【先上链接:http://pan.baidu.com/s/1o62AHbc 】
多图杀猫
先用一组图看看实现的功能:
添加一个功能
假定现在要添加一个书本录入的功能,那么执行如下的操作:
1.添加Controller

public class BookController : DpfbMvcController { public ActionResult List(int size = 10, int index = 1) { throw new NotImplementedException(); } [Authorize] public ActionResult Add() { return View(); } [Authorize] [HttpPost] public ActionResult Add(BookAddViewModel model) { throw new NotImplementedException(); } }
2.定义Book模型,command,查询入口,仓储和实现commandHandler
//book

namespace SI.Cqrs.Models.AggreateRoots { public class Book : AggregateRoot { public string Name { get; set; } public decimal Price { get; set; } } }
//QueryEntry
public interface IBookQueryEntry : IQueryEntry<Book> { }
//Reponsitory
public interface IBookReponsitory:IBasicReponsitory<Book> { }
//handler,至于command,这里偷个懒,用泛型类解决

public class BookHandler:ICommandHandler<DpfbItemInsertCommand<Book>> { [Dependency] internal IBookReponsitory BookReponsitory { get; set; } void ICommandHandler<DpfbItemInsertCommand<Book>>.Execute(DpfbItemInsertCommand<Book> command) { BookReponsitory.Insert(command.AggregateRoot); } }
3.回过头来完成controller未实现的方法

public class BookController : DpfbMvcController { [Dependency] internal IBookQueryEntry BookQueryEntry { get; set; } public ActionResult List(int size = 10, int index = 1) { var pageInfo = new PageInfo(size, index); var result = BookQueryEntry.Page(i => i.Name, pageInfo); return View(result); } [Authorize] public ActionResult Add() { return View(); } [Authorize] [HttpPost] public ActionResult Add(BookAddViewModel model) { var book = new Book {Name = model.Name, Price = model.Price}; var command = new DpfbItemInsertCommand<Book> {AggregateRoot = book}; CommandBus.Send(command); return Redirect("List"); } }
4.实现Storage
//Reponsitory

public class BookReponsitory : SoftDeleteEntityFrameworkReponsitory<Book>, IBookReponsitory { }
//QueryEntry

public class BookQueryEntry : ReponsitoryBasedQueryEntry<Book>, IBookQueryEntry { public override IBasicReponsitory<Book> BasicReponsitory { get { return BookReponsitory; } } [Dependency] internal IBookReponsitory BookReponsitory { get; set; } }
5.同步数据库定义
//定义并添加一个Map

public class BookMap:TableMap<Book> { public BookMap() { /*为Name创建唯一索引*/ Property(i => i.Name).IsRequired() .HasColumnAnnotation(IndexAnnotation.AnnotationName, new IndexAttribute("IU_UserName", 1) {IsUnique = true}) .HasMaxLength(100); } } public class SocialInsuranceContext : DbContext { public SocialInsuranceContext() : base("name=SocialInsuranceContext") { } public DbSet<User> Users { get; set; } protected override void OnModelCreating(DbModelBuilder modelBuilder) { base.OnModelCreating(modelBuilder); modelBuilder.Configurations.Add(new UserMap()); modelBuilder.Configurations.Add(new BookMap()); } }
//更新数据库定义

PM> Add-Migration Initial_Database Scaffolding migration 'Initial_Database'. The Designer Code for this migration file includes a snapshot of your current Code First model. This snapshot is used to calculate the changes to your model when you scaffold the next migration. If you make additional changes to your model that you want to include in this migration, then you can re-scaffold it by running 'Add-Migration Initial_Database' again. PM> Update-Database Specify the '-Verbose' flag to view the SQL statements being applied to the target database. Applying explicit migrations: [201510300844286_Initial_Database]. Applying explicit migration: 201510300844286_Initial_Database. Running Seed method. PM>
结果测试
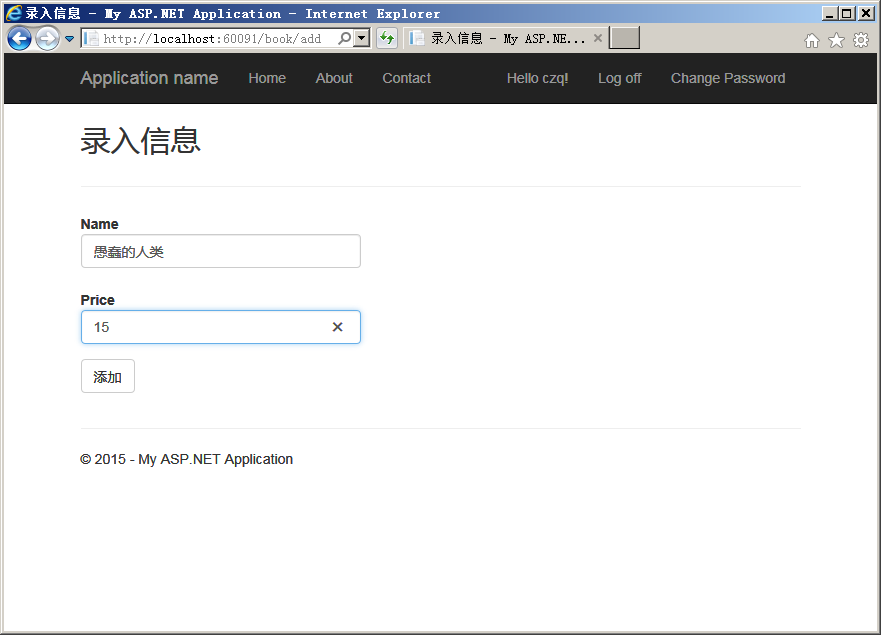
收工。