本文章为转载,出处https://www.cnblogs.com/kuaixue/p/12717972.html
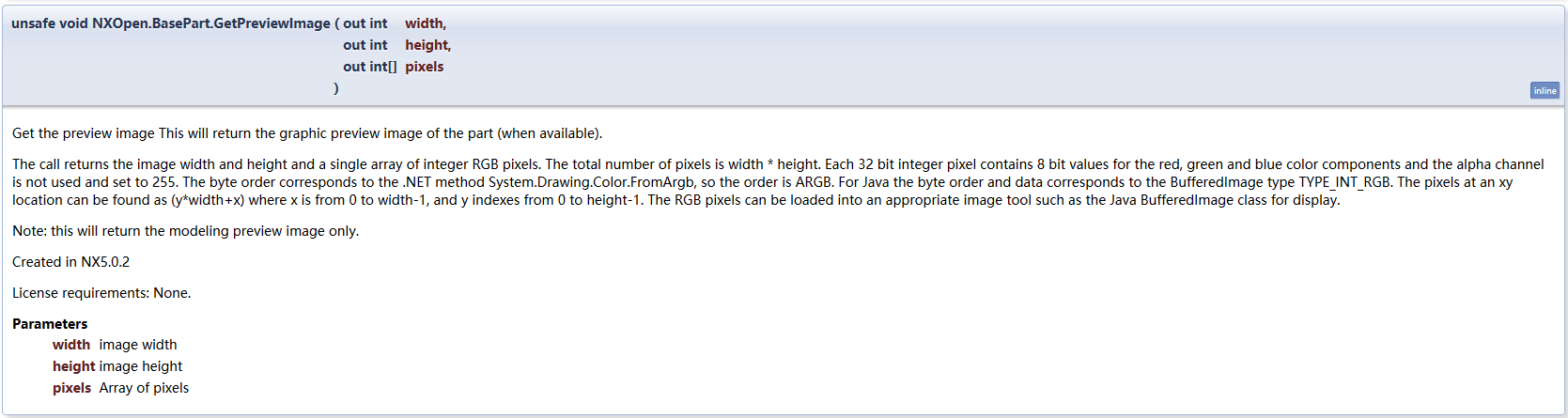
我刚才又找了一下,NXOpen C++也有
西门子GTAC官方上的一个.net例子(我没有webkey账号,代码是从上面大神博客转载的)
NX API Sample NX Open .NET C# program : create bitmap from selected part preview Note: GTAC provides programming examples for illustration only, and assumes that you are familiar with the programming language being demonstrated and the tools used to create and debug procedures. GTAC support professionals can help explain the functionality of a particular procedure, but we will not modify these examples to provide added functionality or construct procedures to meet your specific needs. // Shows existing preview image from work part or selected part // and also works external with 'run_journal' using System; using System.Drawing; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Text; using System.Windows.Forms; using NXOpen; public class Preview : Form { public static Session theSession; public static Part wp; public static ListingWindow lw; private System.Windows.Forms.Button preview_btn; private System.Windows.Forms.PictureBox pictureBox1; private System.Windows.Forms.Button close_btn; private System.Windows.Forms.Button load_btn; private System.Windows.Forms.Button save_btn; private TextBox textRelease; public Preview() { InitializeComponent(); } [STAThread] public static void Main(string[] args) { try { theSession = Session.GetSession(); wp = theSession.Parts.Work; lw = theSession.ListingWindow; Preview preview = new Preview(); preview.ShowDialog(); } catch (NXException ex) { MessageBox.Show(ex.Message); Console.WriteLine(ex.Message); } } private void getPreview(Part prt) { BasePart.HistoryEventInformation[] hInfo = prt.GetHistoryInformation(); string strInfo = ""; for (int i = 0; i < hInfo.Length; i++) { strInfo += hInfo[i] + " "; } textRelease.Text = strInfo; int width = 0; int height = 0; int[] pixels = null; prt.GetPreviewImage(out width, out height, out pixels); if (width > 0 && height > 0) { Bitmap image1 = createBitmap(width, height, pixels); pictureBox1.Image = image1; save_btn.Enabled = true; } else MessageBox.Show("Part has no valid preview", "Information"); } private Bitmap createBitmap(int w, int h, int[] px) { //lw.WriteLine("Bitmap Image Size: " + w.ToString() + "x" + h.ToString()); Bitmap bm = new Bitmap(w, h); for (int y = 0; y < h; y++) { for (int x = 0; x < w; x++) { int pixel = px[y * w + x]; Color col1 = Color.FromArgb(pixel); Color col2 = Color.FromArgb(255, col1); //lw.WriteLine(col2.ToString()); bm.SetPixel(x, y, col2); } } return bm; } public static DialogResult select_part_file(ref String filename) { OpenFileDialog ofd = new OpenFileDialog(); DialogResult result; ofd.Title = "Choose NX part file"; ofd.AddExtension = true; ofd.DefaultExt = "prt"; ofd.Filter = "NX Part files (*.prt)|*.prt|All files (*.*)|*.