1.验证上传文件的类型:
(1)验证图片类型
<template>
<el-upload
class="avatar-uploader"
action="https://jsonplaceholder.typicode.com/posts/"
:show-file-list="false"
:on-success="handleAvatarSuccess"
:before-upload="beforeAvatarUpload"
>
<img v-if="imageUrl" :src="imageUrl" class="avatar">
<i v-else class="el-icon-plus avatar-uploader-icon"></i>
</el-upload>
</template>
<script>
handleAvatarSuccess (res, file) {
this.imageUrl = URL.createObjectURL(file.raw)
},
beforeAvatarUpload (file) {
const isIMG =
file.type === 'image/jpg' ||
file.type === 'image/jpeg' ||
file.type === 'image/png'
const isLt = file.size / 1024 / 50 <= 1
if (!isIMG) {
this.$message.error('上传头像图片只支持jpg、jpeg、png格式!')
}
if (!isLt) {
this.$message.error('上传头像图片大小不能超过50KB!')
}
return isLt && isIMG
}
</script>
(2)限制文件大小及其类型为压缩包
<el-button
size="small"
plain
class="btn-upload"
accept="application/x-zip-compressed"
>点击上传
</el-button>
methods: {
beforeAvatarUpload (file) {
let fileName = file.name
let pos = fileName.lastIndexOf('.')
let lastName = fileName.substring(pos, fileName.length)
if (
lastName.toLowerCase() !== '.zip' &&
lastName.toLowerCase() !== '.rar'
) {
this.$message.error('文件必须为.zip或者.rar类型')
// this.resetCompressData()
return
}
// 限制上传文件的大小
const isLt =
file.size / 1024 / 5 >= 1 && file.size / 1024 / 1024 / 100 <= 1
if (!isLt) {
this.$message.error('上传文件大小不得小于5KB,不得大于100MB!')
}
return isLt
}
}
(3)验证.txt文件也类似
<el-upload
class="upload-file"
style="display:inline-block;"
action="https://jsonplaceholder.typicode.com/posts/"
:show-file-list="false"
:before-upload="beforeAvatarUpload"
>
<el-button size="small" plain class="btn-upload" accept="text/txt">上传关键字</el-button>
</el-upload>
beforeAvatarUpload (file) {
let fileName = file.name
let pos = fileName.lastIndexOf('.')
let lastName = fileName.substring(pos, fileName.length)
if (lastName.toLowerCase() !== '.txt') {
this.$message.error('请上传.txt文件类型')
}
}
2.简单的表单验证处理
<el-form
ref="ruleForm"
:model="ruleForm"
:rules="rules"
label-width="100px" //设置输入框的宽度
size="small" //给定输入框的尺寸大小
class="form"
>
....
</el-form>
<script>
ruleForm: {
name: '',
landing: ''
},
rules: {
name: [
{ required: true, message: '请输入创意名称', trigger: 'blur' }, //required设置它为必填选项
{ min: 3, max: 5, message: '长度在3到5个字符', trigger: 'blur' }
],
landing: [
{ required: true, message: '请输入落地页', trigger: 'blur' },
{ min: 3, max: 5, message: '长度在3到5个字符', trigger: 'blur' }
]
}
</script>
3.回车提交内容
原生的input,使用 @keyup.enter就可以:
原生:<input v-model="form.name" placeholder="昵称" @keyup.enter="submit">
但使用element-ui,则要加上native限制符,因为element-ui把input进行了封装,原事件就不起作用了:
element-ui:
<el-input
type="text"
v-model="keyWord"
placeholder="请输入关键词,回车键(enter提交)"
@keyup.enter.native="submit">
</el-input>
submit () {
const isLength = this.keyWord.length
if (isLength > 30) {
this.$message.error('超长关键词无法添加!')
return
}
this.keyWord = '' //enter提交之后清空输入框的内容
}
注:取消则使用@submit.native.prevent:例<el-form :inline="true" @submit.native.prevent> </el-form> 取消输入框中按下回车提交该表单的默认行为
4.设置路由 this.$router.push({}):
例<el-button @click="cancel">取消</el-button>
cancel () {
this.$router.push({
path: '/customCrowd'
})
}
5. 禁用属性
:disabled="true"
注:在vue中this的使用:this是指整个当前的文档;使用vue框架时script中不能使用冒号;在script中的内容必须使用单引号不能使用双引号;定义函数方法时要注意留出一个空格;避免定义未用到的变量;建议单独建立一个全局的样式文档static/css/下,因为很多时候框架默认样式权值更大,直接在当前文件中设置css样式不起作用,但是要加上该文本的类名,避免影响其他区域;另外,style标签中scoped的作用是表明以下定义的内容只在该区域中生效
6.使用vue的watch监听数据传输中的变化
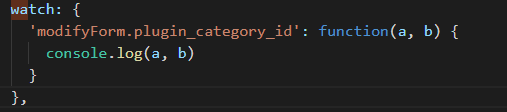
7.常见的分页问题处理bug
问题描述:第n页仅有一条数据,当删除这条数据时再一次请求数据列表,此时this.page的值仍然是n,但实际上此时应该发送的是n-1,因此需要做判断,利用删除该数据前的列表请求回来的total值减1,再对this.pageSize取天花板函数Math.ceil((_this.total - 1)/10)
8.监听输入字数 使用input事件