一 登录案例认证
回顾装饰器
# 1. 装饰器 def auth(func): def inner(*args,**kwargs): print("前") ret = func(*args,**kwargs) print("后") return ret return inner @auth def index(): print('index') index() @auth def detail(): print('detail') detail() print(index.__name__) #打印的函数名 inner print(detail.__name__) #打印的函数名 inner # 执行结果: # 前 # index # 后 # 前 # detail # 后 # inner # inner
# functools.wraps的特点: # # 被装饰函数不受装饰器的影响 # 装饰器的名字变成被装饰名字,外部调用到的被装饰函数的功能 # 1. 装饰器 import functools def auth(func): @functools.wraps(func) # 加上这装饰器会把原来的函数所有信息放入 inner中 伪装的更彻底 但是打印的就会变成被装饰的函数名 def inner(*args,**kwargs): ret = func(*args,**kwargs) return ret return inner @auth def index(): print('index') @auth def detail(): print('detail') print(index.__name__) # index print(detail.__name__) # detail
1.装饰器认证 session(版本一 )
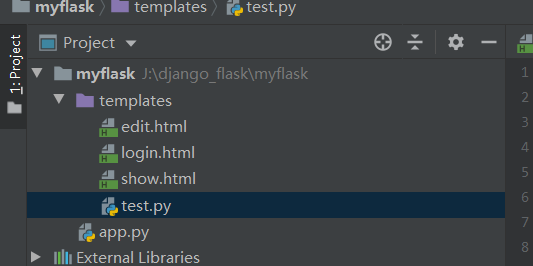
login.html
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Title</title> </head> <body> <h1>欢迎来到登录页面哈哈哈哈</h1> <form method="post"> <p>用户: <input type="text" name="user"></p> <p>密码: <input type="password" name="pwd"></p> <p>用户: <input type="submit" value="登录"></p> <p>{{error}}</p> </form> </body> </html>
show.html
