Java程序设计期中考试
- 计算n!=123…*n.(20分)
- 输入10个整数,然后逆序输出这个10个整数。(20分)
- 计算S=1-1/(2^2)+1/(3^3)-1/(4^4)+…+(-1)^(n-1)/(n^n) (20分)
- 设计一个复数类Complex,用来实现加减运算,并在屏幕上输出当前复数。(30分)
第一题:
import java.util.Scanner;
public class test1 {
public static void main(String[]args){
int ss;
System.out.println("请输入一个数:");
Scanner console=new Scanner(System.in);
ss=console.nextInt();
int s=1;
for(int i=ss;i>=1;i--){
s=s*i;
}
System.out.println(s);
}
}
运行截图:

第二题:
import java.util.Scanner;
public class test {
public static void main(String[]args){
System.out.println("请输入10个数,以空格隔开:");
Scanner sc = new Scanner(System.in);
String str = sc.nextLine().toString();
String[] arr = str.split(" ");
int[]b = new int[arr.length];
for(int j = 0; j<b.length;j++)
{
b[j] = Integer.parseInt(arr[j]);
}
int n=b.length;
for (int i = 0; i < n; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (b[j] > b[j + 1]) {
int t=b[j];
b[j]=b[j+1];
b[j+1]=t;
}
}
}
for(int k=n-1;k>=0;k--){
System.out.print(b[k]+" ");
}
}
}
运行截图:

第三题:
import java.util.Scanner;
public class test {
public static void main(String[]args){
int n;
System.out.print("请输入一个数:");
Scanner console=new Scanner(System.in);
n=console.nextInt();
double s=0;
for(int i=1;i<=n;i++){
double a=0;
if(i%2==0){
a=-1/(Math.pow(i,i));
}
else{
a=1/(Math.pow大专栏 java期中测试="token punctuation">(i,i));
}
s=s+a;
}
System.out.println(s);
}
}
运行截图:

第四题:
import java.util.Scanner;
public class Complex {
double real;
double image;
Complex(){
Scanner input = new Scanner(System.in);
double real = input.nextDouble();
double image = input.nextDouble();
Complex(real,image);
}
private void Complex(double real, double image) {
// TODO Auto-generated method stub
this.real = real;
this.image = image;
}
Complex(double real,double image){
this.real = real;
this.image = image;
}
public double getReal() {
return real;
}
public void setReal(double real) {
this.real = real;
}
public double getImage() {
return image;
}
public void setImage(double image) {
this.image = image;
}
Complex add(Complex a){ // 相加
double real2 = a.getReal();
double image2 = a.getImage();
double newReal = real + real2;
double newImage = image + image2;
Complex result = new Complex(newReal,newImage);
return result;
}
Complex sub(Complex a){ // 相减
double real2 = a.getReal();
double image2 = a.getImage();
double newReal = real - real2;
double newImage = image - image2;
Complex result = new Complex(newReal,newImage);
return result;
}
public void print(){ // 输出
if(image > 0){
System.out.println(real + " + " + image + "i");
}else if(image < 0){
System.out.println(real + "" + image + "i");
}else{
System.out.println(real);
}
}
public static void main(String[] args) {
System.out.println("请用户输入第一个复数的实部和虚部:");
Complex data1 = new Complex();
System.out.println("请用户输入第二个复数的实部和虚部:");
Complex data2 = new Complex();
Complex result_add = data1.add(data2);
Complex result_sub = data1.sub(data2);
result_add.print();
result_sub.print();
}
}
运行截图:
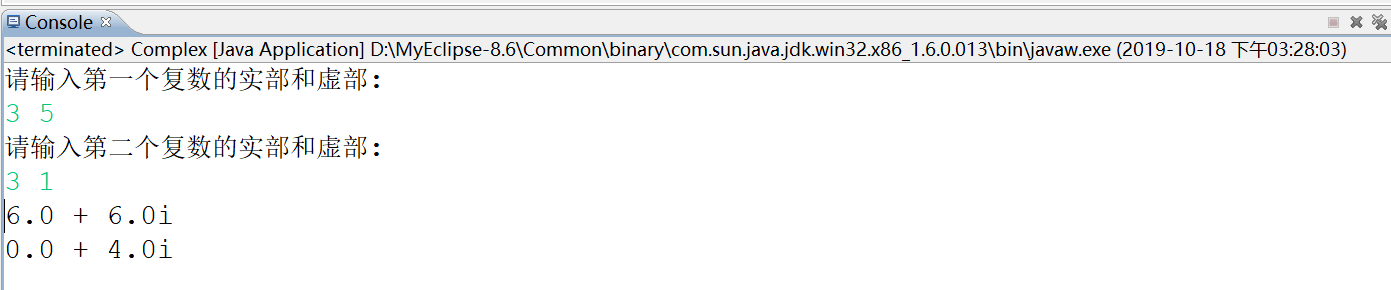