Computer
Time Limit: 1000/1000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others)
Total Submission(s): 5194 Accepted Submission(s): 2620
Problem Description
A
school bought the first computer some time ago(so this computer's id is
1). During the recent years the school bought N-1 new computers. Each
new computer was connected to one of settled earlier. Managers of school
are anxious about slow functioning of the net and want to know the
maximum distance Si for which i-th computer needs to send signal (i.e.
length of cable to the most distant computer). You need to provide this
information.
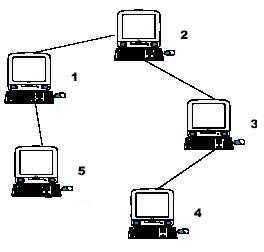
Hint: the example input is corresponding to this graph. And from the graph, you can see that the computer 4 is farthest one from 1, so S1 = 3. Computer 4 and 5 are the farthest ones from 2, so S2 = 2. Computer 5 is the farthest one from 3, so S3 = 3. we also get S4 = 4, S5 = 4.
Hint: the example input is corresponding to this graph. And from the graph, you can see that the computer 4 is farthest one from 1, so S1 = 3. Computer 4 and 5 are the farthest ones from 2, so S2 = 2. Computer 5 is the farthest one from 3, so S3 = 3. we also get S4 = 4, S5 = 4.
Input
Input
file contains multiple test cases.In each case there is natural number N
(N<=10000) in the first line, followed by (N-1) lines with
descriptions of computers. i-th line contains two natural numbers -
number of computer, to which i-th computer is connected and length of
cable used for connection. Total length of cable does not exceed 10^9.
Numbers in lines of input are separated by a space.
Output
For each case output N lines. i-th line must contain number Si for i-th computer (1<=i<=N).
Sample Input
5
1 1
2 1
3 1
1 1
Sample Output
3
2
3
4
4
Author
scnu
题意:求树上每个点到离它最远的点的距离.(方法一难懂,方法二我也不知道为什么是正确的,没办法证明)
方法一:
分析:要求树上每个点能够到达的最远距离,那么最远距离这个值有两个来源,分别是来自其子树或者来自其父亲,我们先用一次深搜将每棵树的子树遍历,求出子树的最大值和次
大值并且两个值所在的分支不同,为什么需要求出次大值呢?这里就是本题的精华了:在第二次DFS时,如果父亲在子树能够到达的最远距离和当前要求解的子结点在同一条分支上,那么我们就肯定不能选这条了,而应该选其"兄弟"结点中的最长路,也就是次短路.(这一点需要画图好好理解)
dp[i][0]代表以i为根子树中的最长路,dp[i][1]代表子树中的次长路,dp[i][2]代表父亲树中的最长路,设i的父亲为fi
dp[i][2] = max(dp[fi][2] , dp[i][0]+dis[i][fi]==dp[fi][0]?dp[fi][1]:dp[fi][0]) + dis[i][fi];
///题意:求树上每个点到离它最远的点的距离. #include <iostream> #include <stdio.h> #include <string.h> #include <algorithm> #define N 10050 using namespace std; struct Edge{ int u,v,w,next; }edge[2*N]; int head[N]; int dp[N][3]; ///dp[i][0]代表以i为根子树中的最长路,dp[i][1]代表子树中的次长路,dp[i][2]代表父亲树中的最长路 void addEdge(int u,int v,int w,int &k){ edge[k].u = u,edge[k].v = v,edge[k].w = w; edge[k].next = head[u],head[u]=k++; } void dfs(int u,int fa){ ///找子树中的最大值和次大值 for(int k = head[u];k!=-1;k=edge[k].next){ int v = edge[k].v; if(v==fa) continue; //printf("%d ",v); dfs(v,u); if(dp[u][1]<dp[v][0]+edge[k].w){ dp[u][1] = dp[v][0] + edge[k].w; if(dp[u][1]>dp[u][0]){ swap(dp[u][1],dp[u][0]); } } } } void dfs1(int u,int fa){///找从父亲延伸过去的最大值 for(int k = head[u];k!=-1;k=edge[k].next){ int v = edge[k].v,w = edge[k].w; if(v==fa) continue; dp[v][2] = max(dp[u][2] , dp[v][0]+edge[k].w==dp[u][0]?dp[u][1]:dp[u][0]) + edge[k].w; dfs1(v,u); } } int main() { int n; while(scanf("%d",&n)!=EOF) { memset(head,-1,sizeof(head)); memset(dp,0,sizeof(dp)); int tot=0; for(int u=2;u<=n;u++){ int v,w; scanf("%d%d",&v,&w); addEdge(u,v,w,tot); addEdge(v,u,w,tot); } dfs(1,-1); dfs1(1,-1); for(int i=1;i<=n;i++){ printf("%d ",max(dp[i][0],dp[i][2])); } } return 0; }
方法二:先对任意一个点进行搜索的到离它最远的端点,这个点必定是树的直径(树的直径指树中的最长路)的其中一个端点,然后以这个端点开始又进行搜索,得到一个离他最远
的店,这个点是直径的另外一个端点,我们在找的时候分别更新所有点到两个端点的距离,对于每个点我们区大值就是结果。
///题意:求树上每个点到离它最远的点的距离. #include <iostream> #include <stdio.h> #include <string.h> #include <algorithm> #include <queue> #define N 10050 using namespace std; struct Edge{ int u,v,w,next; }edge[2*N]; int head[N]; void addEdge(int u,int v,int w,int &k){ edge[k].u = u,edge[k].v = v,edge[k].w = w; edge[k].next = head[u],head[u]=k++; } int dis[N],dis1[N]; int vis[N]; void BFS(int x){ memset(vis,0,sizeof(vis)); queue<int> q; q.push(x); vis[x]=1; while(!q.empty()){ int t = q.front(); q.pop(); for(int k=head[t];k!=-1;k=edge[k].next){ int v = edge[k].v,w=edge[k].w; if(!vis[v]){ vis[v]=1; q.push(v); dis[v] =max(dis[v],dis[t]+w); } } } } int main() { int n; while(scanf("%d",&n)!=EOF) { memset(head,-1,sizeof(head)); memset(dis,0,sizeof(dis)); memset(dis1,0,sizeof(dis1)); int tot=0; for(int u=2;u<=n;u++){ int v,w; scanf("%d%d",&v,&w); addEdge(u,v,w,tot); addEdge(v,u,w,tot); } BFS(1); int START=0,END=0; int len = -1; for(int i=1;i<=n;i++) { if(dis[i]>len) {len = dis[i],START = i;} } memset(dis,0,sizeof(dis)); BFS(START); len = -1; for(int i=1;i<=n;i++) { dis1[i]=dis[i]; if(dis[i]>len) {len = dis[i],END = i;} } memset(dis,0,sizeof(dis)); BFS(END); for(int i=1;i<=n;i++){ printf("%d ",max(dis[i],dis1[i])); } } return 0; }