Flyweight Pattern
享元模式, 将对象的相同属性, 以节省内存为目的,存储为一份公共对象, 所有对象共用此分对象。
The Flyweight Pattern is a pattern for greatly reducing memory requirements by not requiring that heavy-weight objects be created in large numbers when dealing with systems that contain many things that are mostly the same. If for instance, a document was modelled using a complex character class that knew about unicode, fonts, positioning, etc., then the memory requirements could be quite large for large documents if each physical character in the document required its own character class instance. Instead, characters themselves might be kept within Strings and we might have one character class (or a small number such as one character class for each font type) that knew the specifics of how to deal with characters.
In such circumstances, we call the state that is shared with many other things (e.g. the character type) instrinsic state. It is captured within the heavy-weight class. The state which distinguishes the physical character (maybe just its ASCII code or Unicode) is called its extrinsic state.
例子
class Boeing797 {
def wingspan = '80.8 m'
def capacity = 1000
def speed = '1046 km/h'
def range = '14400 km'
// ...
}
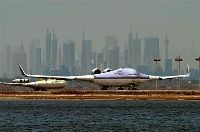
class Airbus380 {
def wingspan = '79.8 m'
def capacity = 555
def speed = '912 km/h'
def range = '10370 km'
// ...
}
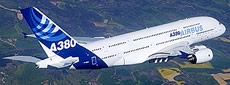
class FlyweightFactory { static instances = [797: new Boeing797(), 380: new Airbus380()] } class Aircraft { private type // instrinsic state private assetNumber // extrinsic state private bought // extrinsic state Aircraft(typeCode, assetNumber, bought) { type = FlyweightFactory.instances[typeCode] this.assetNumber = assetNumber this.bought = bought } def describe() { println """ Asset Number: $assetNumber Capacity: $type.capacity people Speed: $type.speed Range: $type.range Bought: $bought """ } } def fleet = [ new Aircraft(380, 1001, '10-May-2007'), new Aircraft(380, 1002, '10-Nov-2007'), new Aircraft(797, 1003, '10-May-2008'), new Aircraft(797, 1004, '10-Nov-2008') ] fleet.each { p -> p.describe() }