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>show</title> </head> <body> <table border="1"> <!-- border="1" 显示边框 --> <!-- 表格头部 --> <thead> <tr> <!-- 行 --> <th>ID</th> <th>姓名:</th> <th>年龄:</th> <th>姓别:</th> <th>操作:</th> </tr> </thead> <tbody> {% for k,v in dict_data.items() %} <tr> <td>{{ k }}</td> <td>{{ v.get("name")}}</td> <td>{{ v.age}}</td> <td>{{ v.gender}}</td> <td><a href="/dels/{{k}}"/>删除</a> | <a href="/detall/{{k}}/">查看详情</a></td> </tr> {% endfor %} </tbody> </table> </body> </html>
edit.html
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>show</title> </head> <body> <table border="1"> <!-- border="1" 显示边框 --> <!-- 表格头部 --> <thead> <tr> <!-- 行 --> <th>ID</th> <th>姓名:</th> <th>年龄:</th> <th>姓别:</th> <th>操作:</th> </tr> </thead> <tbody> {% for k,v in dict_data.items() %} <tr> <td>{{ k }}</td> <td>{{ v.get("name")}}</td> <td>{{ v.age}}</td> <td>{{ v.gender}}</td> <td><a href="/dels/{{k}}"/>删除</a> | <a href="/detall/{{k}}/">查看详情</a></td> </tr> {% endfor %} </tbody> </table> </body> </html>
app.py
from flask import Flask, request,jsonify,json,render_template,redirect,url_for,session app=Flask(__name__) list_data = { 1:{'name':'王龙泰','age':38,'gender':'中'}, 2:{'name':'小东北','age':73,'gender':'男'}, 3:{'name':'田硕','age':84,'gender':'男'}, } app.secret_key="aswdasdsad" # 认证装饰器 # functools.wraps的特点: # 被装饰函数不受装饰器的影响 # 装饰器的名字变成被装饰名字,外部调用到的被装饰函数的功能 import functools def auth(func): @functools.wraps(func) # 加上这装饰器会把原来的函数所有信息放入 inner中 伪装的更彻底 但是打印的就会变成被装饰的函数名 # 不加 会报错AssertionError: View function