jdbc三件事
1、连接数据库:(driver驱动,url,username,password)
2、发出sql指令:比如:查询某个表格的所有数据 sql="select * from table"
3、接受数据库的反馈结果(ResultSet)
jdbc的使用:
1、ip:本地ip:127.0.0.1(localhost)
2、sid mysql:mysql
3、端口号port: mysql:3306
4、username:root(自己设置的mysql入口用户名)
5、password:admin(自己设置的mysql入口密码)
6、驱动名称:mysql:com.mysql.jdbc.Driver 全限定名(包名+类名)(packagename + classname)
7、url连接地址:mysql:jdbc:mysql://localhost:3306/college (mysql:sid localhost:ip 3306:port college:数据库名称)
jdbc连接mysql
1、加载注册一个驱动 Class.forName
2、创建连接对象:Connection conn = DriverManager.getConnection();
3、Statement statement = conn.createStatement();
4、stament.executeQuery(sql); stament.executeUpdate(sql);
5、接收结果ResultSet int
jdbc连接数据库的代码:
*/
private static String driver = "";
private static String url = "";
private static String username = "";
private static String password = "";
private static Properties properties = new Properties();
public static void main(String[] args) throws IOException {
// 获取类加载器
ClassLoader classLoader = JDBCTest.class.getClassLoader();
// 获取输入流 (properties文件)
InputStream ips = classLoader.getResourceAsStream("jdbc.properties");
// 将输入流 加载到 properties
properties.load(ips);
driver = properties.getProperty("jdbc.driver");
url = properties.getProperty("jdbc.url");
username = properties.getProperty("jdbc.username");
password = properties.getProperty("jdbc.password");
Connection connection = null;
// Statement statement = null;
PreparedStatement preparedStatement = null;
ResultSet rs = null;
try {
Class.forName(driver);
//创建连接对象
connection = DriverManager.getConnection(url, username, password);
//发送SQL语句
String sql = "select * from t_user where username = ? and password = ?";
//对信息语句进行预处理
preparedStatement = connection.prepareStatement(sql);
preparedStatement.setString(1, "admin");
preparedStatement.setString(2, "123456");
// statement = connection.createStatement();
// 查询
rs = preparedStatement.executeQuery();
// 增加 删除 修改
// statement.executeUpdate(sql)
while (rs.next()) {
System.out.println(rs.getInt("id") + "," + rs.getString("username") + "," + rs.getString("password"));
}
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
//从下往上依次关闭
if (rs != null) {
try {
rs.close();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
if (preparedStatement != null) {
try {
preparedStatement.close();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
if (connection != null) {
try {
connection.close();
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
}
别忘了对应的jar包:
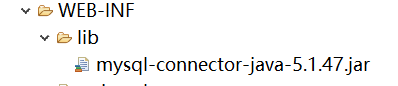
同时,要右击选择build path:
以及对应的properties文件:
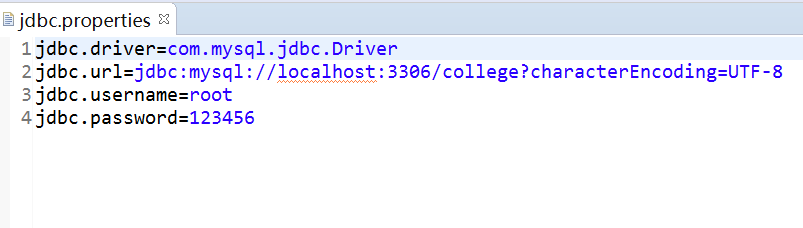
至此,运行过后系统没有报错视为连接成功。