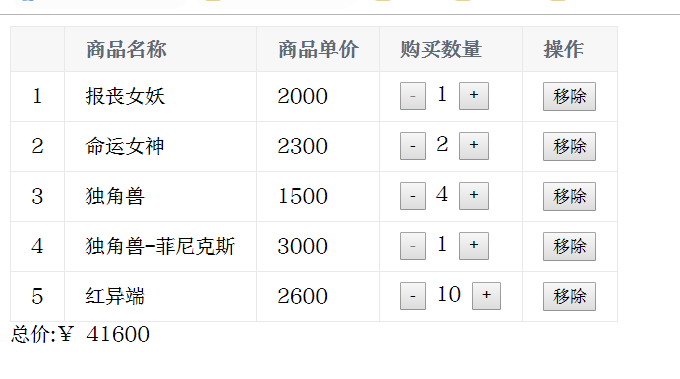
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
<script src="Vue.2.6.10.js"></script>
</head>
<style>
[v-cloak]{
display: none;
}
table{
border: 1px solid #e9e9e9;
border-collapse: collapse;
border-spacing: 0;
empty-cells: show;
}
th,td{
padding: 8px 16px;
border: 1px solid #e9e9e9;
text-align: left;
}
th{
background: #f7f7f7;
color: #5c6b77;
font-weight: 600;
white-space: nowrap;
}
</style>
<body>
<div id="app" v-cloak>
<template v-if = 'list.length'>
<!-- 只有当列表不为空时才显示购物车列表 -->
<table>
<thead>
<tr>
<th></th>
<th>商品名称</th>
<th>商品单价</th>
<th>购买数量</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<tr v-for = "(item,index) in list">
<td>{{ index + 1 }}</td>
<td>{{ item.name }}</td>
<td>{{ item.price }}</td>
<td>
<!-- 参数是商品在列表中的索引 -->
<!-- 按钮组是被包裹在带有v-for的tr中的 -->
<button
@click = 'handleReduce(index)'
:disabled = 'item.count === 1'
>-</button>
{{ item.count }}
<button @click = "handleAdd(index)">+</button>
</td>
<td>
<button @click = "handleRemove(index)">移除</button>
</td>
</tr>
</tbody>
</table>
<div>总价:¥ {{ totalPrice }}</div>
</template>
<div v-else>购物车为空</div>
</div>
</body>
<script>
var app = new Vue({
el:"#app",
data:{
list:[
{
id:001,
name:'报丧女妖',
price:2000,
count:1
},
{
id:002,
name:'命运女神',
price:2300,
count:2
},
{
id:003,
name:'独角兽',
price:1500,
count:4
},
{
id:004,
name:'独角兽-菲尼克斯',
price:3000,
count:1
},
{
id:005,
name:'红异端',
price:2600,
count:10
}
]
},
computed: {
totalPrice:function(){
var total = 0;
for(var i = 0;i<this.list.length;i++){
var item = this.list[i];
total += item.price*item.count;
}
return total.toString().replace(/B(?=(d{3}) + $)/g,',');
}
},
methods: {
handleReduce:function(index){
if(this.list[index].count === 1) return;
this.list[index].count--;
},
handleAdd:function(index){
this.list[index].count++;
},
handleRemove:function(index){
this.list.splice(index,1);//移除且无法在购物车界面恢复
}
},
});
</script>
</html>