1] FND_FLEX_EXT.GET_COMBINATION_ID:
This API Finds combination_id for given set of key flexfield segment values. Segment values must be input in segments(1) – segments(n_segments) in the order displayed.
It also creates a new combination if it is valid and the flexfield allows dynamic inserts and the combination does not already exist. It commit the transaction soon after calling this function since if a combination is created it will prevent other users creating similar combinations on any flexfield until a commit is issued.
It performs all checks on values including security and cross-validation. Value security rules will be checked for the current user identified in the FND_GLOBAL package.
Generally pass in SYSDATE for validation date. If validation date is null, this function considers expired values valid and checks all cross-validation rules even if they are outdated.
This function returns TRUE if combination valid or FALSE and sets error message using FND_MESSAGE utility on error or if invalid. If this function returns FALSE, use GET_MESSAGE to get the text of the error message in the language of the database, or GET_ENCODED_MESSAGE to get the error message in a language-independent encoded format.
The Combination_id output may be NULL if combination is invalid.
1 SET serveroutput ON; 2 DECLARE 3 l_application_short_name VARCHAR2(240); 4 l_key_flex_code VARCHAR2(240); 5 l_structure_num NUMBER; 6 l_validation_date DATE; 7 n_segments NUMBER; 8 SEGMENTS APPS.FND_FLEX_EXT.SEGMENTARRAY; 9 l_combination_id NUMBER; 10 l_data_set NUMBER; 11 l_return BOOLEAN; 12 l_message VARCHAR2(240); 13 BEGIN 14 l_application_short_name := 'SQLGL'; 15 l_key_flex_code := 'GL#'; 16 SELECT id_flex_num 17 INTO l_structure_num 18 FROM apps.fnd_id_flex_structures 19 WHERE ID_FLEX_CODE = 'GL#' 20 AND ID_FLEX_STRUCTURE_CODE=<ACCOUNTING_FLEXFIELD>; 21 l_validation_date := SYSDATE; 22 n_segments := 6; 23 segments(1) := '00101'; 24 segments(2) := '28506'; 25 segments(3) := '00000'; 26 segments(4) := '09063'; 27 segments(5) := '00000'; 28 segments(6) := '00000'; 29 l_data_set := NULL; 30 l_return := FND_FLEX_EXT.GET_COMBINATION_ID( 31 application_short_name => l_application_short_name, 32 key_flex_code => l_key_flex_code, 33 structure_number => l_structure_num, 34 validation_date => l_validation_date, 35 n_segments => n_segments, 36 segments => segments, 37 combination_id => l_combination_id, 38 data_set => l_data_set); 39 l_message:= FND_FLEX_EXT.GET_MESSAGE; 40 IF l_return THEN 41 DBMS_OUTPUT.PUT_LINE('l_Return = TRUE'); 42 DBMS_OUTPUT.PUT_LINE('COMBINATION_ID = ' || l_combination_id); 43 ELSE DBMS_OUTPUT.PUT_LINE('Error: '||l_message); 44 END IF;
2] FND_FLEX_EXT.get_ccid:
This API gets combination id for the specified key flexfield segments.It is identical to get_combination_id() except this function takes segment values in a string concatenated by the segment delimiter for this flexfield, and returns a positive combination id if valid or 0 on error.。
1 Declare 2 v_ChartOfAccoutsId Number := 50268; --GL帐户键弹性域结构ID 3 v_AccountName Varchar2(2000) := '11.110822.4105160101.00.000000000000.000000.000000' --GL帐户 4 v_CodeCombinationId Number; --GL帐户ID 5 Begin 6 v_CodeCombinationId := Fnd_Flex_ext.get_ccid(application_short_name => 'SQLGL', --用应产品简称 7 key_flex_code => 'GL#', --键弹性域代码 8 structure_number => v_ChartOfAccoutsId, --键弹性域结构编号 9 validation_date => to_char(SYSDATE,'YYYY/MM/DD HH24:MI:SS'), --帐户有效日期 10 concatenated_segments => v_AccountName); -- 11 If (Nvl(v_CodeCombinationId, 0) = 0) Then 12 Dbms_Output.put_line('GL帐户组合失败'); 13 Else 14 Dbms_Output.put_line('获得GL帐户ID:' || v_CodeCombinationId); 15 End If; 16 End;
3] FND_FLEX_KEYVAL.VALIDATE_SEGS:
These key flexfields server validations API are a low level interface to key flexfields validation. They are designed to allow access to all the flexfields functionality, and to allow the user to get only the information they need in return. Because of their generality, these functions are more difficult to use than those in the FND_FLEX_EXT package. Oracle strongly suggests using the functions in FND_FLEX_EXT package if at all possible.
