自定义json
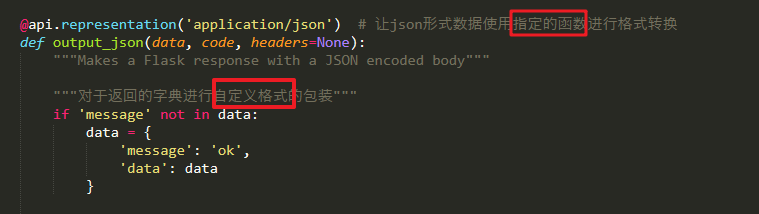
from flask import Flask
from flask_restful import Resource, Api
from flask import make_response, current_app
from flask_restful.utils import PY3
from json import dumps
app = Flask(__name__)
api = Api(app)
class User:
def __init__(self):
self.name = '张三'
self.age = 20
self.height = 1.8
self.scores = [80, 75]
self.info = {
'gender': True
}
def to_dict(self): # 模型的属性转为字典的键值对
return {
'username': self.name,
'age': self.age
}
class DemoResource(Resource):
def get(self):
user = User()
return user.to_dict()
def post(self):
return {'message': 'param error: username', 'data': None}
api.add_resource(DemoResource, '/index')
@api.representation('application/json') # 让json形式数据使用指定的函数进行格式转换
def output_json(data, code, headers=None):
"""Makes a Flask response with a JSON encoded body"""
"""对于返回的字典进行自定义格式的包装"""
if 'message' not in data:
data = {
'message': 'ok',
'data': data
}
settings = current_app.config.get('RESTFUL_JSON', {})
# If we're in debug mode, and the indent is not set, we set it to a
# reasonable value here. Note that this won't override any existing value
# that was set. We also set the "sort_keys" value.
if current_app.debug:
settings.setdefault('indent', 4)
settings.setdefault('sort_keys', not PY3)
# always end the json dumps with a new line
# see https://github.com/mitsuhiko/flask/pull/1262
dumped = dumps(data, **settings) + "
"
resp = make_response(dumped, code)
resp.headers.extend(headers or {})
return resp
if __name__ == '__main__':
app.run(debug=True, host='0.0.0.0')