http://www.gamedev.net/topic/578866-d3d10-how-to-increase-maxcount-of-sv_clipdistance/
The D3D#_CLIP_OR_CULL_DISTANCE_* values are #defines in the d3d11.h header file. For distance count the value is 8 and for element count the value is 2. This means you can have any number of clip or cull values provide that their sum is 8 or less and that they must be fit into 2 vec4 registers.
In D3D10+ you have to code the math yourself in the shader to put the vertex through the plane equation and then store the resulting distance value in one of the registers. Values greater than or equal to zero should not be clipped/culled. The values you provide will be interpolated so that each pixel is clipped appropriately.
Here is an example, using just cull distance.
cbuffer ClipPlanes
{
float4 planes[6];
};
struct VS_OUT
{
float4 pos : SV_Position;
float4 clips[2] : SV_CullDistance;
};
VS_OUT vsmain( float4 pos : SV_Position )
{
VS_OUT vsout = (VS_OUT)0;
vsout.pos = pos;
for( uint i = 0; i < planes.Length; ++i )
{
((float[8])vsout.clips)[i] = dot( planes[i].xyz, pos.xyz ) - planes[i].w;
}
return vsout;
}
下面D3D文档中对ClipDistance和CullDistance的描述。gpu将会在体元装配阶段进行这些clip和cull操作。
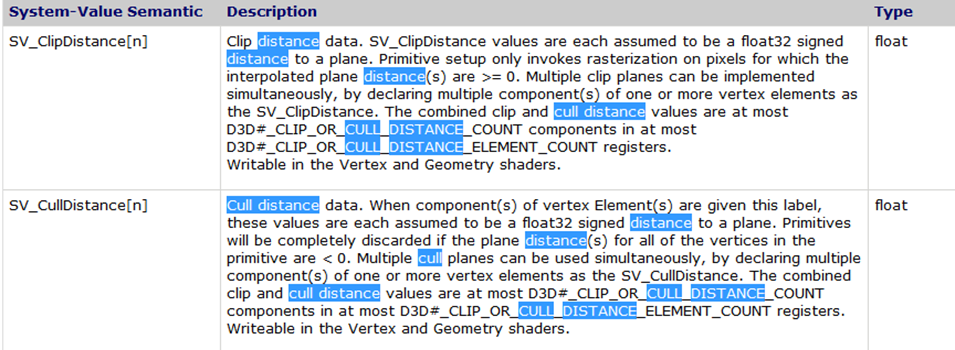