项目目录
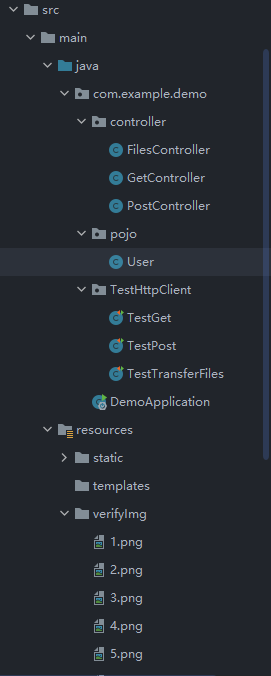
实体类
package com.example.demo.pojo;
import lombok.Data;
/**
* @author lyd
* @Description: 用户实体类
* @date 11:55
*/
@Data
public class User {
private String name;
private Integer age;
private String gender;
private String motto;
}
三种Get的实现方式测试类
package com.example.demo.TestHttpClient;
import org.apache.http.HttpEntity;
import org.apache.http.NameValuePair;
import org.apache.http.client.config.RequestConfig;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.client.utils.URIBuilder;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClientBuilder;
import org.apache.http.message.BasicNameValuePair;
import org.apache.http.util.EntityUtils;
import org.junit.Test;
import java.io.IOException;
import java.net.URI;
import java.net.URISyntaxException;
import java.util.ArrayList;
import java.util.List;
/**
* @author lyd
* @Description: Get测试
* @date 10:21
*/
public class TestGet {
/**
* 无参测试
*/
@Test
public void doGetTestOne() {
// 创建Http客户端
CloseableHttpClient httpClient = HttpClientBuilder.create().build();
// 创建Get请求
HttpGet httpGet = new HttpGet("http://localhost:8080/doGetTestOne");
// 响应模型
CloseableHttpResponse response = null;
try {
// 客户端发送Get请求
response = httpClient.execute(httpGet);
// 从响应模型中获取响应实体
HttpEntity responseEntity = response.getEntity();
System.out.println("响应状态为:" + response.getStatusLine());
if (responseEntity != null) {
System.out.println("响应内容长度为:" + responseEntity.getContentLength());
System.out.println("响应内容为:" + responseEntity);
System.out.println("响应内容为:" + EntityUtils.toString(responseEntity));
}
} catch (IOException e) {
e.printStackTrace();
} finally {
// 释放资源
try {
if (httpClient != null) {
httpClient.close();
}
if (response != null) {
response.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
/**
* 有参测试:直接拼接url
*/
@Test
public void doGetTestWayOne() {
// 参数
StringBuffer params = new StringBuffer();
params.append("name=liu");
params.append("&");
params.append("age=24");
// 创建Http客户端
CloseableHttpClient httpClient = HttpClientBuilder.create().build();
// 创建Get请求
HttpGet httpGet = new HttpGet("http://localhost:8080/doGetTestWayOne?" + params);
// 响应模型
CloseableHttpResponse response = null;
try {
// 配置信息
RequestConfig requestConfig = RequestConfig.custom()
// 设置连接超时时间
.setConnectTimeout(5000)
// 设置请求超时时间
.setConnectionRequestTimeout(5000)
// 设置读写超时时间
.setSocketTimeout(5000)
// 设置是否允许重定向
.setRedirectsEnabled(true).build();
// 添加配置信息
httpGet.setConfig(requestConfig);
// 客户端发送Get请求
response = httpClient.execute(httpGet);
// 从响应模型中获取响应实体
HttpEntity responseEntity = response.getEntity();
System.out.println("响应状态为:" + response.getStatusLine());
if (responseEntity != null) {
System.out.println("响应内容长度为:" + responseEntity.getContentLength());
System.out.println("响应内容为:" + responseEntity);
System.out.println("响应内容为:" + EntityUtils.toString(responseEntity));
}
} catch (IOException e) {
e.printStackTrace();
} finally {
// 释放资源
try {
if (httpClient != null) {
httpClient.close();
}
if (response != null) {
response.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
/**
* 有参测试:动态生成url
*/
@Test
public void doGetTestWayTwo() {
// 参数
List<NameValuePair> params = new ArrayList<>();
params.add(new BasicNameValuePair("name", "liu"));
params.add(new BasicNameValuePair("age", "12"));
/**
* URL是一种具体的URI,它是URI的一个子集,它不仅唯一标识资源,而且还提供了定位该资源的信息
*/
URI uri = null;
try {
uri = new URIBuilder()
.setScheme("http")
.setHost("localhost")
.setPort(8080)
.setPath("/doGetTestWayTwo")
.setParameters(params)
.build();
} catch (URISyntaxException e) {
e.printStackTrace();
}
// 创建Http客户端
CloseableHttpClient httpClient = HttpClientBuilder.create().build();
// 创建Get请求
HttpGet httpGet = new HttpGet(uri);
// 响应模型
CloseableHttpResponse response = null;
try {
// 配置信息
RequestConfig requestConfig = RequestConfig.custom()
// 设置连接超时时间
.setConnectTimeout(5000)
// 设置请求超时时间
.setConnectionRequestTimeout(5000)
// 设置读写超时时间
.setSocketTimeout(5000)
// 设置是否允许重定向
.setRedirectsEnabled(true).build();
