HTTP-GET和HTTP-POST定义:
都是使用HTTP的标准协议动词,用于编码和传送变量名/变量值对参数,并且使用相关的请求语义。
每个HTTP-GET和HTTP-POST都由一系列HTTP请求头组成,这些请求头定义了客户端从服务器请求了什么,
而响应则是由一系列HTTP请求数据和响应数据组成,如果请求成功则返回响应的数据。
Ø HTTP-GET以使用MIME类型application/x-www-form-urlencoded的urlencoded文本的格式传递参数。
Urlencoding是一种字符编码,保证被传送的参数由遵循规范的文本组成,例如一个空格的编码是"%20"。附加参数还能被认为是一个查询字符串。
Ø与HTTP-GET类似,HTTP-POST参数也是被URL编码的。然而,变量名/变量值不作为URL的一部分被传送,而是放在实际的HTTP请求消息内部被传送。
GET和POST之间的主要区别:
1、GET是从服务器上获取数据,POST是向服务器传送数据。
2、在客户端, GET方式在通过URL提交数据,数据在URL中可以看到;POST方式,数据放置在HTML HEADER内提交
3、对于GET方式,服务器端用Request.QueryString获取变量的值,对于POST方式,服务器端用Request.Form获取提交的数据。
4、GET方式提交的数据最多只能有1024字节,而POST则没有此限制
5、安全性问题。正如在(2)中提到,使用 GET 的时候,参数会显示在地址栏上,而 POST 不会。所以,如果这些数据是中文数据而且是非敏感数据,那么使用 GET ;如果用户输入的数据不是中文字符而且包含敏感数据,那么还是使用 POST为好
Java中进行HTTP编程有如下的两种方式:标准的Java接口和标准Apache接口
标准的Java接口 两个案例(GET和POST)
案例1:以Java接口GET方式从服务器获得一张图片下载到本地磁盘
首次得导入相关第三方的包
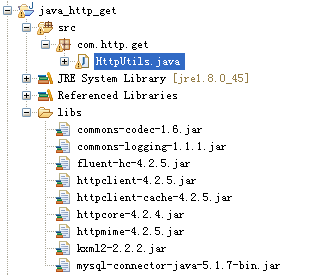

1 package com.http.get; 2 3 import java.io.FileOutputStream; 4 import java.io.IOException; 5 import java.io.InputStream; 6 import java.net.HttpURLConnection; 7 import java.net.MalformedURLException; 8 import java.net.URL; 9 10 public class HttpUtils { 11 12 private static String urlPath="http://i6.topit.me/6/5d/45/1131907198420455d6o.jpg"; 13 private static HttpURLConnection httpURLConnection=null; 14 public HttpUtils() { 15 // TODO Auto-generated constructor stub 16 } 17 public static InputStream getInputStream() throws IOException{ 18 InputStream inputStream=null; 19 URL url=new URL(urlPath); 20 if(url!=null){ 21 httpURLConnection=(HttpURLConnection)url.openConnection(); 22 httpURLConnection.setConnectTimeout(3000); 23 httpURLConnection.setDoInput(true); 24 httpURLConnection.setRequestMethod("GET"); 25 int code=httpURLConnection.getResponseCode(); 26 if(code==200){ 27 inputStream=httpURLConnection.getInputStream(); 28 } 29 } 30 return inputStream; 31 } 32 public static void getImgToDisk() throws IOException{ 33 InputStream inputStream=getInputStream(); 34 FileOutputStream fileOutputStream=new FileOutputStream("D:\TEST.png"); 35 byte[] data=new byte[1024]; 36 int len=0; 37 while((len=inputStream.read(data))!=-1){ 38 fileOutputStream.write(data, 0, len); 39 } 40 if(inputStream!=null) 41 inputStream.close(); 42 if(fileOutputStream!=null) 43 fileOutputStream.close(); 44 } 45 public static void main(String[] args) throws IOException { 46 getImgToDisk(); 47 } 48 }
案例2:以Java接口POST方式向服务器提交用户名和密码,在服务器端进行简单判断,如果正确返回字符串success,否则返回fail
服务器端简单校验:

1 */ 2 public void doPost(HttpServletRequest request, HttpServletResponse response) 3 throws ServletException, IOException { 4 response.setContentType("text/html;charset=utf-8"); 5 request.setCharacterEncoding("utf-8"); 6 response.setCharacterEncoding("utf-8"); 7 8 PrintWriter out = response.getWriter(); 9 //String jsonString =JsonTools.createJsonString("person", service.getPerson()); 10 String name = request.getParameter("username"); 11 String pwd = request.getParameter("password"); 12 if (name.equals("admin")&&pwd.equals("123")) { 13 out.print("success"); 14 } 15 else { 16 out.print("fail"); 17 } 18 out.flush(); 19 out.close(); 20 }
