随机漫游
# random_flow.py 随机漫游
import random
class RandomFlow():
"""一个生成随机漫游数据的类"""
def __init__(self, num_points=5000):
self.num_points = num_points
self.x_values = [0]
self.y_values = [0]
def fill_walk(self):
while len(self.x_values) < self.num_points:
# To left or to right
x_direction = random.choice([1, -1])
x_distance = random.choice([0, 1, 2, 3, 4])
x_step = x_direction * x_distance
y_direction = random.choice([1, -1])
y_distance = random.choice([0, 1, 2, 3, 4])
y_step = y_direction * y_distance
# must move
if x_step == 0 and y_step == 0:
continue
next_x = self.x_values[-1] + x_step
next_y = self.y_values[-1] + y_step
self.x_values.append(next_x)
self.y_values.append(next_y)
# rf_visual.py 实现随机漫游
import matplotlib.pyplot as plt
import random
from random_flow import RandomFlow
while True:
rf = RandomFlow()
rf.fill_walk()
plt.plot(rf.x_values, rf.y_values, color='b', linewidth=1)
plt.title('Random Flow', fontsize=24)
plt.axes().get_xaxis().set_visible(False)
plt.axes().get_yaxis().set_visible(False)
filename = 'rf_' + str(random.randint(000000, 999999)) + '.png'
plt.savefig('images/' + filename, bbox_inches='tight')
flag = input('Keep flowing? (Y/N): ')
if flag.lower() not in ['y', 'yes']:
break
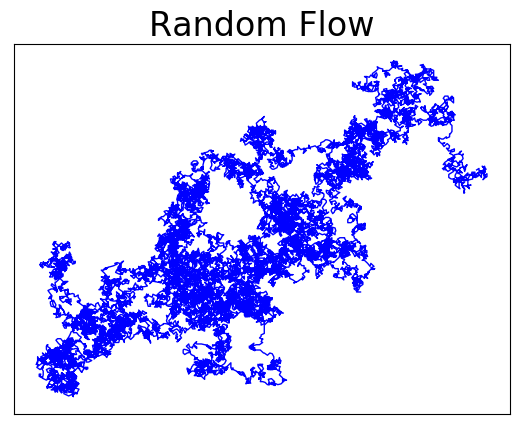
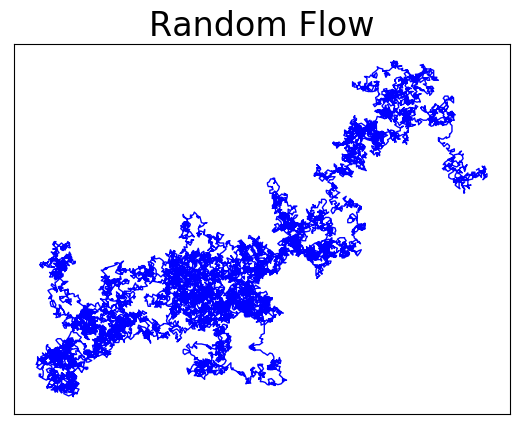
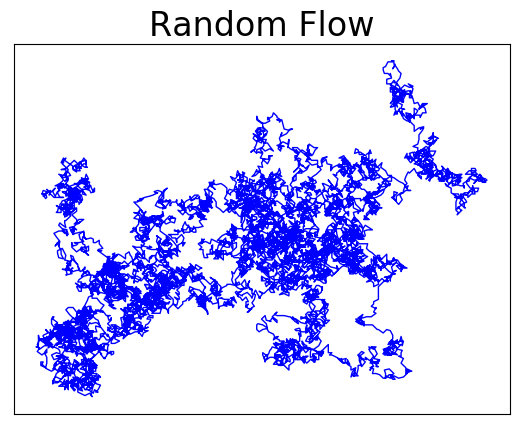
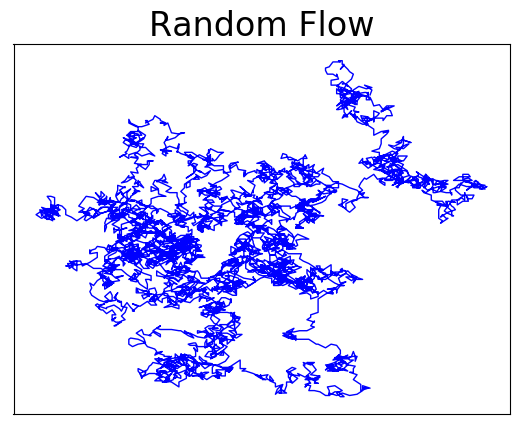
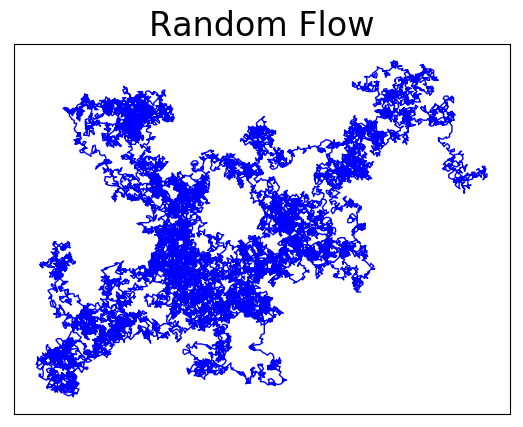