生成1-33中的6个随机数,无重复
------------------------------------------------------------------------
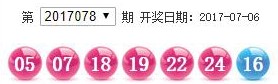
方法1.每生成一个随机数,便于前面的所有随机数进行比较,如果有重复,则舍去不要,重新选取。
但该方法十分费时,并且在数据量巨大的并且有一定限制的时候,会引发巨大问题。
例如要生成10000个随机数,范围是0-9999,且不能重复,那么最后几个随机数有可能需要相当长的时间才能筛选出来。
方法2. 下面我们从另外一个角度来思考,
假设我们已经由一个数组长度为10000的数组,里面分别存储了数据0-9999,我现在的做法是想办法让10000个数进行随机排列,
便得到了这样一个随机数列,如果我只要其中的100个数,那么从前面取出100个就好。这里利用algorithm.h里面的一个函数 random_shuffle ,来进行简单处理。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 | #include <algorithm> #include <iostream> #include <vector> using namespace std; void randperm( int Num) { vector< int > temp; for ( int i = 0; i < Num; ++i) { temp.push_back(i + 1); } random_shuffle(temp.begin(), temp.end()); //for (int i = 0; i < temp.size(); i++) for ( int i = 0; i < 6; i++) { cout << temp[i] << " " ; } } int main() { randperm(33); return 0; } |
方法3.使用链表(先sort在unique可以去重) 或者 set
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 | #include<iostream> using namespace std; #include <stdlib.h> #include <time.h> #include <list> #include <set> int act_rand( int a, int b) { int m = rand ()%(b-a+1)+a; cout << "m = " << m << endl; return m; } void genRandomList() { list< int > l; while ( l.size() < 6) { int randnum=act_rand(1,6); l.push_back(randnum); l.sort(); l.unique(); } list< int >::iterator it; //迭代器 for (it = l.begin(); it != l.end(); it++) { cout << *it << ' ' ; } } void genRandomSet() { set< int > s; while ( s.size() < 6) { int randnum=act_rand(1,33); s.insert(randnum); } set< int >::iterator it; //迭代器 for (it = s.begin(); it != s.end(); it++) { cout << *it << ' ' ; } } |
参考:
c++生成不重复的随机整数 - ~~(v_v)~~ - CSDN博客
http://blog.csdn.net/pythontojava/article/details/45132059
C++中随机数和不重复的随机数 - 朝拜SUNS - 博客园
http://www.cnblogs.com/salan668/p/3652532.html
(1)C++产生不重复的随机数_金庭波_新浪博客
http://blog.sina.com.cn/s/blog_4522f0b8010008r5.html