版权声明:本文为博主原创文章,未经博主允许不得转载。
WebService、soap、gsoap
WebService:就是一个应用程序,它向外界暴露出一个可以通过web进行调用的API,是分布式的服务组件。本质上就是要以标准的形式实现企业内外各个不同服务系统之间的互调和集成。
soap:简单对象访问协议,是一种轻量的、简单的、基于 XML 的协议,它被设计成在WEB 上交换结构化的和固化的信息。从这里的概念可以看得出来,soap是一个基于xml格式的web交互协议,而webservice是一种使用web方式实现的功能。就好像是网络视频服务器和http的关系,就是这里的webservice服务器和soap的关系。其实从历史上来说,先有的soap这种协议,然后微软用基于这种协议制作了webservice这种服务。
gsoap:是一种能够把C/C++语言的接口转换成基于soap协议的webservice服务的工具。
soap:简单对象访问协议,是一种轻量的、简单的、基于 XML 的协议,它被设计成在WEB 上交换结构化的和固化的信息。从这里的概念可以看得出来,soap是一个基于xml格式的web交互协议,而webservice是一种使用web方式实现的功能。就好像是网络视频服务器和http的关系,就是这里的webservice服务器和soap的关系。其实从历史上来说,先有的soap这种协议,然后微软用基于这种协议制作了webservice这种服务。
gsoap:是一种能够把C/C++语言的接口转换成基于soap协议的webservice服务的工具。
使用gsoap创建webservice服务
下载gsop
首先到http://sourceforge.net/projects/gsoap2/files/gSOAP/下载gsoap的最新版本。解压后目录结构如下:
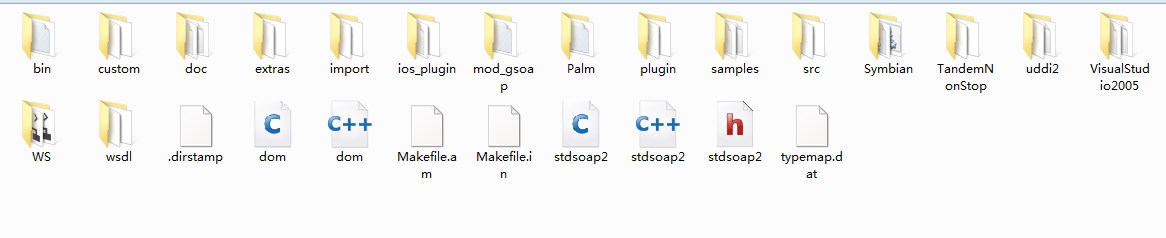
我们看到binwin32目录下有两个exe可执行文件:soapcpp2.exe,wsdl2h.exe。另外根目录还有两个比较重要的源文件:stdsoap2.h和stdsoap2.cpp。
准备待导出的服务接口定义文件(比如gservice.h)
//gsoap ns service name: gservice
//gsoap ns service style: rpc
int ns__add(int num1, int num2, int* result );
int ns__sub(int num1, int num2, int* result );
int ns__mult( int num1, int num2, int *result);
int ns__divid( int num1, int num2, int *result);
新建webservice服务器端(gServer)
选择空控制台程序,并将soapcpp2.exe、stdsoap2.h、stdsoap2.cpp、gservice.h文件拷贝到gserver目下。启用cmd命令行程序,进入到gServer目录下执行如下命令:
soapcpp2.exe -S gservice.h
生成服务器端接口文件。并将stdsoap2.h、soapStub.h、soapH.h、gservice.nsmap和stdsoap2.cpp、soapC.cpp、soapServer.cpp文件导入到gServer中,项目目录结构如下:
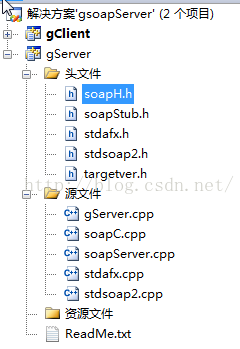
实现main函数:
#include
"stdio.h"
#include "soapH.h"
#include "gservice.nsmap"
#include "soapH.h"
#include "gservice.nsmap"
int
main(int argc,char **argv)
{
int nPort = 8080;
struct soap fun_soap;
soap_init(&fun_soap);
int nMaster = (int)soap_bind(&fun_soap, NULL, nPort, 100);
if (nMaster < 0)
{
soap_print_fault(&fun_soap, stderr);
exit(-1);
}
{
int nPort = 8080;
struct soap fun_soap;
soap_init(&fun_soap);
int nMaster = (int)soap_bind(&fun_soap, NULL, nPort, 100);
if (nMaster < 0)
{
soap_print_fault(&fun_soap, stderr);
exit(-1);
}
fprintf(stderr, "Socket connection successful : master socket = %d ", nMaster);
while (true)
{
int nSlave = (int)soap_accept(&fun_soap);
if (nSlave < 0)
{
soap_print_fault(&fun_soap, stderr);
exit(-1);
}
fprintf(stderr, "Socket connection successful : slave socket = %d ", nSlave);
soap_serve(&fun_soap);
soap_end(&fun_soap);
}
return 0;
}
{
int nSlave = (int)soap_accept(&fun_soap);
if (nSlave < 0)
{
soap_print_fault(&fun_soap, stderr);
exit(-1);
}
fprintf(stderr, "Socket connection successful : slave socket = %d ", nSlave);
soap_serve(&fun_soap);
soap_end(&fun_soap);
}
return 0;
}
/*加法的具体实现*/
int ns__add(struct soap *soap, int num1, int num2, int* result )
{
if (NULL == result)
{
printf("Error:The third argument should not be NULL! ");
return SOAP_ERR;
}
else
{
(*result) = num1 + num2;
return SOAP_OK;
}
return SOAP_OK;
}
/*减法的具体实现*/
int ns__sub(struct soap *soap,int num1, int num2, int* result )
{
if (NULL == result)
{
printf("Error:The third argument should not be NULL! ");
return SOAP_ERR;
}
else
{
(*result) = num1 - num2;
return SOAP_OK;
}
return SOAP_OK;
}
/*乘法的具体实现*/
int ns__mult(struct soap *soap, int num1, int num2, int *result)
{
if (NULL == result)
{
printf("Error:The third argument should not be NULL! ");
return SOAP_ERR;
}
else
{
(*result) = num1 * num2;
return SOAP_OK;
}
return SOAP_OK;
}
/*除法的具体实现*/