客户端的提交:

1 package com.http.post; 2 import java.io.ByteArrayOutputStream; 3 import java.io.IOException; 4 import java.io.InputStream; 5 import java.io.OutputStream; 6 import java.io.UnsupportedEncodingException; 7 import java.net.HttpURLConnection; 8 import java.net.MalformedURLException; 9 import java.net.URL; 10 import java.net.URLEncoder; 11 import java.util.HashMap; 12 import java.util.Map; 13 14 import sun.net.www.http.HttpClient; 15 16 public class HttpUtils { 17 18 private static String PATH="http://122.206.79.193:8080/myhttp/servlet/LoginAction"; 19 private static URL URL; 20 static{ 21 try { 22 URL=new URL(PATH); 23 } catch (MalformedURLException e) { 24 // TODO Auto-generated catch block 25 e.printStackTrace(); 26 } 27 } 28 public HttpUtils() { 29 // TODO Auto-generated constructor stub 30 } 31 32 /** 33 * 34 * @param params 填写的参数 35 * @param encode 字节编码 为了避免乱码 36 * @return 从服务端返回的信息 37 * @throws Exception 38 */ 39 public static String sendPostMessage(Map<String,String>params,String encode) throws Exception{ 40 StringBuffer sb=new StringBuffer(); 41 if(params!=null&&!params.isEmpty()){ 42 for(Map.Entry<String,String> entry:params.entrySet()){ 43 sb.append(entry.getKey()).append("=").append(URLEncoder.encode(entry.getValue(),encode)).append("&"); 44 } 45 sb.deleteCharAt(sb.length()-1); 46 HttpURLConnection httpURLConnection=(HttpURLConnection)URL.openConnection(); 47 httpURLConnection.setConnectTimeout(3000); 48 httpURLConnection.setDoInput(true);//从服务器获取数据 49 httpURLConnection.setDoOutput(true);//向服务器提交数据 50 //获得上传信息字节大小及长度 51 byte[] data=sb.toString().getBytes(); 52 //浏览器内置封装了http协议信息,但是手机或者用Java访问时并没有 所以最重要的是需要设置请求体的属性封装http协议 53 //设置请求体的请求类型是文本类型 54 httpURLConnection.setRequestProperty("Content-Type", "application/x-www-form-urlencoded");//文本类型 55 //设置请求体的长度 56 httpURLConnection.setRequestProperty("Content-Length",String.valueOf(data.length)); 57 //想要向服务器提交数据必须设置输出流,向服务器输出数据 58 System.out.println(httpURLConnection.toString()); 59 OutputStream out=httpURLConnection.getOutputStream(); 60 out.write(data, 0, data.length); 61 out.close(); 62 int code=httpURLConnection.getResponseCode(); 63 if(code==200){ 64 return changeInputStream(httpURLConnection.getInputStream(),encode); 65 } 66 67 } 68 return ""; 69 } 70 private static String changeInputStream(InputStream inputStream,String encode) { 71 // TODO Auto-generated method stub 72 String resString = ""; 73 ByteArrayOutputStream outputStream = new ByteArrayOutputStream();//内存流 74 int len = 0; 75 byte[] data = new byte[1024]; 76 try { 77 while ((len = inputStream.read(data)) != -1) { 78 outputStream.write(data, 0, len); 79 } 80 resString = new String(outputStream.toByteArray()); 81 } catch (IOException e) { 82 // TODO Auto-generated catch block 83 e.printStackTrace(); 84 } 85 return resString; 86 } 87 public static void main(String[] args) throws Exception { 88 Map<String,String>params=new HashMap<String, String>(); 89 params.put("username", "admin"); 90 params.put("password", "123"); 91 String res=sendPostMessage(params,"utf-8"); 92 System.out.println("-res->>"+res); 93 } 94 }
