抽奖程序源代码下载地址:
1抽奖程序效果图
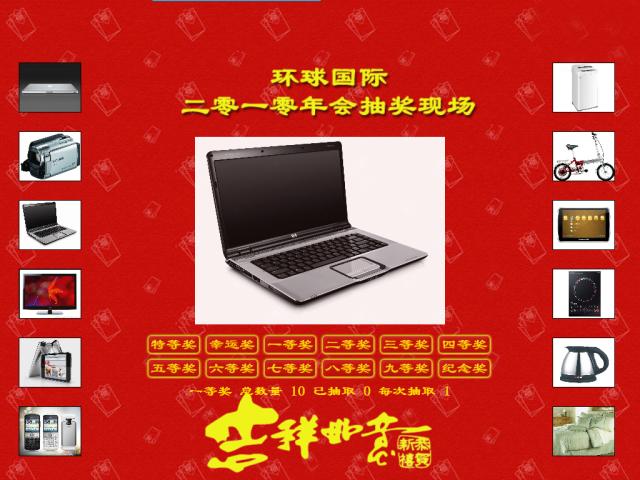
2抽奖程序功能设计
1、人员清单、奖项、抽奖记录等数据均在存储在Excel中,易于维护;2、抽奖结束时即可打印中奖人员清单及纪念奖清单,数据可排序、可筛选;3、在Excel中即可定义奖项数量、一次抽取数量、奖品图片等;4、可临时追加抽奖人数;5、每个奖项抽取完成后可显示当前奖项中奖人员清单;6、可自定义背景图片;7、限定每次按键间隔时间约5秒钟,防止误操作;8、限制抽奖数量不超过设定数量,并在屏幕上同步显示已抽取数量;9、奖品图片展示。
3 抽奖原理
人员名单来自人事,按工号升序排序,首次读入程序后,以随机方式打乱顺序,在此基础上,随机抽取。开始抽奖时,程序每隔0.01秒随机取得一个号,屏幕上每0.1秒显示一次当前随机取得的号,当停止抽奖时,程序随机抽取一个号作为中奖号码,并从人员清单中删除。
4运行环境
开发语言c#,开发工具VS2008,运行环境windows XP/VISTA、office2007、.netFrameWork3.5。
5部分代码讲解
5.1取得系统路径
String path = System.Windows.Forms.Application.StartupPath + "\\";
5.2取得电脑屏幕的宽度和高度
int screenWidth = System.Windows.Forms.Screen.GetBounds(this).Width;
int screenHeight = System.Windows.Forms.Screen.GetBounds(this).Height;
5.3设置背景图片,并设置图片自动伸缩
this.BackgroundImage = Image.FromFile(path + "bg.jpg");
this.BackgroundImageLayout = ImageLayout.Stretch;
5.4以RGB方式设置背景颜色
5.5读取setting.txt中颜色的RGB值,setting.txt内容是color=196,14,10
String color = "";
try
{
using (StreamReader sr = new StreamReader(path + "setting.txt"))
{
String line;
while ((line = sr.ReadLine()) != null)
{
if (line.StartsWith("color"))
color = line;
}
}
}
catch (Exception ex1) { MessageBox.Show("未找到文件setting.txt"); }
5.6将字符串拆分成数组,例如color=196,14,10
color = color.Substring(6);
String[] arr = color.Split(',');
//arr[0],arr[1],arr[2]
5.7设置label背景透明,即采用Form背景,以免快速变换时出现白色区域
this.labelMsg.BackColor = Color.Transparent;
设置label大小随文字大小变化
this.labelMsg.AutoSize = true;
this.labelMsg.Text = "";
设置label字体
this.labelMsg.Font = new System.Drawing.Font("LiSu", 16F, System.Drawing.FontStyle.Bold, System.Drawing.GraphicsUnit.Point, ((byte)(134)));
5.8设置窗体Form居中
this.Location = new System.Drawing.Point(Convert.ToInt32(screenWidth / 2) - Convert.ToInt32(this.Width / 2), Convert.ToInt32(screenHeight / 2) - Convert.ToInt32(this.Height / 2));
5.9操作Excel
首先引用
using Excel = Microsoft.Office.Interop.Excel;
定义变量
private Excel.Application excel;
private Excel.Workbook wb;//data.xls文件
private Excel.Worksheet renyuanWs, jiangxiangWs, rizhiWs, liwaiWs;
private String dataName = "";
private Excel.XlFileFormat version = Excel.XlFileFormat.xlExcel8;//2003版本
打开Excel进程
excel = new Excel.Application();
不显示Excel界面
excel.Visible = false;
不显示提示或警告
excel.DisplayAlerts = false;
打开data.xls
wb = excel.Workbooks.Add(path + "data.xls");
定义多个Excel区域,
Excel.Range range = null;
取得第一个Sheet
Excel.Worksheet s = (Excel.Worksheet)wb.Worksheets[1];
从第二行读取
for (int i = 2; i < s.UsedRange.Rows.Count + 1; i++)
{
取得第一个单元格或区域
