一、栈的应用——迷宫问题
1、解题思路
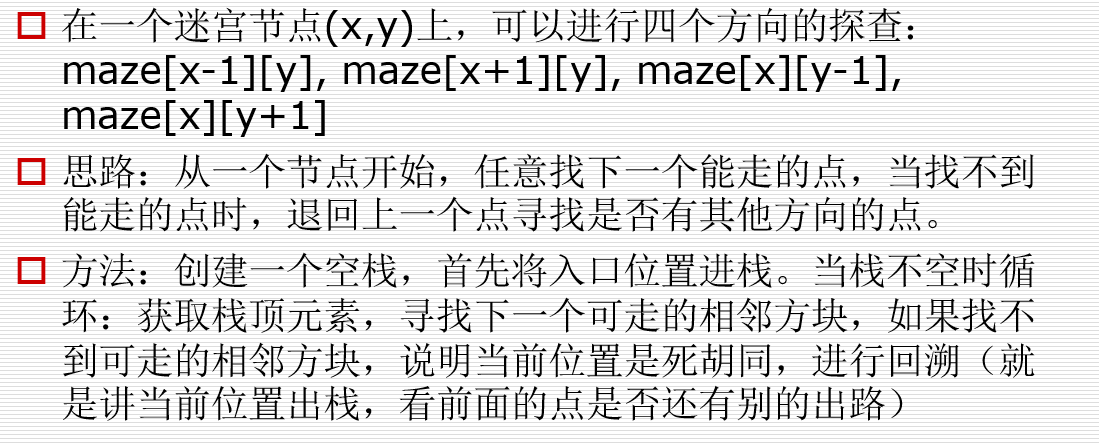
2、实现代码
import time
from collections import deque
maze = [
[1,1,1,1,1,1,1,1,1,1],
[1,0,0,1,0,0,0,1,0,1],
[1,0,0,1,0,0,0,1,0,1],
[1,0,0,0,0,1,1,0,0,1],
[1,0,1,1,1,0,0,0,0,1],
[1,0,0,0,1,0,0,0,0,1],
[1,0,1,0,0,0,1,0,0,1],
[1,0,1,1,1,0,1,1,0,1],
[1,1,0,0,0,0,0,1,0,1],
[1,1,1,1,1,1,1,1,1,1]
]
dirs = [lambda x, y: (x + 1, y),
lambda x, y: (x - 1, y),
lambda x, y: (x, y - 1),
lambda x, y: (x, y + 1)]
def mpath(x1, y1, x2, y2):
stack = []
stack.append((x1, y1))
while len(stack) > 0: #当栈不空循环
cur_node = stack[-1]
if cur_node[0] == (x2,y2): #到达终点
for p in stack:
print(p)
return True
for dir in dirs:
next_node = dir(*cur_node)
if maze[next_node[0]][next_node[1]] == 0: #找到了一个能走的方向
stack.append(next_node)
maze[next_node[0]][next_node[1]] = 2 # 2标记为已经走过,防止死循环
break
else:#如果一个方向都没找到
maze[curNode[0]][curNode[1]] = -1 # 死路一条,下次别走了
stack.pop() #回溯
else:
print("没有路")
return False
二、队列的应用——迷宫问题
1、解题思路
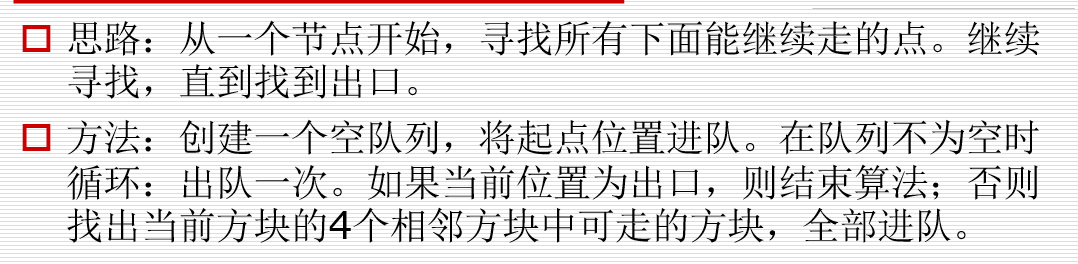
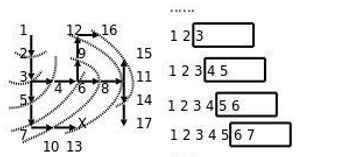
2、实现代码
import time
from collections import deque
maze = [
[1,1,1,1,1,1,1,1,1,1],
[1,0,0,1,0,0,0,1,0,1],
[1,0,0,1,0,0,0,1,0,1],
[1,0,0,0,0,1,1,0,0,1],
[1,0,1,1,1,0,0,0,0,1],
[1,0,0,0,1,0,0,0,0,1],
[1,0,1,0,0,0,1,0,0,1],
[1,0,1,1,1,0,1,1,0,1],
[1,1,0,0,0,0,0,1,0,1],
[1,1,1,1,1,1,1,1,1,1]
]
dirs = [lambda x, y: (x + 1, y),
lambda x, y: (x - 1, y),
lambda x, y: (x, y - 1),
lambda x, y: (x, y + 1)]
def solve_maze2(x1,y1,x2,y2):
queue = deque()
path = [] #记入出队之后的节点
queue.append(x1,y1,-1)
maze[x1][y1] = 2
while len(queue) > 0:
cur_node = queue.popleft()
path.append(cur_node)
if cur_node[0] == x2 and cur_node[1] == y2: #找到终点
real_path = []
x,y,i = path[-1]
real_path.append((x,y))
while i >= 0:
node = path[i]
real_path.append(node[0:2])
i = node[2]
real_path.reverse()
for p in real_path:
print(p)
return True
for dir in dirs:
next_node =dir(cur_node[0],cur_node[1])
if maze[next_node[0]][next_node[1]] == 0:
queue.append(next_node[0],next_node[1],len((path)-1))
maze[next_node[0]][next_node[1]] = 2 #标记已经走过
else:
print("无路可走")
mpath(1,1,8,8)