二叉树创建
class TreeNode:
def __init__(self, val):
self.val = val
self.left = None
self.right = None
def createTree(l):
l = l[::-1]
def create(l):
a = l.pop()
if a == '#':
root = None
else:
root = TreeNode(a)
root.left = create(l)
root.right = create(l)
return root
return create(l)
验证二叉搜索树
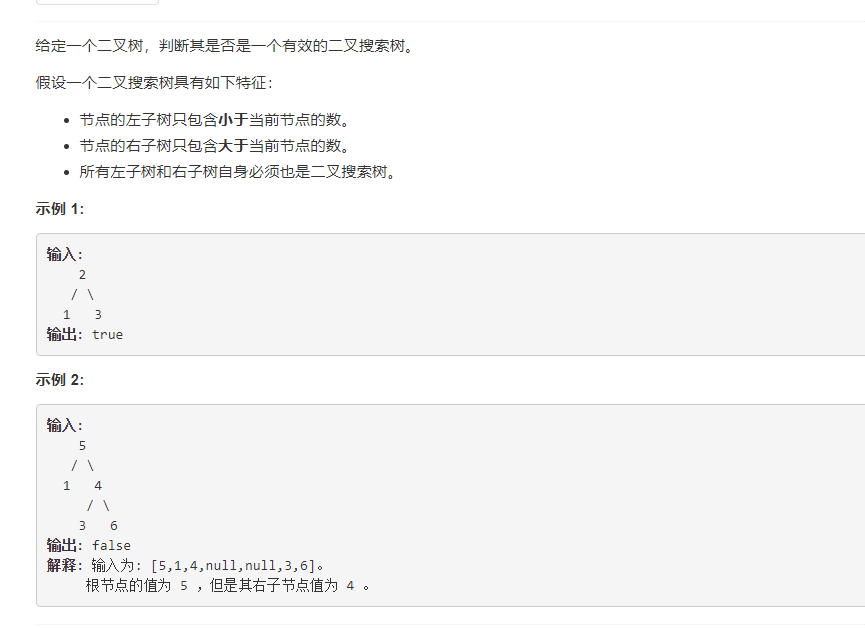
思路:刚开始以为直接判断每个节点大于左节点,小于右节点,就可以。测试不通过,原因是隔代有可能出现错误。
后面查阅了一番,大部分的解法思路如下:当前节点值就是左子树的最大值,右子树的最小值,所以只要传递下去即可。
# Definition for a binary tree node.
# class TreeNode:
# def __init__(self, x):
# self.val = x
# self.left = None
# self.right = None
class Solution:
def validBST(self, root, small, large):
if root is None:
return True
elif root.val <= small or root.val >= large:
return False
else:
return self.validBST(root.left, small, root.val) and self.validBST(root.right, root.val, large)
def isValidBST(self, root):
"""
:type root: TreeNode
:rtype: bool
"""
return self.validBST(root,-2**32,2**32)