range = s.get_Range(s.Cells[i, 1], s.Cells[i, 1]);
range.Value2即是range中的内容
if (range.Value2 !=null && range.Value2.ToString() != "")
MessageBox.Show(range.Value2.ToString());
else
break;
}
按顺序设置工作表的单元格内容
String now = DateTime.Now.ToLocalTime().ToString();
int r = rizhiWs.UsedRange.Rows.Count;
ws.Cells[r + 1, 1] = now;
保存Excel
wb.SaveAs(dataName, version, Type.Missing, Type.Missing, Type.Missing, Type.Missing, Excel.XlSaveAsAccessMode.xlExclusive, Type.Missing, Type.Missing, Type.Missing, Type.Missing, Type.Missing);
5.10取得随机数
new Random(new Random().Next(100)).Next(100);
5.11动态创建图片按钮
for (int i = 0; i < 10; i++)
{
System.Windows.Forms.PictureBox btn = new System.Windows.Forms.PictureBox();
每行显示6个按钮
if (i > 0 && i % 6 == 0)
level++;
btn.Location = new System.Drawing.Point(30 + (i - 6 * level) * 93, 30 + level * 38);
加上btn前缀便于识别
btn.Name = "btn" + i.ToString();
btn.Size = new System.Drawing.Size(90, 34);
设置图片按钮
btn.Image = Image.FromFile(path + "001.jpg");
btn.SizeMode = PictureBoxSizeMode.StretchImage;
btn.BackColor = Color.Transparent;
注册单击事件
btn.Click += new System.EventHandler(btn_Click);
btn.Cursor = Cursors.Hand;
this.Controls.Add(btn);
}
在btn_Click中取得传递过来的对象
private void btn_Click(object sender, EventArgs e)
{
PictureBox pic = (PictureBox)sender;
}
5.12填充字符串到一定长度,便于显示整齐
String str = "abc"
str = new String(' ', 10 - str.Length);
5.13窗体全屏
private void mFullScreen()
{
try
{
if (this.WindowState == FormWindowState.Maximized)
{
this.WindowState = FormWindowState.Normal;
this.FormBorderStyle = FormBorderStyle.Sizable;
}
else
{
this.FormBorderStyle = FormBorderStyle.None;
this.WindowState = FormWindowState.Maximized;
}
}
catch (Exception ex) { MessageBox.Show(ex.ToString()); }
}
5.14注册按键事件
比如按空格键开始或停止抽奖,这里我设置通过PageUp或是PageDown按键,这样就可通过翻页笔来控制
this.KeyDown += new System.Windows.Forms.KeyEventHandler(this.Form1_KeyDown);
private void Form1_KeyDown(object sender, KeyEventArgs e)
{
if (e.KeyValue == 32 || e.KeyValue == 33 || e.KeyValue == 34)
{
;//抽奖代码
}
}
5.15取得纳秒
long l = DateTime.Now.Ticks;
5.16显示或隐藏图片按钮
private void mShowCon(bool show)
{
try
{
foreach (Control con in this.Controls)
{
if (con.Name.StartsWith("btn") || con.Name.StartsWith("img"))
{
if (con.Visible == show)
break;
con.Visible = show;
}
}
}
catch (Exception ex) { MessageBox.Show(ex.ToString()); }
}
5.17打开第二个窗体
Form f = new Form2();
可以传递参数
Form f = new Form2("5");
f.Show();
在Form2中接收传递过来的值
public Form2(String str)
{
InitializeComponent();
Console.WrietLine(str);
}
1抽奖程序效果图