int ns__divid(struct soap *soap, int num1, int num2, int *result)
{
if (NULL == result || 0 == num2)
{
printf("Error:The second argument is 0 or The third argument is NULL! ");
return SOAP_ERR;
}
else
{
(*result) = num1 / num2;
return SOAP_OK;
}
return SOAP_OK;
}
编译,运行服务器端程序,从浏览器访问localhost:8080
如能看到如下信息,说明服务器运行正常:
XML
Source Code
新建webservice客户端(gClient)
选择空控制台程序,并将soapcpp2.exe、stdsoap2.h、stdsoap2.cpp、gservice.h文件拷贝到gClient目下。启用cmd命令行程序,进入到gClient目录下执行如下命令:
soapcpp2.exe -C gservice.h
生成客户端代理接口文件。并将stdsoap2.h、soapStub.h、soapH.h、gservice.nsmap和stdsoap2.cpp、soapC.cpp、soapClient.cpp文件导入到gClient中,项目目录结构如下:
实现main函数:
#include "stdio.h"
#include "gservice.nsmap"
int main( int argc, char *argv[])
{
printf("The Client is runing... ");
int num1 = 110;
int num2 = 11;
int result = 0;
struct soap *CalculateSoap = soap_new();
//soap_init(CalculateSoap);
char * server_addr = "http://localhost:8080";
int iRet = soap_call_ns__add(CalculateSoap,server_addr,"",num1,num2,&result);
if ( iRet == SOAP_ERR)
{
printf("Error while calling the soap_call_ns__add");
}
else
{
printf("Calling the soap_call_ns__add success。 ");
printf("%d + %d = %d ",num1,num2,result);
}
iRet = soap_call_ns__sub(CalculateSoap,server_addr,"",num1,num2,&result);
if ( iRet == SOAP_ERR)
{
printf("Error while calling the soap_call_ns__sub");
}
else
{
printf("Calling the soap_call_ns__sub success。 ");
printf("%d - %d = %d ",num1,num2,result);
}
iRet = soap_call_ns__mult(CalculateSoap,server_addr,"",num1,num2,&result);
if ( iRet == SOAP_ERR)
{
printf("Error while calling the soap_call_ns__mult");
}
else
{
printf("Calling the soap_call_ns__mult success。 ");
printf("%d * %d = %d ",num1,num2,result);
}
iRet = soap_call_ns__divid(CalculateSoap,server_addr,"",num1,num2,&result);
if ( iRet == SOAP_ERR)
{
printf("Error while calling the soap_call_ns__divid");
}
else
{
printf("Calling the soap_call_ns__divid success。 ");
printf("%d / %d = %d ",num1,num2,result);
}
soap_end(CalculateSoap);
soap_done(CalculateSoap);
free(CalculateSoap);
#include "gservice.nsmap"
int main( int argc, char *argv[])
{
printf("The Client is runing... ");
int num1 = 110;
int num2 = 11;
int result = 0;
struct soap *CalculateSoap = soap_new();
//soap_init(CalculateSoap);
char * server_addr = "http://localhost:8080";
int iRet = soap_call_ns__add(CalculateSoap,server_addr,"",num1,num2,&result);
if ( iRet == SOAP_ERR)
{
printf("Error while calling the soap_call_ns__add");
}
else
{
printf("Calling the soap_call_ns__add success。 ");
printf("%d + %d = %d ",num1,num2,result);
}
iRet = soap_call_ns__sub(CalculateSoap,server_addr,"",num1,num2,&result);
if ( iRet == SOAP_ERR)
{
printf("Error while calling the soap_call_ns__sub");
}
else
{
printf("Calling the soap_call_ns__sub success。 ");
printf("%d - %d = %d ",num1,num2,result);
}
iRet = soap_call_ns__mult(CalculateSoap,server_addr,"",num1,num2,&result);
if ( iRet == SOAP_ERR)
{
printf("Error while calling the soap_call_ns__mult");
}
else
{
printf("Calling the soap_call_ns__mult success。 ");
printf("%d * %d = %d ",num1,num2,result);
}
iRet = soap_call_ns__divid(CalculateSoap,server_addr,"",num1,num2,&result);
if ( iRet == SOAP_ERR)
{
printf("Error while calling the soap_call_ns__divid");
}
else
{
printf("Calling the soap_call_ns__divid success。 ");
printf("%d / %d = %d ",num1,num2,result);
}
soap_end(CalculateSoap);
soap_done(CalculateSoap);
free(CalculateSoap);
return 0;
}
}
测试服务
启动服务器端gServer程序:启动客户端gClient程序: