前言
记录下最近使用Python
操作Excel
的方法
读取Excel实现列拼接写入数据
- 原数据
- 实现效果
- 安装
pandas
pip install pandas
excel_handle.py
# coding=utf-8
"""
拼接excel中的列写入新列
"""
import pandas as pd
def handle_excel(file):
df = pd.read_excel(file) # 读取excel
print(df)
col_names = df.columns.tolist() # 列名
print(col_names)
# 拼接日期时间
df['date_time'] = df.apply(lambda x: str(x[5])[:11] + str(x[6]), axis=1)
print(df)
# 修改列顺序
date_time = df['date_time']
df.drop(labels=['date_time'], axis=1, inplace=True)
df.insert(7, '日期时间', date_time)
print(df)
# 计算总和
df.insert(5, '总和', df[col_names[0]] + df[col_names[1]] + df[col_names[2]] + df[col_names[3]] + df[col_names[4]], True)
print(df)
# 写入数据
df.to_excel(file, index=False)
if __name__ == '__main__':
my_file = r'test.xls'
handle_excel(my_file)
读取Excel中的数据写入新的Excel
- 读取第一行前五列写入新的
Excel
- 写入效果
- 安装
openpyxl
pip install openpyxl
excel_splice.py
# coding=utf-8
"""
截取Excel数据从新写入
"""
import pandas as pd
from openpyxl.reader.excel import load_workbook
from openpyxl import Workbook
# 创建excel
def create_excel():
wb = Workbook()
wb.create_sheet('Sheet1',0)
wb.save('test1.xlsx')
# 读取原数据
def read_create_data():
return pd.read_excel('test.xls')
# 处理excel
def handle_excel():
df = read_create_data()
# print(df)
data = df.iloc[:, 0:5] # 取前5列
# 取第一行数据写入
insert_data(data[:1], 'test1.xlsx')
# 写入数据
def insert_data(data, file_name):
wb = load_workbook(file_name)
ws = wb["Sheet1"]
print(ws)
print(data)
# 写入列名
col_names = data.columns.tolist()
for index in range(len(col_names)):
print(col_names[index])
ws.cell(row=1, column=index + 1, value=col_names[index])
# 写入数据
for index, row in data.iterrows():
for column in range(len(row)):
ws.cell(row=index + 2, column=column + 1, value=row[column])
# 保存
wb.save(file_name)
if __name__ == '__main__':
# create_excel()
handle_excel()
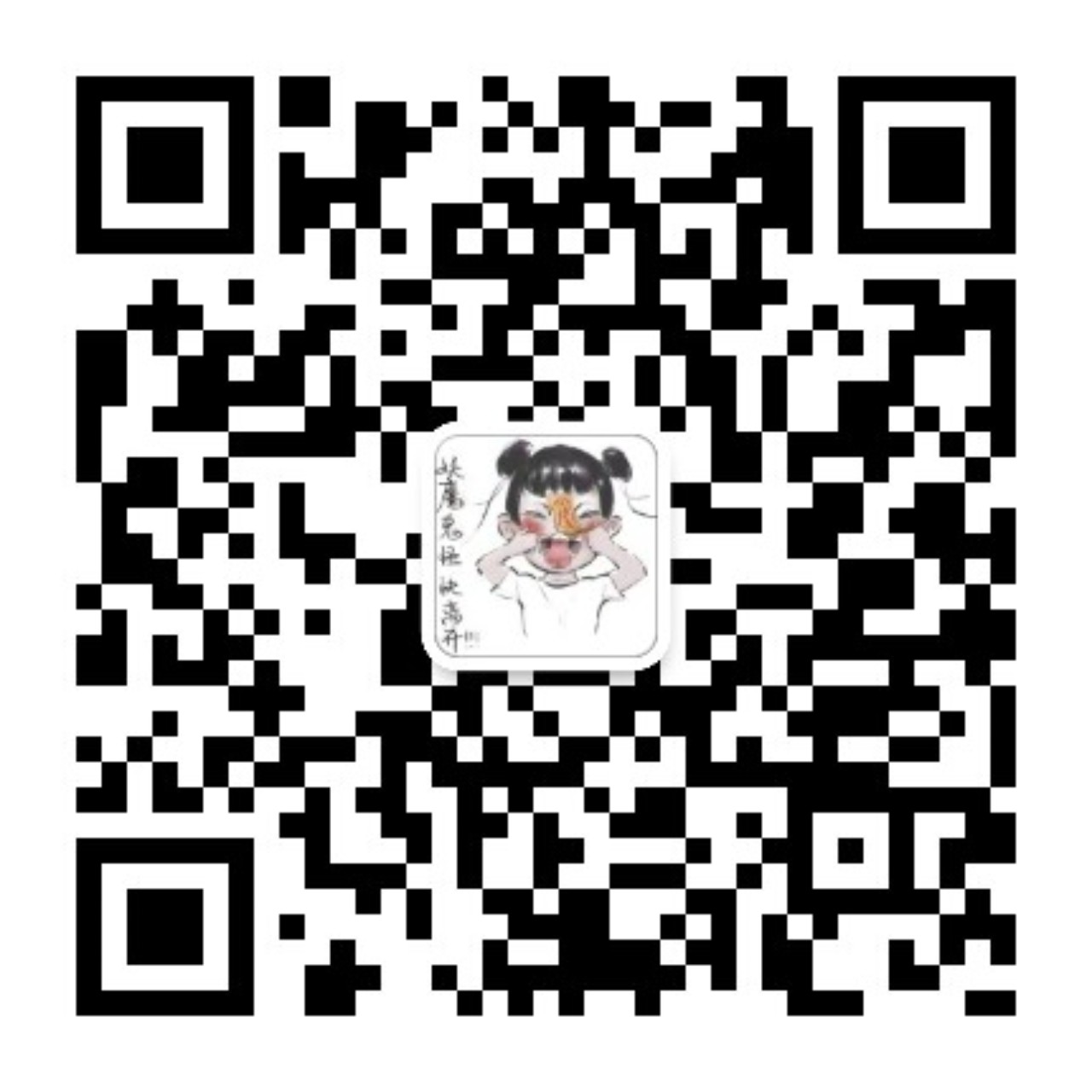