问题描述
X星球的一处迷宫游乐场建在某个小山坡上。
它是由10x10相互连通的小房间组成的。
房间的地板上写着一个很大的字母。
我们假设玩家是面朝上坡的方向站立,则:
L表示走到左边的房间,
R表示走到右边的房间,
U表示走到上坡方向的房间,
D表示走到下坡方向的房间。
X星球的居民有点懒,不愿意费力思考。
他们更喜欢玩运气类的游戏。这个游戏也是如此!
开始的时候,直升机把100名玩家放入一个个小房间内。
玩家一定要按照地上的字母移动。
迷宫地图如下:
------------
UDDLUULRUL
UURLLLRRRU
RRUURLDLRD
RUDDDDUUUU
URUDLLRRUU
DURLRLDLRL
ULLURLLRDU
RDLULLRDDD
UUDDUDUDLL
ULRDLUURRR
------------
请你计算一下,最后,有多少玩家会走出迷宫?
而不是在里边兜圈子。
请提交该整数,表示走出迷宫的玩家数目,不要填写任何多余的内容。
如果你还没明白游戏规则,可以参看一个简化的4x4迷宫的解说图:
p1.png
图p1.png
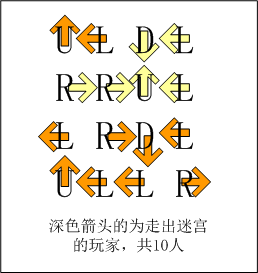
思路:
只需要统计每个点是否可以走出边界即可,具体判断是否出界,考虑使用图的深度搜索。
C/C++ 非递归实现
#include <iostream>
#include <string>
#include <stack>
#define SIZE 10
using namespace std;
string maze[SIZE];
bool check(int x,int y){
return (x > SIZE-1 || x < 0 || y > SIZE-1 || y < 0 );
}
bool dfs_maze(int x,int y){
int visited[SIZE][SIZE];
memset(visited, -1, sizeof(visited));
stack<pair<int,int>>s;
s.push(make_pair(x, y));
while (s.size()) {
pair<int,int> front = s.top();
s.pop();
int new_x = front.first;
int new_y = front.second;
if (visited[new_x][new_y] == 0) {
return false;
}
visited[new_x][new_y] = 0;
switch (maze[new_x][new_y]) {
case 'D':
new_x += 1;
break;
case 'U':
new_x += -1;
break;
case 'L':
new_y += -1;
break;
case 'R':
new_y += 1;
break;
}
if (check(new_x, new_y))
return true;
else
s.push(make_pair(new_x, new_y));
}
return false;
}
void test_maze(){
int ret = 0;
for(int i = 0;i < SIZE;i++)
cin >> maze[i];
for(int i = 0;i < SIZE;i++){
for (int j = 0; j < SIZE; j++) {
if(dfs_maze(i,j)){
ret++;
}
}
}
cout << ret << endl;
}
int main(int argc, const char * argv[]) {
test_maze();
return 0;
}