mapping is overwriting an existing endpoint function: inner def inner(*args,**kwargs): if not session.get("username"): return redirect(url_for("myapp")) ret=func(*args,**kwargs) return ret return inner @app.route("/login/",methods=["GET","POST"]) def myapp(): if request.method=="GET": return render_template("login.html") # 默认是get请求 user=request.form.get("user") pwd= request.form.get("pwd") print(user,pwd,"这是获取值") if user=="aaa" and pwd=="111": session["username"]=user # 设置session # return "登录成功了哈哈哈" return redirect("/home/") return render_template("login.html",error="你的用户密码错误了哈哈哈哈") # 方法一传参数 单个形式 # return render_template("login.html", **{"error":"你的用户密码错误了哈哈哈哈"}) # 方法二传参数 字典形式 @app.route("/home/",methods=["GET","POST"]) @auth def index(): print(url_for("index")) return render_template("show.html",dict_data=list_data) @app.route("/dels/<int:nid>/") @auth def dels(nid): del list_data[nid] return redirect(url_for("index")) @app.route("/detall/<int:nid>/") @auth def detall(nid): info=list_data[nid] return render_template("edit.html",info=info) if __name__=="__main__": app.run()![]()
![]()
2. 使用before_request装饰器(版本二简介 )
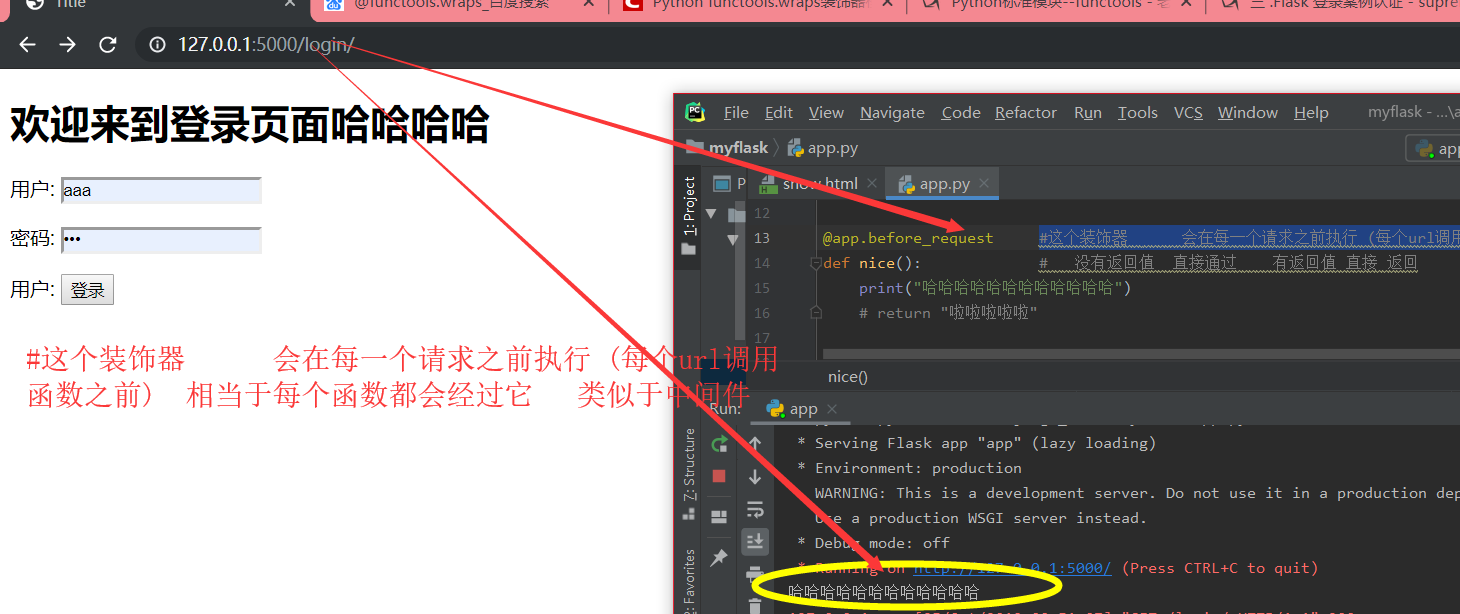
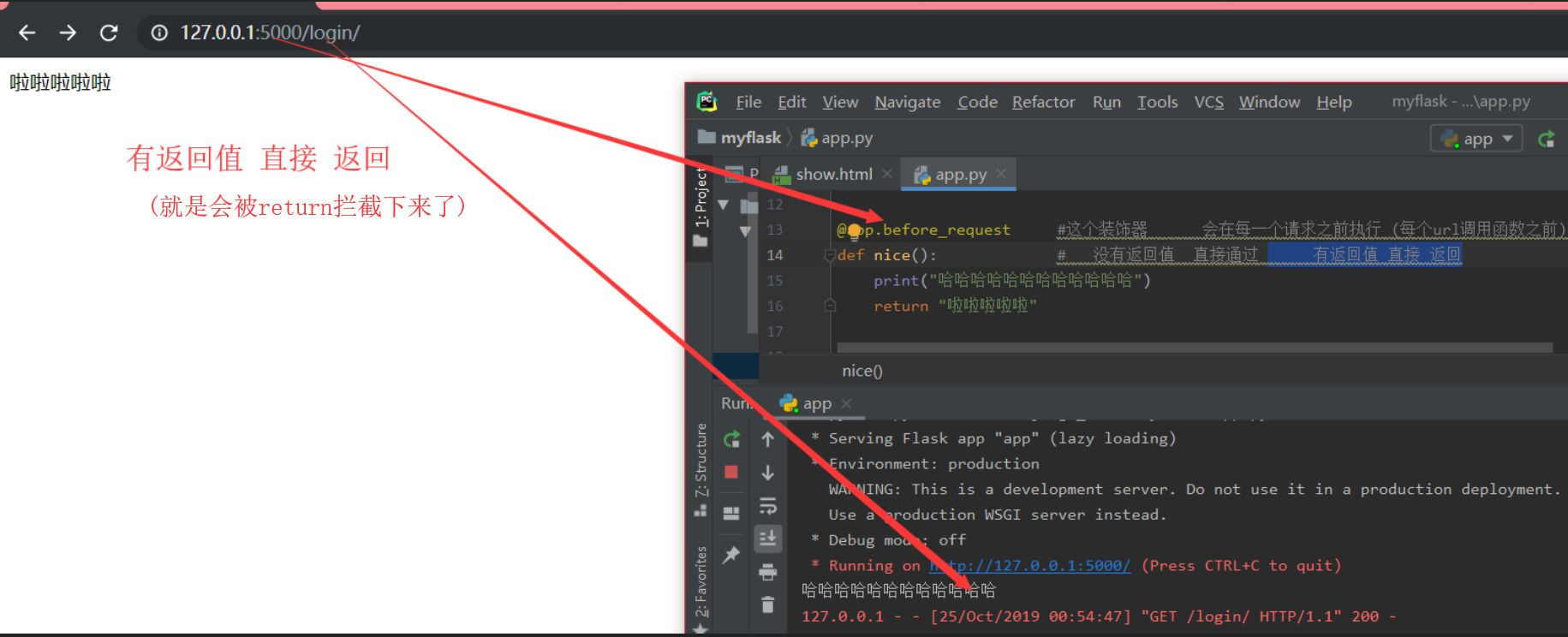
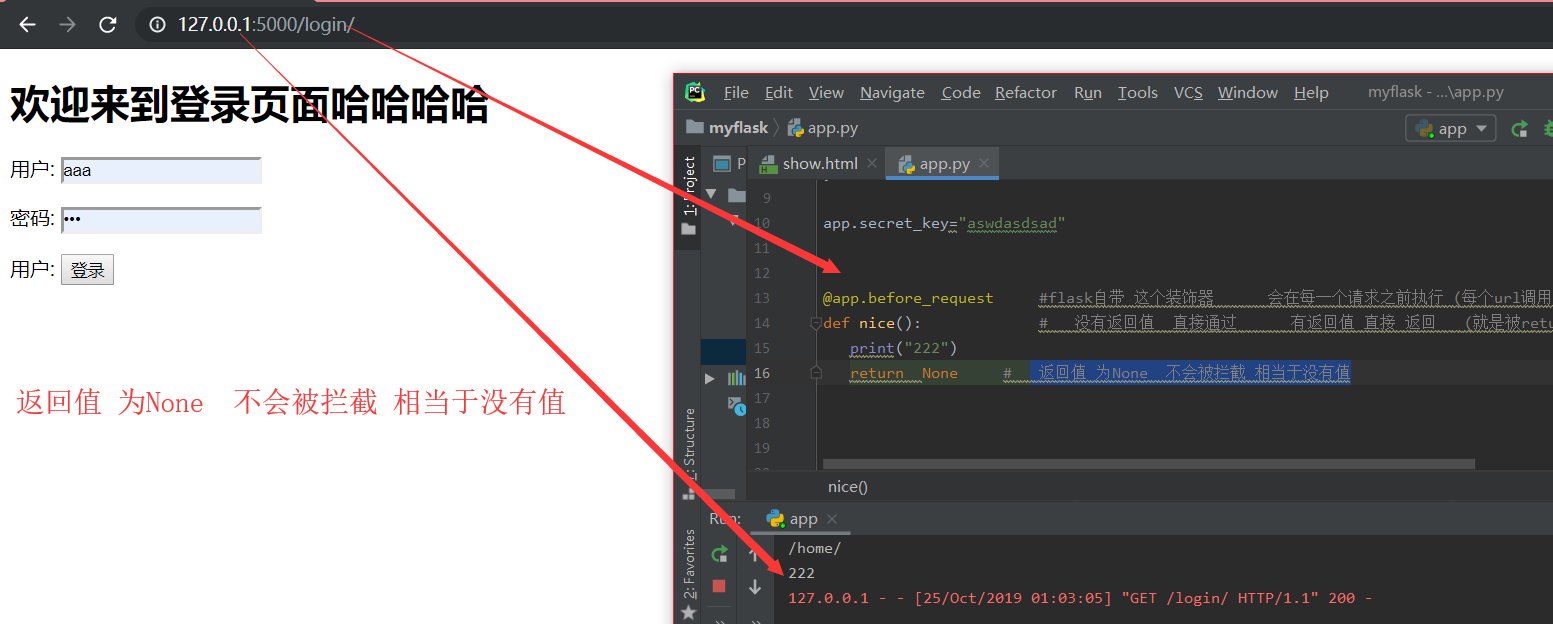
