SSM环境中 Ajax 发送简单数组
第一种方法(不推荐)
jsp代码:
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<!doctype html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>首页</title>
<script type="text/javascript" src="jquery/jquery-2.1.1.min.js"></script>
<script>
$(function () {
$("#btn1").click(function () {
$.ajax({
"url": "send/array/one.html",//请求目标资源的地址
"type": "post",//请求方式
"data": {
"array": [5, 8, 10]
},//要发送的请求参数
"dataType": "text",//如何对待服务器返回的数据
"success": function (response) {//服务器端成功处理请求后调用的回调函数,response是响应体数据
alert(response);
},
"error": function (response) {//服务器端处理请求失败后调用的回调函数,response是响应体数据
alert(response);
}
})
})
})
</script>
</head>
<body>
<button id="btn1">Send [5,8,10] One</button>
</body>
</html>
Controller代码:
@ResponseBody
@RequestMapping("/send/array/one.html")
public String testReceiveArrayOne(@RequestParam("array[]") List<Integer> array){
for (Integer number: array) {
System.out.println("number="+number);
}
return "success";
}
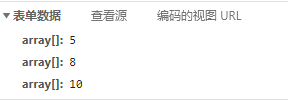
第二种方法(不推荐)需要用到实体类
pojo代码:
package com.lyc.pojo;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.NoArgsConstructor;
import java.util.List;
@Data
@AllArgsConstructor
@NoArgsConstructor
public class ParamData {
private List<Integer> array;
}
jsp代码:
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<!doctype html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>首页</title>
<script type="text/javascript" src="jquery/jquery-2.1.1.min.js"></script>
<script>
$(function () {
$("#btn2").click(function () {
$.ajax({
"url": "send/array/two.html",//请求目标资源的地址
"type": "post",//请求方式
"data": {
"array[0]": 5,
"array[1]": 8,
"array[2]": 12
},//要发送的请求参数
"dataType": "text",//如何对待服务器返回的数据
"success": function (response) {//服务器端成功处理请求后调用的回调函数,response是响应体数据
alert(response);
},
"error": function (response) {//服务器端处理请求失败后调用的回调函数,response是响应体数据
alert(response);
}
})
})
})
</script>
</head>
<body>
<button id="btn2">Send [5,8,10] Two</button>
</body>
</html>
Controller代码:
@ResponseBody
@RequestMapping("/send/array/two.html")
public String testReceiveArrayTwo(ParamData paramData){
List<Integer> array = paramData.getArray();
for (Integer number: array) {
System.out.println("number="+number);
}
return "success";
}

第三种方法(推荐)
jsp代码:
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<!doctype html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>首页</title>
<script type="text/javascript" src="jquery/jquery-2.1.1.min.js"></script>
<script>
$(function () {
$("#btn3").click(function () {
//准备好要发送到服务器端的数组
let array = [5, 8, 10];
console.log(array.length);
//将JSON数组转换为JSON字符串
let requestBody = JSON.stringify(array);
console.log(requestBody.length);
$.ajax({
"url": "send/array/three.html",//请求目标资源的地址
"type": "post",//请求方式
"data": requestBody,//请求体
"contentType": "application/json;charset=UTF-8",//请求体的内容类型,告诉服务器端本次请求的请求体是json数据
"dataType": "text",//如何对待服务器返回的数据
"success": function (response) {//服务器端成功处理请求后调用的回调函数,response是响应体数据
alert(response);
},
"error": function (response) {//服务器端处理请求失败后调用的回调函数,response是响应体数据
alert(response);
}
})
})
})
</script>
</head>
<body>
<button id="btn3">Send [5,8,10] Three</button>
</body>
</html>
Controller代码:
@ResponseBody
@RequestMapping("/send/array/three.html")
public String testReceiveArrayThree(@RequestBody List<Integer> array){
for (Integer number: array) {
logger.info("number="+number);
}
return "success";
}
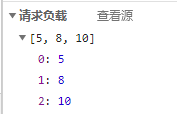