标准Apache接口

1 package com.http.post; 2 3 import java.io.ByteArrayOutputStream; 4 import java.io.IOException; 5 import java.io.InputStream; 6 import java.io.UnsupportedEncodingException; 7 import java.util.ArrayList; 8 import java.util.HashMap; 9 import java.util.List; 10 import java.util.Map; 11 12 import org.apache.http.HttpResponse; 13 import org.apache.http.NameValuePair; 14 import org.apache.http.client.ClientProtocolException; 15 import org.apache.http.client.entity.UrlEncodedFormEntity; 16 import org.apache.http.client.methods.HttpPost; 17 import org.apache.http.impl.client.DefaultHttpClient; 18 import org.apache.http.message.BasicNameValuePair; 19 20 public class HttpUtils { 21 22 public HttpUtils() { 23 // TODO Auto-generated constructor stub 24 } 25 public static String sendHttpClientPost(String path,Map<String,String>map,String encode) throws Exception, IOException{ 26 27 //将参数map进行封装到list中 28 List<NameValuePair> list=new ArrayList<NameValuePair>(); 29 if(map!=null&&!map.isEmpty()){ 30 for(Map.Entry<String, String> entry:map.entrySet()){ 31 list.add(new BasicNameValuePair(entry.getKey(), entry.getValue())); 32 } 33 } 34 try { 35 //将请求的参数封装的list到表单,即请求体中 36 UrlEncodedFormEntity entity=new UrlEncodedFormEntity(list,encode); 37 //使用post方式提交数据 38 HttpPost httpPost=new HttpPost(path); 39 httpPost.setEntity(entity); 40 DefaultHttpClient client=new DefaultHttpClient(); 41 HttpResponse response= client.execute(httpPost); 42 //以上完成提交 43 //以下完成读取服务端返回的信息 44 if(response.getStatusLine().getStatusCode()==200){ 45 return changeInputStream(response.getEntity().getContent(),encode); 46 } 47 } catch (UnsupportedEncodingException e) { 48 // TODO Auto-generated catch block 49 e.printStackTrace(); 50 } 51 return ""; 52 } 53 public static String changeInputStream(InputStream inputStream,String encode) { 54 // TODO Auto-generated method stub 55 String resString = ""; 56 ByteArrayOutputStream outputStream = new ByteArrayOutputStream();//内存流 57 int len = 0; 58 byte[] data = new byte[1024]; 59 try { 60 while ((len = inputStream.read(data)) != -1) { 61 outputStream.write(data, 0, len); 62 } 63 resString = new String(outputStream.toByteArray()); 64 } catch (IOException e) { 65 // TODO Auto-generated catch block 66 e.printStackTrace(); 67 } 68 return resString; 69 } 70 public static void main(String[] args) throws Exception, Exception { 71 String path="http://localhost:8080/myhttp/servlet/LoginAction"; 72 Map<String,String>map=new HashMap<String, String>(); 73 map.put("username", "admin"); 74 map.put("password", "123"); 75 String msg=HttpUtils.sendHttpClientPost(path, map, "utf-8"); 76 System.out.println("-->>--"+msg); 77 } 78 79 }