This function finds combination from given segment values. Segments are passed in as a concatenated string in increasing order of segment_number (display order).
Various Operations that can be performed are:
- ‘FIND_COMBINATION’ – Combination must already exist.所给定的组合字段必须在系统中已经被定义
- ‘CREATE_COMBINATION’ – Combination is created if doesn’t exist.如果组合字段不存在,则在系统中创建该组合
- ‘CREATE_COMB_NO_AT’ – same as create_combination but does not use an autonomous transaction.功能和CREATE_COMBINATION相同,只是不像前者要使用相对独立的子事务autonomous transaction
- ‘CHECK_COMBINATION’ – Checks if combination valid, doesn’t create.只是检查一下给定的组合字段是否合法,不管检查结果如何,都不创建新的组合
- ‘DEFAULT_COMBINATION’ – Returns minimal default combination.返回默认的最小组合
- ‘CHECK_SEGMENTS’ – Validates segments individually.分别验证字段
If validation date is NULL checks all cross-validation rules. It returns TRUE if combination valid or FALSE and sets error message on server if invalid. Use the default values if you do not want any special functionality.
1 SET serveroutput ON; 2 DECLARE 3 l_segment1 GL_CODE_COMBINATIONS.SEGMENT1%TYPE; 4 l_segment2 GL_CODE_COMBINATIONS.SEGMENT2%TYPE; 5 l_segment3 GL_CODE_COMBINATIONS.SEGMENT3%TYPE; 6 l_segment4 GL_CODE_COMBINATIONS.SEGMENT4%TYPE; 7 l_segment5 GL_CODE_COMBINATIONS.SEGMENT5%TYPE; 8 l_segment6 GL_CODE_COMBINATIONS.SEGMENT6%TYPE; 9 l_valid_combination BOOLEAN; 10 l_cr_combination BOOLEAN; 11 l_ccid GL_CODE_COMBINATIONS_KFV.code_combination_id%TYPE; 12 l_structure_num FND_ID_FLEX_STRUCTURES.ID_FLEX_NUM%TYPE; 13 l_conc_segs GL_CODE_COMBINATIONS_KFV.CONCATENATED_SEGMENTS%TYPE; 14 p_error_msg1 VARCHAR2(240); 15 p_error_msg2 VARCHAR2(240); 16 BEGIN 17 l_segment1 := '00101'; 18 l_segment2 := '28506'; 19 l_segment3 := '00000'; 20 l_segment4 := '14302'; 21 l_segment5 := '00455'; 22 l_segment6 := '00000'; 23 l_conc_segs := l_segment1 || '.' || l_segment2 || '.' || l_segment3 || '.' || 24 l_segment4 || '.' || l_segment5 || '.' || l_segment6; 25 BEGIN 26 SELECT id_flex_num 27 INTO l_structure_num 28 FROM apps.fnd_id_flex_structures 29 WHERE id_flex_code = 'GL#' 30 AND id_flex_structure_code = 'EPC_GL_ACCOUNTING_FLEXFIELD'; 31 EXCEPTION 32 WHEN OTHERS THEN 33 l_structure_num := NULL; 34 END; 35 ---------------Check if CCID exits with the above Concatenated Segments--------------- 36 BEGIN 37 SELECT code_combination_id 38 INTO l_ccid 39 FROM apps.gl_code_combinations_kfv 40 WHERE concatenated_segments = l_conc_segs; 41 EXCEPTION 42 WHEN OTHERS