from flask import Flask, request,jsonify,json,render_template,redirect,url_for,session app=Flask(__name__) list_data = { 1:{'name':'王龙泰','age':38,'gender':'中'}, 2:{'name':'小东北','age':73,'gender':'男'}, 3:{'name':'田硕','age':84,'gender':'男'}, } app.secret_key="aswdasdsad" @app.before_request #flask自带 这个装饰器 会在每一个请求之前执行 (每个url调用函数之前) 相当于每个函数都会经过它 类似于中间件 def nice(): # 没有返回值 直接通过 有返回值且返回值有值 直接 返回 (就是被return拦截了) if request.path=="/login/": return None # 返回值为None 相当于没有值 直接通过 也不会拦截 if session.get("username"): return None return redirect("/login/") @app.route("/login/",methods=["GET","POST"]) def myapp(): if request.method=="GET": return render_template("login.html") # 默认是get请求 user=request.form.get("user") pwd= request.form.get("pwd") print(user,pwd,"这是获取值") if user=="aaa" and pwd=="111": session["username"]=user # 设置session # return "登录成功了哈哈哈" return redirect("/home/") return render_template("login.html",error="你的用户密码错误了哈哈哈哈") # 方法一传参数 单个形式 # return render_template("login.html", **{"error":"你的用户密码错误了哈哈哈哈"}) # 方法二传参数 字典形式 @app.route("/home/",methods=["GET","POST"]) def index(): print(url_for("index")) return render_template("show.html",dict_data=list_data) @app.route("/dels/<int:nid>/") def dels(nid): del list_data[nid] return redirect(url_for("index")) @app.route("/detall/<int:nid>/") def detall(nid): info=list_data[nid] return render_template("edit.html",info=info) if __name__=="__main__": app.run()