题目链接:http://acm.hdu.edu.cn/showproblem.php?pid=1016
Prime Ring Problem
Time Limit: 4000/2000 MS (Java/Others) Memory Limit: 65536/32768 K (Java/Others)
Total Submission(s): 18110 Accepted Submission(s): 8109
Problem Description
A
ring is compose of n circles as shown in diagram. Put natural number 1,
2, ..., n into each circle separately, and the sum of numbers in two
adjacent circles should be a prime.
Note: the number of first circle should always be 1.
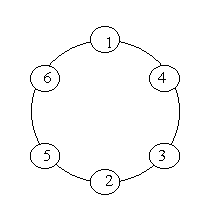
Note: the number of first circle should always be 1.
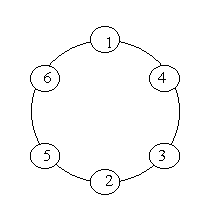
Input
n (0 < n < 20).
Output
The
output format is shown as sample below. Each row represents a series of
circle numbers in the ring beginning from 1 clockwisely and
anticlockwisely. The order of numbers must satisfy the above
requirements. Print solutions in lexicographical order.
You are to write a program that completes above process.
Print a blank line after each case.
You are to write a program that completes above process.
Print a blank line after each case.
Sample Input
6
8
Sample Output
Case 1:
1 4 3 2 5 6
1 6 5 2 3 4
Case 2:
1 2 3 8 5 6 7 4
1 2 5 8 3 4 7 6
1 4 7 6 5 8 3 2
1 6 7 4 3 8 5 2
Source
Recommend
JGShining
一开始想到用BFS搜索,但是标志设定是个大问题,想了很久还是没想出解决方法,经同学指点之后,我忽然明白,DFS可以解决这个问题,果断换上DFS,WA了几次,对比发现那case没大写。。。。囧
贴下龌龊的代码:
1 #include<stdio.h> 2 #include<string.h> 3 #include<math.h> 4 int vis[25], pr[104]; 5 int n; 6 void prime() 7 { 8 int m = 10, i, j; 9 memset(pr, 0, sizeof(pr)); 10 for(i = 2; i <= m; i++) 11 { 12 if(!pr[i]) 13 { 14 for(j = i * i; j <= 100; j += i) 15 pr[j] = 1; 16 } 17 } 18 } 19 void dfs(int g[], int cur) 20 { 21 int i, j; 22 for(i = 2; i <= n; i++) 23 { 24 if(vis[i] || pr[g[cur - 1] + i])continue; 25 g[cur] = i; 26 if(cur + 1 == n) 27 { 28 if(pr[g[cur] + 1])continue; 29 j = 0; 30 printf("%d", g[j]); 31 for(j = 1; j <= cur; j++) 32 printf(" %d", g[j]); 33 printf("\n"); 34 return ; 35 } 36 vis[i] = 1; 37 dfs(g, cur + 1); 38 vis[i] = 0; 39 } 40 } 41 int main() 42 { 43 int count = 0, g[30]; 44 prime(); 45 while(scanf("%d", &n) != EOF) 46 { 47 printf("Case %d:\n", ++count); 48 memset(g, 0, sizeof(g)); 49 memset(vis, 0, sizeof(vis)); 50 g[0] = 1; 51 dfs(g, 1); 52 printf("\n"); 53 } 54 return 0; 55 }