THEN 43 l_ccid := NULL; 44 END; 45 IF l_ccid IS NOT NULL THEN 46 ------------------------The CCID is Available---------------------- 47 DBMS_OUTPUT.PUT_LINE('COMBINATION_ID= ' || l_ccid); 48 ELSE 49 DBMS_OUTPUT.PUT_LINE('This is a New Combination. Validation Starts....'); 50 ------------Validate the New Combination-------------------------- 51 l_valid_combination := APPS.FND_FLEX_KEYVAL.VALIDATE_SEGS(operation => 'CHECK_COMBINATION', 52 appl_short_name => 'SQLGL', 53 key_flex_code => 'GL#', 54 structure_number => L_STRUCTURE_NUM, 55 concat_segments => L_CONC_SEGS); 56 p_error_msg1 := FND_FLEX_KEYVAL.ERROR_MESSAGE; 57 IF l_valid_combination then 58 DBMS_OUTPUT.PUT_LINE('Validation Successful! Creating the Combination...'); 59 -------------------Create the New CCID-------------------------- 60 L_CR_COMBINATION := APPS.FND_FLEX_KEYVAL.VALIDATE_SEGS(operation => 'CREATE_COMBINATION', 61 appl_short_name => 'SQLGL', 62 key_flex_code => 'GL#', 63 structure_number => L_STRUCTURE_NUM, 64 concat_segments => L_CONC_SEGS); 65 p_error_msg2 := FND_FLEX_KEYVAL.ERROR_MESSAGE; 66 IF l_cr_combination THEN 67 -------------------Fetch the New CCID-------------------------- 68 SELECT code_combination_id 69 INTO l_ccid 70 FROM apps.gl_code_combinations_kfv 71 WHERE concatenated_segments = l_conc_segs; 72 DBMS_OUTPUT.PUT_LINE('NEW COMBINATION_ID = ' || l_ccid); 73 ELSE 74 -------------Error in creating a combination----------------- 75 DBMS_OUTPUT.PUT_LINE('Error in creating the combination: ' || 76 p_error_msg2); 77 END IF; 78 ELSE 79 --------The segments in the account string are not defined in gl value set---------- 80 DBMS_OUTPUT.PUT_LINE('Error in validating the combination: ' || 81 p_error_msg1); 82 END IF; 83 END IF; 84 EXCEPTION 85 WHEN OTHERS THEN 86 DBMS_OUTPUT.PUT_LINE(SQLCODE || ' ' || SQLERRM); 87 END;
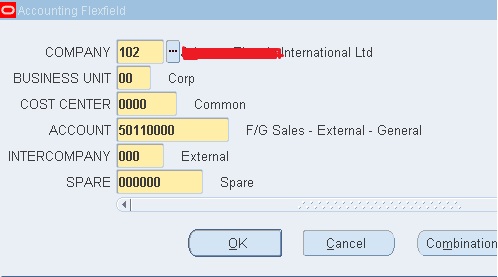
1 SQL> declare 2 pi_concat_segs varchar2(4000) := '102-00-0000-50110000-000-000000'; 3 po_desc varchar2(4000); 4 BEGIN 5 IF fnd_flex_keyval.validate_segs(operation => 'FIND_COMBINATION', 6 appl_short_name => 'SQLGL', 7 key_flex_code => 'GL#', 8 structure_number => 101, 9 concat_segments => pi_concat_segs ) THEN 10 po_desc := fnd_flex_keyval.concatenated_descriptions(); 11 ELSE 12 po_desc := null; 13 END IF; 14 dbms_output.put_line(po_desc); 15 END; 16 / 17 xxxxx -Corp-Common-F/G Sales - External - Ge-External-Spare 18 PL/SQL procedure successfully completed