1、布局文件
a、主布局文件
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context="lpc.com.animation2demo.MainActivity">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="48dp">
<TextView
android:id="@+id/tv1"
android:text="苹果"
android:gravity="center"
android:layout_weight="1"
android:layout_width="match_parent"
android:layout_height="match_parent" />
<TextView
android:id="@+id/tv2"
android:text="香蕉"
android:gravity="center"
android:layout_weight="1"
android:layout_width="match_parent"
android:layout_height="match_parent" />
<TextView
android:text="梨"
android:id="@+id/tv3"
android:gravity="center"
android:layout_weight="1"
android:layout_width="match_parent"
android:layout_height="match_parent" />
</LinearLayout>
<ImageView
android:id="@+id/iv"
android:src="@mipmap/line"
android:scaleType="matrix"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
<android.support.v4.view.ViewPager
android:id="@+id/viewPager1"
android:layout_weight="1"
android:layout_width="match_parent"
android:flipInterval="30"
android:layout_gravity="center"
android:persistentDrawingCache="animation"
android:layout_height="0dp">
</android.support.v4.view.ViewPager>
</LinearLayout>

b、子布局
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical" android:layout_width="match_parent"
android:layout_height="match_parent">
<ImageView
android:background="@drawable/a1"
android:layout_width="match_parent"
android:layout_height="match_parent" />
</LinearLayout>
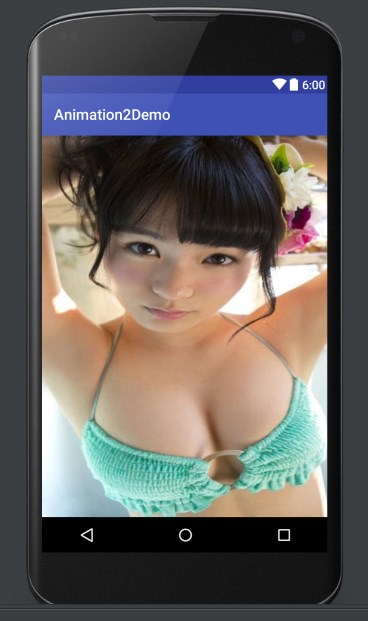
另外两子布局类似,只是图片和id不一样
2、主Java文件
package lpc.com.animation2demo;
import android.graphics.BitmapFactory;
import android.graphics.Matrix;
import android.os.Bundle;
import android.support.v4.view.ViewPager;
import android.support.v7.app.AppCompatActivity;
import android.util.DisplayMetrics;
import android.view.LayoutInflater;
import android.view.View;
import android.view.animation.Animation;
import android.view.animation.TranslateAnimation;
import android.widget.ImageView;
import android.widget.TextView;
import java.util.ArrayList;
public class MainActivity extends AppCompatActivity implements View.OnClickListener,ViewPager.OnPageChangeListener{
private TextView tv1; //文本对象1
private TextView tv2; //文本对象2
private TextView tv3; //文本对象3
private ImageView iv; //图片对象(用于移动条)
private ViewPager vp; //Viewpager对象
private ArrayList<View> viewArrayList; //创建一个View储存数组
private int offset = 0; //移动条图片的偏移量
private int currentIndex = 0; //当前页面
private int bmpWidth ; //图片宽度
private int one = 0; //移动条滑动一页的距离
private int two = 0; //滑动条移动两页的距离
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
initView();
}
private void initView() {
tv1 = (TextView) findViewById(R.id.tv1);
tv2 = (TextView) findViewById(R.id.tv2);
tv3 = (TextView) findViewById(R.id.tv3);
iv = (ImageView) findViewById(R.id.iv);
vp = (ViewPager) findViewById(R.id.viewPager1);
//下划线动画的相关设置:
bmpWidth = BitmapFactory.decodeResource(getResources(), R.mipmap.line).getWidth();// 获取图片宽度
DisplayMetrics dm = new DisplayMetrics();
getWindowManager().getDefaultDisplay().getMetrics(dm);
int screenW = dm.widthPixels;// 获取分辨率宽度
offset = (screenW / 3 - bmpWidth) / 2;// 计算偏移量
Matrix matrix = new Matrix();
matrix.postTranslate(offset, 0);
iv.setImageMatrix(matrix);// 设置动画初始位置
//移动的距离
one = offset * 2 + bmpWidth;// 移动一页的偏移量,比如1->2,或者2->3
two = one * 2;// 移动两页的偏移量,比如1直接跳3
//往ViewPager填充View,同时设置点击事件与页面切换事件
viewArrayList = new ArrayList<View>();
LayoutInflater inflater = getLayoutInflater();
viewArrayList.add(inflater.inflate(R.layout.view1,null,false));
viewArrayList.add(inflater.inflate(R.layout.view2,null,false));
viewArrayList.add(inflater.inflate(R.layout.view3,null,false));
vp.setAdapter(new MyAdapter(viewArrayList));
vp.setCurrentItem(0); //设置ViewPager当前页,从0开始算
tv1.setOnClickListener(this);
tv2.setOnClickListener(this);
tv3.setOnClickListener(this);
vp.addOnPageChangeListener(this);
}
@Override
public void onClick(View view) {
switch (view.getId()){
case R.id.tv1:
vp.setCurrentItem(0);
break;
case R.id.tv2:
vp.setCurrentItem(1);
break;
case R.id.tv3:
vp.setCurrentItem(2);
break;
}
}
@Override
public void onPageScrolled(int position, float positionOffset, int positionOffsetPixels) {
}
@Override
public void onPageSelected(int position) {
Animation animation = null;
switch (position){
case 0:
if (currentIndex == 1){
animation = new TranslateAnimation(one,0,0,0);
}else if (currentIndex == 2){
animation = new TranslateAnimation(two,0,0,0);
}
break;
case 1:
if (currentIndex == 0){
animation = new TranslateAnimation(offset,one,0,0);
}else if (currentIndex == 2){
animation = new TranslateAnimation(two,one,0,0);
}
break;
case 2:
if (currentIndex == 0){
animation = new TranslateAnimation(offset,two,0,0);
}else if (currentIndex == 1){
animation = new TranslateAnimation(one,two,0,0);
}
break;
}
currentIndex = position;
animation.setFillAfter(true);// true表示图片停在动画结束位置
animation.setDuration(300); //设置动画时间为300毫秒
iv.startAnimation(animation);//开始动画
}
@Override
public void onPageScrollStateChanged(int state) {
}
}
3、适配器文件
package lpc.com.animation2demo;
import android.support.v4.view.PagerAdapter;
import android.view.View;
import android.view.ViewGroup;
import java.util.ArrayList;
/**
* Created by Administrator on 2016/3/16.
* 适配器
*/
public class MyAdapter extends PagerAdapter {
//创建一个对象数组 存储View
private ArrayList<View> viewArrayList;
//空构造函数
public MyAdapter() {
}
//初始化对象数组
public MyAdapter(ArrayList<View> viewArrayList) {
this.viewArrayList = viewArrayList;
}
//获取对象数组的大小
@Override
public int getCount() {
return viewArrayList.size();
}
//将视图转化成对象
@Override
public boolean isViewFromObject(View view, Object object) {
return view == object;
}
//获取选定 对象数组并将其添加到viewpager中
@Override
public Object instantiateItem(ViewGroup container, int position) {
container.addView(viewArrayList.get(position));
return viewArrayList.get(position);
}
//移除选定的对象数组对象
@Override
public void destroyItem(ViewGroup container, int position, Object object) {
container.removeView(viewArrayList.get(position));
}
}
4、manifest文件
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="lpc.com.animation2demo">
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
5、实现效果