*"; ofd.FilterIndex = 1; ofd.ShowHelp = true; // start browsing at current folder, uncomment for any other default directory // ofd.InitialDirectory = GetEnvironmentVariable("UGII_BASE_DIR"); // ofd.InitialDirectory = GetEnvironmentVariable("UGII_BASE_DIR") + "Moldwizard"; // ofd.InitialDirectory = "c:mypath1mypath2"; result = ofd.ShowDialog(); filename = ofd.FileName; ofd.Dispose(); return result; } private void preview_btn_Click(object sender, EventArgs e) { if (wp == null) { MessageBox.Show("No Part", "Information"); return; } else { getPreview(wp); } } private void load_btn_Click(object sender, EventArgs e) { String filename = ""; if (select_part_file(ref filename) == DialogResult.OK) { PartLoadStatus loadStatus = null; int failCount = 0; Part mypart = theSession.Parts.Open(filename, out loadStatus); if (loadStatus.NumberUnloadedParts > 0) failCount = reportLoadStatus(loadStatus); else { getPreview(mypart); mypart.Close(BasePart.CloseWholeTree.True, BasePart.CloseModified.CloseModified, null); } } } public static int reportLoadStatus(PartLoadStatus loadStatus) { lw.Open(); int counter = 0; do { string file = loadStatus.GetPartName(counter); string failure = loadStatus.GetStatusDescription(counter); int statusCode = loadStatus.GetStatus(counter); MessageBox.Show("File: " + file + " Code: " + statusCode + " Status: " + failure, "Unloaded Part"); counter += 1; } while (!(counter == loadStatus.NumberUnloadedParts)); return counter; } private void save_btn_Click(object sender, EventArgs e) { SaveFileDialog save = new SaveFileDialog(); save.Filter = "Bitmap File(*.bmp)|*.bmp"; if (save.ShowDialog() == DialogResult.OK) { pictureBox1.Image.Save(save.FileName, System.Drawing.Imaging.ImageFormat.Bmp); } } private void close_btn_Click(object sender, EventArgs e) { Close(); } private void InitializeComponent() { this.preview_btn = new System.Windows.Forms.Button(); this.pictureBox1 = new System.Windows.Forms.PictureBox(); this.close_btn = new System.Windows.Forms.Button(); this.load_btn = new System.Windows.Forms.Button(); this.save_btn = new System.Windows.Forms.Button(); this.textRelease = new System.Windows.Forms.TextBox(); ((System.ComponentModel.ISupportInitialize)(this.pictureBox1)).BeginInit(); this.SuspendLayout(); // // preview_btn // this.preview_btn.Location = new System.Drawing.Point(14, 481); this.preview_btn.Name = "preview_btn"; this.preview_btn.Size = new System.Drawing.Size(75, 23); this.preview_btn.TabIndex = 0; this.preview_btn.Text = "Work Part"; this.preview_btn.UseVisualStyleBackColor = true; this.preview_btn.Click += new System.EventHandler(this.preview_btn_Click); // // pictureBox1 // this.pictureBox1.BorderStyle = System.Windows.Forms.BorderStyle.FixedSingle; this.pictureBox1.Location = new System.Drawing.Point(14, 12); this.pictureBox1.Name = "pictureBox1"; this.pictureBox1.Size = new System.Drawing.Size(534, 400); this.pictureBox1.SizeMode = System.Windows.Forms.PictureBoxSizeMode.Zoom; this.pictureBox1.TabIndex = 1; this.pictureBox1.TabStop = false; // // close_btn // this.close_btn.Location = new System.Drawing.Point(473, 481); this.close_btn.Name = "close_btn"; this.close_btn.Size = new System.Drawing.Size(75, 23); this.close_btn.TabIndex = 2; this.close_btn.Text = "Close"; this.close_btn.UseVisualStyleBackColor = true; this.close_btn.Click += new System.EventHandler(this.close_btn_Click); // // load_btn // this.load_btn.Location = new System.Drawing.Point(95, 481); this.load_btn.Name = "load_btn"; this.load_btn.Size = new