Problem Description
A ring is compose of n circles as shown in diagram. Put natural number 1, 2, ..., n into each circle separately, and the sum of numbers in two adjacent circles should be a prime.
Note: the number of first circle should always be 1.
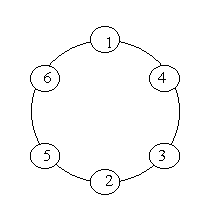
Note: the number of first circle should always be 1.
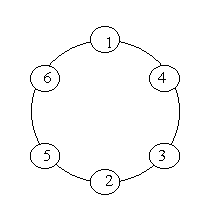
Input
n (0 < n < 20).
Output
The output format is shown as sample below. Each row represents a series of circle numbers in the ring beginning from 1 clockwisely and anticlockwisely. The order of numbers must satisfy the above requirements. Print solutions in lexicographical order.
You are to write a program that completes above process.
Print a blank line after each case.
You are to write a program that completes above process.
Print a blank line after each case.
Sample Input
6
8
Sample Output
Case 1:
1 4 3 2 5 6
1 6 5 2 3 4
Case 2:
1 2 3 8 5 6 7 4
1 2 5 8 3 4 7 6
1 4 7 6 5 8 3 2
1 6 7 4 3 8 5 2
1 #include <stdio.h> 2 #include <math.h> 3 #define MAX 20 4 int a[MAX],b[MAX],N; 5 int Is_Prime(int num) 6 { 7 for(int i=2;i<=(int)sqrt((double)num);i++) 8 if(num%i==0) 9 return 0; 10 return 1; 11 } 12 void Dfs(int n) 13 { 14 int i,j; 15 if(n==N && Is_Prime(a[n-1]+a[0])) 16 { 17 for(i=0;i<N;i++) 18 printf(i==N-1?"%d":"%d ",a[i]);//草蛋的格式 19 printf(" "); 20 } 21 else 22 { 23 for(i=1;i<N;i++) 24 { 25 if(b[i]!=0 && Is_Prime(a[n-1]+b[i])) 26 { 27 a[n++]=b[i]; 28 b[i]=0; 29 Dfs(n); 30 b[i]=i+1; 31 n--; 32 } 33 } 34 } 35 } 36 int main() 37 { 38 int i,j,k,num=1; 39 while(scanf("%d",&N)!=EOF) 40 { 41 a[0]=1; 42 for(i=0;i<N;i++) 43 b[i]=i+1; 44 printf("Case %d: ",num++); 45 Dfs(1); 46 printf(" "); 47 } 48 }