数组扁平化
1.使用arr.flat()
const arr = [1,[2,[3,[4,5]]], 6]
const res = arr.flat(Infinity)
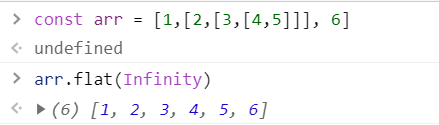
2.使用正则
const arr = [1,[2,[3,[4,5]]], 6]
const res = JSON.stringify(arr).split(',').map(n=>Number.parseInt(n))

3.正则版本2
const arr = [1,[2,[3,[4,5]]], 6]
const res = JSON.parse('[' + JSON.stringify(arr).replace(/[|]/g, '') + ']')
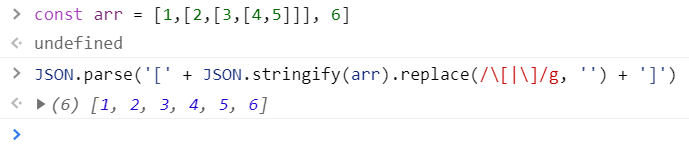
数组去重
1.使用Set
const arr = [1, 1, '1', 17, true, true, false, false, 'true', 'a', {}, {}]
const res = Array.from(new Set(arr))

2.使用indexOf
const arr = [1, 1, '1', 17, true, true, false, false, 'true', 'a', {}, {}]
const res = []
for(let i=0; i< arr.length; i++){
if(res.indexOf(arr[i]) === -1) res.push(arr[i])
}
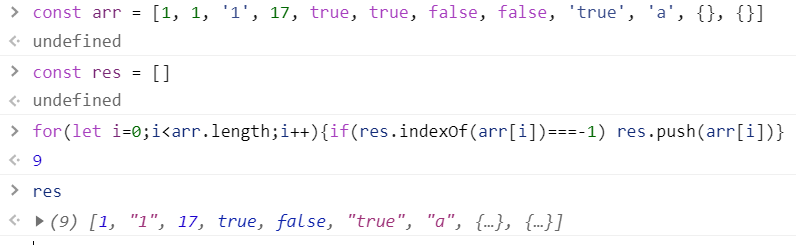
2.使用includes
const arr = [1, 1, '1', 17, true, true, false, false, 'true', 'a', {}, {}]
const res = []
for(let i=0; i< arr.length; i++){
if(!res.includes(arr[i])) res.push(arr[i])
}
3.使用filter
const arr = const arr = [1, 1, '1', 17, true, true, false, false, 'true', 'a', {}, {}]
const res = arr.filter((item, index)=> arr.indexOf(item)===index)