// 添加配置信息
httpGet.setConfig(requestConfig);
// 客户端发送Get请求
response = httpClient.execute(httpGet);
// 从响应模型中获取响应实体
HttpEntity responseEntity = response.getEntity();
System.out.println("响应状态为:" + response.getStatusLine());
if (responseEntity != null) {
System.out.println("响应内容长度为:" + responseEntity.getContentLength());
System.out.println("响应内容为:" + responseEntity);
System.out.println("响应内容为:" + EntityUtils.toString(responseEntity));
}
} catch (IOException e) {
e.printStackTrace();
} finally {
// 释放资源
try {
if (httpClient != null) {
httpClient.close();
}
if (response != null) {
response.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
三个Get请求对应的三个地址
package com.example.demo.controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
/**
* @author lyd
* @Description: Get对应接收示例
* @date 10:38
*/
@RestController
public class GetController {
@RequestMapping("doGetTestOne")
public String doGetTestOne(){
return "123";
}
@RequestMapping("doGetTestWayOne")
public String doGetTestWayOne(String name,Integer age){
return name+age+"岁";
}
@RequestMapping("doGetTestWayTwo")
public String doGetTestWayTwo(String name,Integer age){
return name+age+"岁";
}
}
两种Post的实现方式测试类
package com.example.demo.TestHttpClient;
import com.alibaba.fastjson.JSON;
import com.example.demo.pojo.User;
import org.apache.http.HttpEntity;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClientBuilder;
import org.apache.http.util.EntityUtils;
import org.junit.Test;
import java.io.IOException;
/**
* @author lyd
* @Description: Post测试
* @date 11:32
*/
public class TestPost {
/**
* Post无参测试
*/
@Test
public void doPostTestOne() {
// 创建Http客户端
CloseableHttpClient closeableHttpClient = HttpClientBuilder.create().build();
// 创建Post请求
HttpPost httpPost = new HttpPost("http://localhost:8080/doPostTestOne");
// 响应模型
CloseableHttpResponse response = null;
try {
// 客户端发送Get请求
response = closeableHttpClient.execute(httpPost);
// 从响应模型中获取响应实体
HttpEntity responseEntity = response.getEntity();
System.out.println("响应状态为:" + response.getStatusLine());
if (responseEntity != null) {
System.out.println("响应内容长度为:" + responseEntity.getContentLength());
System.out.println("响应内容为:" + responseEntity);
System.out.println("响应内容为:" + EntityUtils.toString(responseEntity));
}
} catch (IOException e) {
e.printStackTrace();
} finally {
// 释放资源
try {
if (closeableHttpClient != null) {
closeableHttpClient.close();
}
if (response != null) {
response.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
/**
* Post对象参数测试
*/
@Test
public void doPostTestTwo() {
// 创建Http客户端
CloseableHttpClient closeableHttpClient = HttpClientBuilder.create().build();
// 创建Post请求
HttpPost httpPost = new HttpPost("http://localhost:8080/doPostTestTwo");
User user = new User();
user.setName("liuliu");
user.setGender("boy");
user.setMotto("要帅");
user.setAge(13);
String jsonStr = JSON.toJSONString(user);
StringEntity stringEntity = new StringEntity(jsonStr,"utf8");
httpPost.setHeader("Content-Type","application/json;charset=utf8");
httpPost.setEntity(stringEntity);
// 响应模型
CloseableHttpResponse response = null;
try {
// 客户端发送Get请求
response = closeableHttpClient.execute(httpPost);
// 从响应模型中获取响应实体
HttpEntity responseEntity = response.getEntity();
System.out.println("响应状态为:" + response.getStatusLine());
if (responseEntity != null) {
System.out.println("响应内容长度为:" + responseEntity.getContentLength());
System.out.println("响应内容为:" + responseEntity);
System.out.println("响应内容为:" + EntityUtils.toString(responseEntity));
}
} catch (IOException e) {
e.printStackTrace();
} finally {
// 释放资源
try {
if (closeableHttpClient != null) {
closeableHttpClient.close();
}
if (response != null) {
response.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
Post测试对应的两个地址
package com.example.demo.controller;
import com.example.demo.pojo.User;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RestController;
/**
* @author lyd
* @Description: Post
* @date 11:38
*/
@RestController
public class PostController {
