先上效果图
1、说明
Ext.tree.Panel 控件是树形控件,大家知道树形结构在软件开发过程中的应用是很广泛的,树形控件的数据有本地数据、服务器端返回的数据两种。
对于本地数据的加载,在extjs的 api文档中都有介绍,但是Extjs.tree.Panel怎么动态加载数据库中的数据,API文档介绍的较少,
以至于初学者,不知道怎么实现。
本篇文章将介绍Extjs.tree.Panel动态加载数据的思路。
本篇文章的主要内容有:
(1)、搭建环境
(2)、创建数据表
(3)、创建实体类
(4)、创建ViewModel
(5)、编写后台代码
(6)、编写前台代码
注意:虽然本文写的非常详细,但是也要求读者具有mvc、fluentdata方面的知识准备。
一、搭建环境
开发环境:visualstudio 2012
ORM : fluentdata
开发语言:c#
项目类型:asp.net mvc4
搭建环境步骤:
(1)、打开visualstudio 2012 ,新建-项目-模板-visual c#-web-asp.net mvc4 (基本)
(2)、引用fluentd.dll
在方案资源管理器中,找到刚刚创建的项目,右键单击“引用”文件夹,在弹出菜单选择“添加引用”,浏览,找到fluentd.dll 单击“确定”按钮即可
(3)、在项目下添加一个文件夹,命名为“DAL”,在该文件夹中添加一个类DBHelper.cs
(4)、在web.config文件中添加数据库连接字符串
<connectionStrings>
<add name="testDBContext" connectionString="server=192.168.1.105;uid=sa;pwd=***;database=NewDB;"/>
</connectionStrings>
DBHelper.cs类代码如下:

using System; using System.Collections.Generic; using System.Linq; using System.Web; using FluentData; namespace MvcExtJsTree.DAL { public class DBHelper { public static IDbContext Context() { return new DbContext().ConnectionStringName("testDBContext", new SqlServerProvider()); } } }
注意:用别的orm也可以,只要能访问数据库即可。
二、创建数据表
首先在sql数据库中创建一个数据库,命名为NewDB
接下来,在该数据库下创建数据表,命名为testTree
1、创建数据表的脚本如下:

USE NewDB GO CREATE TABLE testTree ( id int primary key identity(1,1) NOT NULL, myTitle nvarchar(50) NULL, iconCls nvarchar(50) NULL, fatherId int NOT NULL )
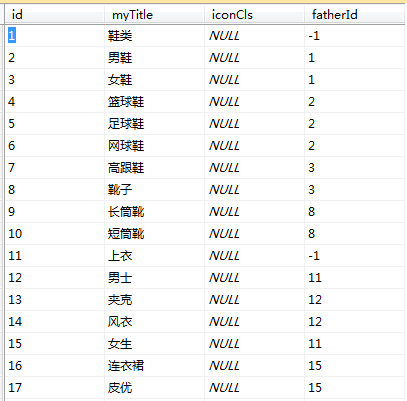
该类的作用是方法数据,查询数据用的
代码如下:

using System; using System.Collections.Generic; using System.Linq; using System.Web; namespace MvcExtJsTree.Models { public class testTree { public int id { get; set; } public string myTitle { get; set; } public string iconCls { get; set; } public int fatherId { get; set; } } }
四、创建ViewModel
在项目下添加一个文件夹,命名为ViewModel
在该文件夹下添加一个类,命名为Tree.cs
该类的作用就是把后台从数据库查询出的数据转换为前台treePanel支持的数据格式
Tree.cs类的代码如下:

using System; using System.Collections.Generic; using System.Linq; using System.Web; namespace MvcExtJsTree.ViewModel { public class Tree { public String id; public String text; public String iconCls; public Boolean leaf; public String fatherId; public List<Tree> children; } }
五、mvc项目Home控制器的代码如下:

using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.Mvc; using FluentData; using MvcExtJsTree.Models; using MvcExtJsTree.ViewModel; namespace MvcExtJsTree.Controllers { public class HomeController : Controller { // // GET: /Home/ public ActionResult Index() { return View(); } public ActionResult HandlerTreeFromDB() { var categoryList = DAL.DBHelper.Context().Select<testTree>("*").From("testTree").QueryMany(); var result = this.ConvertTreeNodes(categoryList); return Json(result, JsonRequestBehavior.AllowGet); } #region 和树节点相关的 private List<Tree> ConvertTreeNodes(List<testTree> listCategory) { List<Tree> listTreeNodes = new List<Tree>(); LoadTreeNode(listCategory, listTreeNodes, -1); return listTreeNodes; } private void LoadTreeNode(List<testTree> listCategory, List<Tree> listTreeNodes, int pid) { foreach (testTree category in listCategory) { if (category.fatherId == pid) { Tree node = this.TransformTreeNode(category); listTreeNodes.Add(node); LoadTreeNode(listCategory, node.children,Convert.ToInt32(node.id)); } } } private Tree TransformTreeNode(testTree category) { Tree treeNode = new Tree() { id = category.id.ToString(), text = category.myTitle, leaf=false, fatherId=category.fatherId.ToString(), iconCls=category.iconCls, children = new List<Tree>() }; var categoryId = category.id; var childrens = DAL.DBHelper.Context().Select<testTree>("*").From("testTree").Where("fatherId=@0").Parameters(categoryId).QueryMany(); //判断节点是否有子节点 if (childrens.Count == 0) { treeNode.leaf=true; treeNode.children = null; } return treeNode; } #endregion } }
六、Home控制器的Index方法对应的Index.cshtml页面的代码如下:

@{ Layout = null; } <!DOCTYPE html> <html> <head> <meta name="viewport" content="width=device-width" /> <title>Index</title> <link href="~/ExtJS4.2/resources/css/ext-all-neptune-rtl.css" rel="stylesheet" /> <link href="~/ExtJS4.2/resources/css/ext-all.css" rel="stylesheet" /> <script type="text/javascript" src="~/ExtJS4.2/ext-all.js"></script> <script src="~/ExtJS4.2/locale/ext-lang-zh_CN.js"></script> <script type="text/javascript"> Ext.onReady(function () { var model = Ext.define("TreeModel", { // 定义树节点数据模型 extend: "Ext.data.Model", fields: [ { name: "id", type: "string" }, { name: "text", type: "string" }, { name: "iconCls", type: "string" }, { name: "leaf", type: "boolean" }, { name: 'url', type: "string" }, { name: 'description', type: "string" }] }); var store = Ext.create('Ext.data.TreeStore', { model: model,//定义当前store对象的Model数据模型 // autoLoad : true, proxy: { type: 'ajax', url: 'Home/HandlerTreeFromDB',//请求 reader: { type: 'json', // root : 'data'//数据 } }, root: {//定义根节点,此配置是必须的 // text : '管理菜单', expanded: true } }); Ext.create('Ext.tree.Panel', { title: 'Simple Tree', 200, height: 550, store: store, rootVisible: false,//隐藏根节点 renderTo: Ext.getBody() }); }); </script> </head> <body> </body> </html>
注意:
(1)、需要注意的是,在项目下添加文件夹“ExtJS4.2”,并把extjs4.2的源文件拷贝到该文件夹下,即给项目引用extjs4.2框架。
(2)、后台代码处理思路:通过递归,把从数据库中查询的数据转换为Ext.tree.Panel所支持的数据
七、土豪打赏