效果
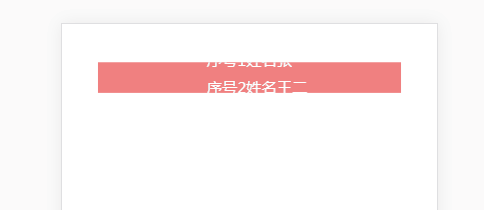
html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>纵向滚动</title>
<link rel="stylesheet" type="text/css" href="css/scrollVertical.css">
</head>
<script src="https://code.jquery.com/jquery-3.1.1.min.js"></script>
<script src="js/scroll.js"></script>
<body>
<div id="scrollFlash" class="scrollFlash">
<ul class="ulFlash"></ul>
</div>
<script type="text/javascript">
var list = [{
"id": "1",
"name": "张一",
},
{
"id": "2",
"name": "王二",
}
];
if (list.length > 0) {
$('#scrollFlash').show();
var j = 0;
while (j < list.length) {
var string = "<li>序号<span class='flashName t_center'>" +
list[j].id + "</span>姓名<span class='flashName t_center'>" +
list[j].name + "</span></li>";
$('.ulFlash').append(string);
j++;
}
var h = $('.ulFlash li:first-child').height() + 5;
if (list.length > 1) {
$("div#scrollFlash").myScroll({
speed: 40, // 数值越大,速度越慢
rowHeight: h // li的高度
});
}
}
</script>
</body>
</html>
scrollVertical.css
.t_center {
text-align: center;
}
#scrollFlash {
80%;
margin: 100px auto auto auto;
height: 60px;
background-color: lightcoral;
line-height: 60px;
padding: 5px;
font-size: 40px;
overflow: hidden;
color: #ffffff;
}
#scrollFlash ul li {
display: flex;
align-items: center;
justify-content: center;
margin-bottom: 2px;
}
.flashName {
max- 20%;
white-space: nowrap;
overflow: hidden;
text-overflow: ellipsis;
}
scroll.js
(function($){
$.fn.myScroll = function(options){
//默认配置
var defaults = {
speed:40, //滚动速度,值越大速度越慢
rowHeight:24 //每行的高度
};
var opts = $.extend({}, defaults, options),
intId = [];
function marquee(obj, step){
obj.find("ul").animate({
marginTop: '-=1'
},0,function(){
var s = Math.abs(parseInt($(this).css("margin-top")));
if(s >= step){
$(this).find("li").slice(0, 1).appendTo($(this));
$(this).css("margin-top", 0);
}
});
}
this.each(function(i){
var sh = opts["rowHeight"],speed = opts["speed"],
_this = $(this);
intId[i] = setInterval(function(){
if(_this.find("ul").height()<=_this.height()){
clearInterval(intId[i]);
}else{
marquee(_this, sh);
}
}, speed);
_this.hover(function(){
clearInterval(intId[i]);
},function(){
intId[i] = setInterval(function(){
if(_this.find("ul").height()<=_this.height()){
clearInterval(intId[i]);
}else{
marquee(_this, sh);
}
}, speed);
});
});
}
})(jQuery);