System.Drawing.Size(75, 23); this.load_btn.TabIndex = 3; this.load_btn.Text = "Load Part"; this.load_btn.UseVisualStyleBackColor = true; this.load_btn.Click += new System.EventHandler(this.load_btn_Click); // // save_btn // this.save_btn.Enabled = false; this.save_btn.Location = new System.Drawing.Point(191, 481); this.save_btn.Name = "save_btn"; this.save_btn.Size = new System.Drawing.Size(75, 23); this.save_btn.TabIndex = 3; this.save_btn.Text = "Save BMP"; this.save_btn.UseVisualStyleBackColor = true; this.save_btn.Click += new System.EventHandler(this.save_btn_Click); // // textRelease // this.textRelease.BackColor = System.Drawing.SystemColors.Control; this.textRelease.BorderStyle = System.Windows.Forms.BorderStyle.FixedSingle; this.textRelease.Location = new System.Drawing.Point(14, 418); this.textRelease.Multiline = true; this.textRelease.Name = "textRelease"; this.textRelease.ScrollBars = System.Windows.Forms.ScrollBars.Vertical; this.textRelease.Size = new System.Drawing.Size(534, 57); this.textRelease.TabIndex = 4; // // Preview // this.AutoScaleDimensions = new System.Drawing.SizeF(6F, 13F); this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Font; this.ClientSize = new System.Drawing.Size(563, 516); this.Controls.Add(this.load_btn); this.Controls.Add(this.save_btn); this.Controls.Add(this.close_btn); this.Controls.Add(this.pictureBox1); this.Controls.Add(this.preview_btn); this.Controls.Add(this.textRelease); this.Name = "Preview"; this.Text = "NX Part Preview"; ((System.ComponentModel.ISupportInitialize)(this.pictureBox1)).EndInit(); this.ResumeLayout(false); this.PerformLayout(); } }
使用步骤:
1.使用开发向导先新建一个NXOpen C#项目(是编译成功的项目)
2.将上面代码替换到项目中
3.引入这两个
4.编译成功
演示:
除了这个方法外,还有其他的读取方法,上面的大神博客上有介绍。
补充:
prt文件是一个复合文档
可以用7-Zip去解压出来,会在里面找到预览图,可以用画图打开。
既然是这样,就可以写C++或者C#代码去读取这个图片了。
这部分资料,转载自大神小猛微信公众号
获取NX图档缩略图https://mp.weixin.qq.com/s?__biz=MzU5MTcyMzUxMg==&mid=2247483739&idx=1&sn=5f6a9a08c7f28234288000b522b8ccc3&chksm=fe2befccc95c66dab405c1a28a72ebb7f287aac019e250be4803ce25bd1c120e2a952b82ff58&mpshare=1&scene=23&srcid=08317ykWRXhlXzmhy8VXMyWq&sharer_sharetime=1598868484417&sharer_shareid=f9acc1784d34dc6b930269ce523df917#rd
C#如何读复合文档 https://mp.weixin.qq.com/s?__biz=MzU5MTcyMzUxMg==&mid=2247483745&idx=1&sn=70c1e016357fe504511054e0c55b30b5&chksm=fe2beff6c95c66e0d1a20c4711409e169b4911a3f6bb466d252ff9d9ab4436ba183c9df78ad5&mpshare=1&scene=23&srcid=0831eUi1pgplsxJzB6krpk9I&sharer_sharetime=1598868515557&sharer_shareid=f9acc1784d34dc6b930269ce523df917#rd
C#创建复合文档
https://mp.weixin.qq.com/s?__biz=MzU5MTcyMzUxMg==&mid=2247483759&idx=1&sn=b448dfd41cb9db872cbbe002739677af&chksm=fe2beff8c95c66eeae30f54d927ccf50219b8a95bb2a14206992a687481a78700c820fcb3198&mpshare=1&scene=23&srcid=0831OusJeWGQTAJZMOc3SbMq&sharer_sharetime=1598868542472&sharer_shareid=f9acc1784d34dc6b930269ce523df917#rd
.NET反射的应用 https://mp.weixin.qq.com/s?__biz=MzU5MTcyMzUxMg==&mid=2247483751&idx=1&sn=aa62c13adbc9a4b69d696bce88226535&chksm=fe2beff0c95c66e654c3db3f87ca5d694307e1830235669c0864f73727d7e16795b85c07e909&mpshare=1&scene=23&srcid=0831exkuErh5ChIChkCCG6zC&sharer_sharetime=1598868557028&sharer_shareid=f9acc1784d34dc6b930269ce523df917#rd
Caesar卢尚宇
2020年8月31日