Luogu题地址
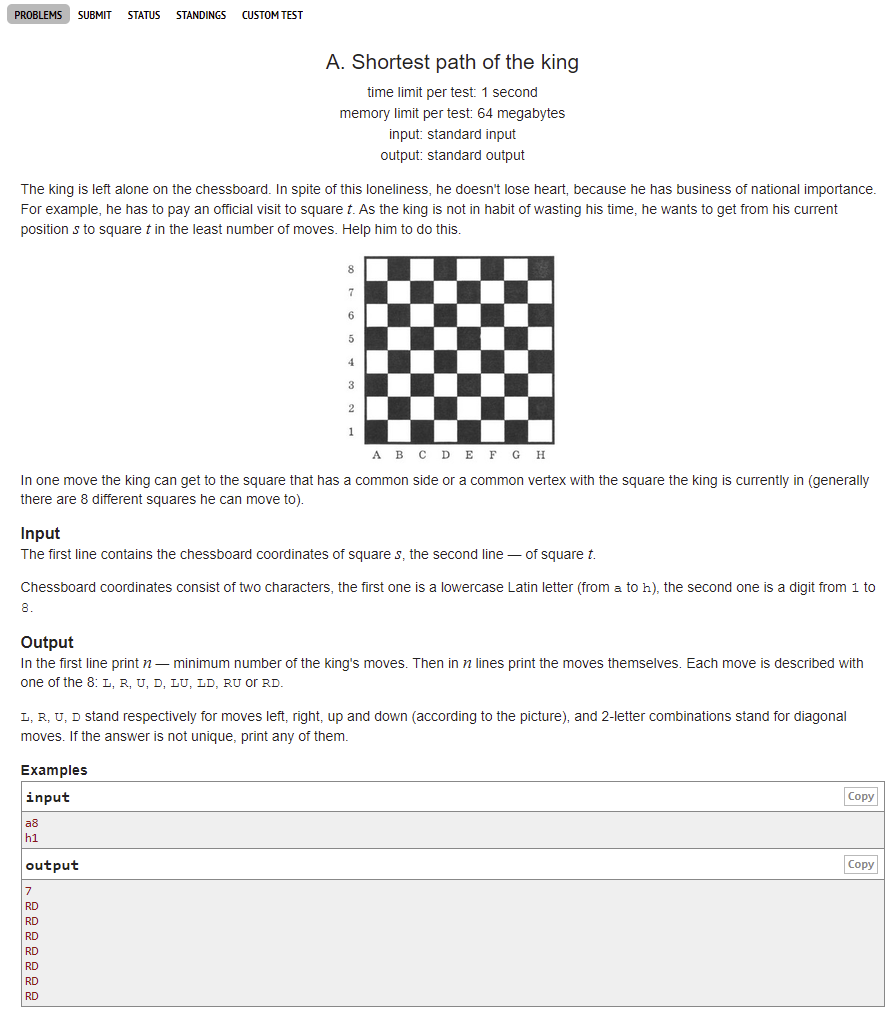


#include <iostream>
#include <cstdio>
#include <cstring>
#include <algorithm>
#include <queue>
using namespace std;
const int N = 10;
int dx[8] = {1, 1, 0, -1, -1, -1, 0, 1};
int dy[8] = {0, 1, 1, 1, 0, -1, -1, -1};
string s[8] = {"U", "RU", "R", "RD", "D", "LD", "L", "LU"};
vector<string> ans;
struct Node{
int x, y, step;
}node, S, T;
bool st[N][N];
int d[N][N];
Node q[N];
void bfs()
{
d[S.x][S.y] = 0;
queue<Node> q;
q.push(S);
st[S.x][S.y] = true;
while(q.size())
{
Node t = q.front();
q.pop();
int x = t.x, y = t.y, step = t.step;
d[x][y] = step;
for(int i = 0; i < 8; i ++)
{
int nx = x + dx[i], ny = y + dy[i];
if(nx >= 0 && nx <= 7 && ny >= 0 && ny <= 7 && !st[nx][ny])
{
node.x = nx, node.y = ny, node.step = step + 1;
q.push(node);
st[nx][ny] = true;
}
}
}
}
void find(int x, int y)
{
for(int i = 0; i < 8; i ++)
{
if(x == dx[i] && y == dy[i])
ans.push_back(s[i]);
}
}
int main()
{
char s1[3], s2[3];
cin >> s1 >> s2;
S = {s1[1] - '1', s1[0] - 'a', 0};
T = { s2[1] - '1',s2[0] - 'a', 0};
bfs();
cout << d[T.x][T.y] << endl;
int x = T.x, y = T.y;
if(d[x][y] == 0) return 0;
while(1)
{
bool flag = false;
for(int i = 0; i < 8; i ++)
{
int nx = x + dx[i], ny = y + dy[i];
if(nx < 0 || nx > 7 || ny < 0 || ny > 7) continue;
if(d[nx][ny] == 0)
{
find(x - nx, y - ny);
flag = true;
break;
}
if(d[nx][ny] == d[x][y] - 1)
{
find(x - nx, y - ny);
x = nx, y = ny;
continue;
}
}
if(flag == true) break;
}
int len = ans.size() - 1;
for(int i = len; i >= 0; i --)
cout << ans[i] << endl;
}