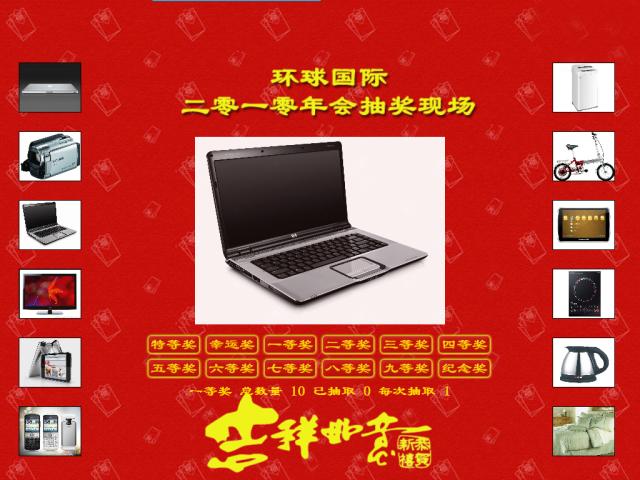
2抽奖程序功能设计
1、人员清单、奖项、抽奖记录等数据均在存储在Excel中,易于维护;2、抽奖结束时即可打印中奖人员清单及纪念奖清单,数据可排序、可筛选;3、在Excel中即可定义奖项数量、一次抽取数量、奖品图片等;4、可临时追加抽奖人数;5、每个奖项抽取完成后可显示当前奖项中奖人员清单;6、可自定义背景图片;7、限定每次按键间隔时间约5秒钟,防止误操作;8、限制抽奖数量不超过设定数量,并在屏幕上同步显示已抽取数量;9、奖品图片展示。
3 抽奖原理
人员名单来自人事,按工号升序排序,首次读入程序后,以随机方式打乱顺序,在此基础上,随机抽取。开始抽奖时,程序每隔0.01秒随机取得一个号,屏幕上每0.1秒显示一次当前随机取得的号,当停止抽奖时,程序随机抽取一个号作为中奖号码,并从人员清单中删除。
4运行环境
开发语言c#,开发工具VS2008,运行环境windows XP/VISTA、office2007、.netFrameWork3.5。
5部分代码讲解
5.1取得系统路径
String path = System.Windows.Forms.Application.StartupPath + "\\";
5.2取得电脑屏幕的宽度和高度
int screenWidth = System.Windows.Forms.Screen.GetBounds(this).Width;
int screenHeight = System.Windows.Forms.Screen.GetBounds(this).Height;
5.3设置背景图片,并设置图片自动伸缩
this.BackgroundImage = Image.FromFile(path + "bg.jpg");
this.BackgroundImageLayout = ImageLayout.Stretch;
5.4以RGB方式设置背景颜色
this.BackColor = Color.FromArgb(196, 14, 10);
5.5读取setting.txt中颜色的RGB值,setting.txt内容是color=196,14,10
String color = "";
try
{
using (StreamReader sr = new StreamReader(path + "setting.txt"))
{
String line;
while ((line = sr.ReadLine()) != null)
{
if (line.StartsWith("color"))
color = line;
}
}
}
catch (Exception ex1) { MessageBox.Show("未找到文件setting.txt"); }
5.6将字符串拆分成数组,例如color=196,14,10
color = color.Substring(6);
String[] arr = color.Split(',');
//arr[0],arr[1],arr[2]
5.7设置label背景透明,即采用Form背景,以免快速变换时出现白色区域
this.labelMsg.BackColor = Color.Transparent;
设置label大小随文字大小变化
this.labelMsg.AutoSize = true;
this.labelMsg.Text = "";
设置label字体
this.labelMsg.Font = new System.Drawing.Font("LiSu", 16F, System.Drawing.FontStyle.Bold, System.Drawing.GraphicsUnit.Point, ((byte)(134)));
5.8设置窗体Form居中
this.Location = new System.Drawing.Point(Convert.ToInt32(screenWidth / 2) - Convert.ToInt32(this.Width / 2), Convert.ToInt32(screenHeight / 2) - Convert.ToInt32(this.Height / 2));
5.9操作Excel
首先引用
using Excel = Microsoft.Office.Interop.Excel;
定义变量
private Excel.Application excel;
private Excel.Workbook wb;//data.xls文件
private Excel.Worksheet renyuanWs, jiangxiangWs, rizhiWs, liwaiWs;
private String dataName = "";
private Excel.XlFileFormat version = Excel.XlFileFormat.xlExcel8;//2003版本
打开Excel进程
excel = new Excel.Application();
不显示Excel界面
excel.Visible = false;
不显示提示或警告
excel.DisplayAlerts = false;
打开data.xls
wb = excel.Workbooks.Add(path + "data.xls");
定义多个Excel区域,
Excel.Range range = null;
取得第一个Sheet
Excel.Worksheet s = (Excel.Worksheet)wb.Worksheets[1];
从第二行读取
for (int i = 2; i < s.UsedRange.Rows.Count + 1; i++)
{
