4 5 console.log(arr1.reduce(reducer)); // 输出 10