@RequestMapping(value = "/doPostTestOne",method = RequestMethod.POST)
public String doPostTestOne(){
return "这个Post请求没有任何参数";
}
/**
* 对象参数
* @return
*/
@RequestMapping(value = "/doPostTestTwo",method = RequestMethod.POST)
public String doPostTestTwo(@RequestBody User user){
return user.toString();
}
}
发送文件测试类
package com.example.demo.TestHttpClient;
import org.apache.http.HttpEntity;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.ContentType;
import org.apache.http.entity.mime.MultipartEntityBuilder;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClientBuilder;
import org.apache.http.util.EntityUtils;
import org.junit.Test;
import java.io.File;
import java.io.IOException;
import java.io.UnsupportedEncodingException;
import java.net.URLEncoder;
import java.nio.charset.Charset;
/**
* @author lyd
* @Description: 测试发送文件
* @date 13:58
*/
public class TestTransferFiles {
@Test
public void transferFiles() {
CloseableHttpClient closeableHttpClient = HttpClientBuilder.create().build();
HttpPost httpPost = new HttpPost("http://localhost:8080/transferFiles");
CloseableHttpResponse closeableHttpResponse = null;
MultipartEntityBuilder multipartEntityBuilder = MultipartEntityBuilder.create();
// 多个文件可以同使用一个key,后端用集合或数组接收即可
String filesKey = "files";
// 第一个文件
File file1 = new File("D:\IdeaProjects\test-HttpClient\src\main\resources\verifyImg\1.png");
multipartEntityBuilder.addBinaryBody(filesKey, file1);
// 第二个文件
File file2 = new File("D:\IdeaProjects\test-HttpClient\src\main\resources\verifyImg\2.png");
try {
// 防止服务端收到的文件名乱码。 我们这里可以先将文件名URLEncode,然后服务端拿到文件名时在URLDecode。就能避免乱码问题。
multipartEntityBuilder.addBinaryBody(filesKey, file2, ContentType.DEFAULT_BINARY, URLEncoder.encode(file2.getName(), "utf8"));
} catch (UnsupportedEncodingException e) {
e.printStackTrace();
}
// text/plain的意思是将文件设置为纯文本的形式,浏览器在获取到这种文件时并不会对其进行处理。
ContentType contentType = ContentType.create("text/plain", Charset.forName("UTF-8"));
multipartEntityBuilder.addTextBody("name", "关羽", contentType);
multipartEntityBuilder.addTextBody("age", "20", contentType);
HttpEntity httpEntity = multipartEntityBuilder.build();
httpPost.setEntity(httpEntity);
try {
// 客户端发送post请求
closeableHttpResponse = closeableHttpClient.execute(httpPost);
// 从响应模型中获取响应实体
HttpEntity responseEntity = closeableHttpResponse.getEntity();
System.out.println("响应状态为:" + closeableHttpResponse.getStatusLine());
if (responseEntity != null) {
System.out.println("响应内容长度为:" + responseEntity.getContentLength());
System.out.println("响应内容为:" + responseEntity);
System.out.println("响应内容为:" + EntityUtils.toString(responseEntity));
}
} catch (IOException e) {
e.printStackTrace();
} finally {
// 释放资源
try {
if (closeableHttpClient != null) {
closeableHttpClient.close();
}
if (closeableHttpResponse != null) {
closeableHttpResponse.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
接收文件的地址
package com.example.demo.controller;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.multipart.MultipartFile;
import java.util.List;
/**
* @author lyd
* @Description: 传送文件测试
* @date 14:19
*/
@RestController
public class FilesController {
@PostMapping(value = "/transferFiles")
public String transferFiles(@RequestParam String name,
@RequestParam Integer age,
@RequestParam List<MultipartFile> multipartFiles) {
StringBuilder stringBuilder = new StringBuilder(64);
stringBuilder
.append("name=")
.append(name)
.append(",age=")
.append(age);
String fileName;
for (MultipartFile file : multipartFiles) {
stringBuilder.append("
文件信息:
");
fileName = file.getOriginalFilename();
if (fileName == null) {
continue;
}
stringBuilder.append("文件名:" + fileName);
stringBuilder.append("
文件类型:" + file.getContentType());
}
return stringBuilder.toString();
}
}
上面有用到的实体类
package com.example.demo.pojo;
import lombok.Data;
/**
* @author lyd
* @Description: 用户实体类
* @date 11:55
*/
@Data
public class User {
private String name;
private Integer age;
private String gender;
private String motto;
}
项目源码
https://github.com/Wranglery/test-HttpClient