实现效果:
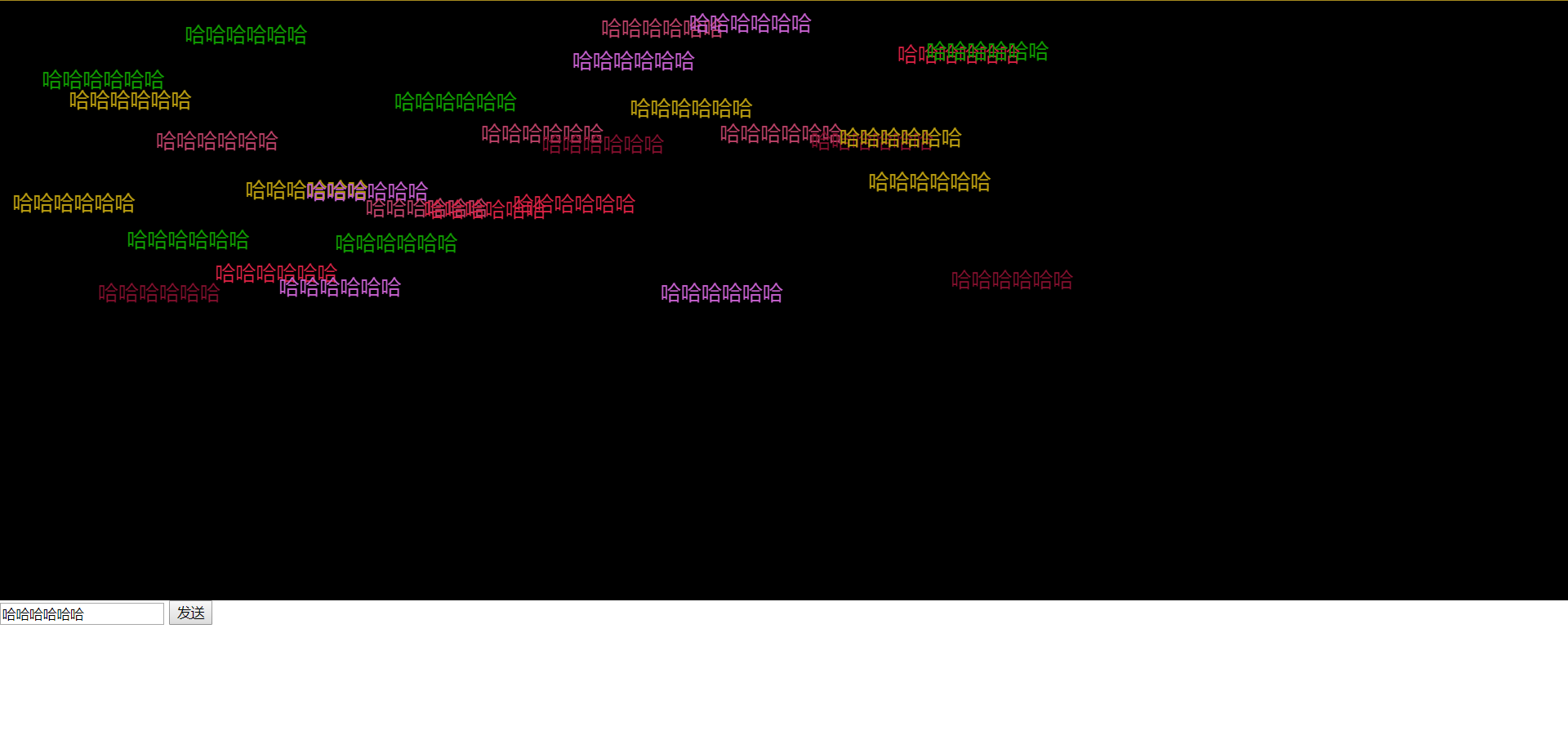
Html
1 <html> 2 3 <head> 4 <script type="text/javascript" src="js/jquery.min.js"></script> 5 <script type="text/javascript" src="js/index.js"></script> 6 </head> 7 <style> 8 body{ 9 margin: 0; 10 width:100%; 11 height:100%; 12 } 13 .box{ 14 width: 100%; 15 height:80%; 16 z-index: 50; 17 } 18 .show{ 19 top:0; 20 left:0; 21 width: 100%; 22 height:50%; 23 opacity:0.9;//改变值可以改变弹幕的透明度 24 z-index:100;//实际上就是两个div,一个在上显示,一个在下显示,z-index就是设置显示的优先级,值越大优先级越高 25 } 26 span{ 27 position: absolute; 28 font-size: 20px; 29 } 30 </style> 31 32 <body> 33 <div class="box"> 34 <div class="show"></div> 35 </div> 36 37 <div> 38 <input type="text" id="msg"> 39 <button id="send">发送</button> 40 </div> 41 </body> 42 </html> 43 44 JS: 45 $(function () { 46 $('#send').click(sendMsg); 47 }); 48 function sendMsg() { 49 var msg=$('#msg').val(); 50 var span="<span style='color:"+getColor()+";right:0;top:"+getTop()+"; '>"+msg+"</span>"; 51 span=$(span); 52 $('.show').append(span); 53 //动画效果 54 span.stop().animate({'right':getRight(span)},8000,'linear',function () { 55 $(this).remove(); 56 }); 57 58 } 59 //获取随机字体颜色 60 function getColor() { 61 var colorArr=["#cfaf12","#12af01","#981234","#adefsa","#db6be4","#f5264c","#d34a74"]; 62 var index=parseInt(colorArr.length*Math.random()); 63 return colorArr[index]; 64 } 65 //获取弹幕出现的随机高度位置 66 function getTop() { 67 var pageH=parseInt($('.show').height()); 68 var top=parseInt(pageH*Math.random()); 69 if (top>=pageH-20){ 70 top=top-20; 71 } 72 return top; 73 } 74 //获取动画的宽度最大值 75 function getRight(span) { 76 var width=span.width(); 77 var pageW=parseInt($('.show').width()); 78 var w=pageW-width; 79 return w; 80 }