取得第一个单元格或区域
range = s.get_Range(s.Cells[i, 1], s.Cells[i, 1]);
range.Value2即是range中的内容
if (range.Value2 !=null && range.Value2.ToString() != "")
MessageBox.Show(range.Value2.ToString());
else
break;
}
按顺序设置工作表的单元格内容
String now = DateTime.Now.ToLocalTime().ToString();
int r = rizhiWs.UsedRange.Rows.Count;
ws.Cells[r + 1, 1] = now;
保存Excel
wb.SaveAs(dataName, version, Type.Missing, Type.Missing, Type.Missing, Type.Missing, Excel.XlSaveAsAccessMode.xlExclusive, Type.Missing, Type.Missing, Type.Missing, Type.Missing, Type.Missing);
5.10取得随机数
new Random(new Random().Next(100)).Next(100);
5.11动态创建图片按钮
for (int i = 0; i < 10; i++)
{
System.Windows.Forms.PictureBox btn = new System.Windows.Forms.PictureBox();
每行显示6个按钮
if (i > 0 && i % 6 == 0)
level++;
btn.Location = new System.Drawing.Point(30 + (i - 6 * level) * 93, 30 + level * 38);
加上btn前缀便于识别
btn.Name = "btn" + i.ToString();
btn.Size = new System.Drawing.Size(90, 34);
设置图片按钮
btn.Image = Image.FromFile(path + "001.jpg");
btn.SizeMode = PictureBoxSizeMode.StretchImage;
btn.BackColor = Color.Transparent;
注册单击事件
btn.Click += new System.EventHandler(btn_Click);
btn.Cursor = Cursors.Hand;
this.Controls.Add(btn);
}
在btn_Click中取得传递过来的对象
private void btn_Click(object sender, EventArgs e)
{
PictureBox pic = (PictureBox)sender;
}
5.12填充字符串到一定长度,便于显示整齐
String str = "abc"
str = new String(' ', 10 - str.Length);
5.13窗体全屏
private void mFullScreen()
{
try
{
if (this.WindowState == FormWindowState.Maximized)
{
this.WindowState = FormWindowState.Normal;
this.FormBorderStyle = FormBorderStyle.Sizable;
}
else
{
this.FormBorderStyle = FormBorderStyle.None;
this.WindowState = FormWindowState.Maximized;
}
}
catch (Exception ex) { MessageBox.Show(ex.ToString()); }
}
5.14注册按键事件
比如按空格键开始或停止抽奖,这里我设置通过PageUp或是PageDown按键,这样就可通过翻页笔来控制
this.KeyDown += new System.Windows.Forms.KeyEventHandler(this.Form1_KeyDown);
private void Form1_KeyDown(object sender, KeyEventArgs e)
{
if (e.KeyValue == 32 || e.KeyValue == 33 || e.KeyValue == 34)
{
;//抽奖代码
}
}
5.15取得纳秒
long l = DateTime.Now.Ticks;
5.16显示或隐藏图片按钮
private void mShowCon(bool show)
{
try
{
foreach (Control con in this.Controls)
{
if (con.Name.StartsWith("btn") || con.Name.StartsWith("img"))
{
if (con.Visible == show)
break;
con.Visible = show;
}
}
}
catch (Exception ex) { MessageBox.Show(ex.ToString()); }
}
5.17打开第二个窗体
Form f = new Form2();
可以传递参数
Form f = new Form2("5");
f.Show();
在Form2中接收传递过来的值
public Form2(String str)
{
InitializeComponent();
Console.WrietLine(str);
}