1
using System;
2
using System.Collections.Generic;
3
using System.Linq;
4
using System.Text;
5
6
namespace Calculator
7
{
8
public delegate double MathFunction(double a,double b);
9
//主程序代码
10
class CalTest
11
{
12
static void Main()
13
{
14
Console .Write ("请输入数值a :");
15
Double a=double .Parse (Console .ReadLine ());
16
Console .Write ("请输入数值b :");
17
Double b=double .Parse (Console .ReadLine ());
18
Console.WriteLine("请在下面的列表中选择函数:");
19
Console .WriteLine ("加 (1) \n减 (2) \n 乘 (3) \n除 (4) \n求余 (5) \n乘方 (6) \n开方 (7) \n对数 (8) ");
20
int iMethod=int.Parse (Console .ReadLine ());
21
MathFunction myFunc=PrimaryFunc.AFunc (iMethod );
22
Console .WriteLine ("计算结果为:{0}",myFunc (a ,b ));
23
Console .ReadLine ();
24
}
25
}
26
public class PrimaryFunc
27
{
28
public static MathFunction AFunc(int i)
29
{
30
switch (i)
31
{
32
case 1:
33
return delegate(double a, double b) { return a + b; };
34
case 2:
35
return delegate(double a, double b) { return a - b; };
36
case 3:
37
return delegate(double a, double b) { return a * b; };
38
case 4:
39
return delegate(double a, double b) { return a / b; };
40
case 5:
41
return delegate(double a, double b) { return a % b; };
42
case 6:
43
return delegate(double a, double b) { return Math .Pow (a ,b ); };
44
case 7:
45
return delegate(double a, double b) { return Math.Pow(a,1/b); };
46
case 8:
47
return delegate(double a, double b) { return Math.Log (a, b); };
48
default:
49
return delegate(double a, double b) { return 0; };
50
}
51
}
52
}
53
54
}
55
56
运行结果如下:
2

3

4

5

6

7

8

9

10

11

12

13

14

15

16

17

18

19

20

21

22

23

24

25

26

27

28

29

30

31

32

33

34

35

36

37

38

39

40

41

42

43

44

45

46

47

48

49

50

51

52

53

54

55

56

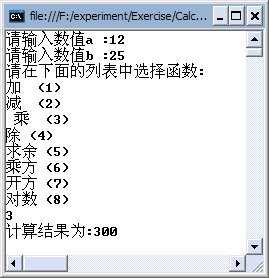