2020-03-31
二叉树的最小深度
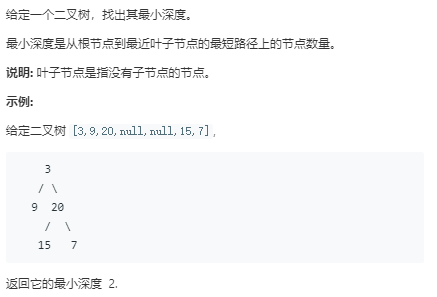
题解:
思路1: 递归 深度优先搜索
从最底层开始计算深度 往上依次+1 取两侧更小的那个
/** * Definition for a binary tree node. * function TreeNode(val) { * this.val = val; * this.left = this.right = null; * } */ /** * @param {TreeNode} root * @return {number} */ var minDepth = function (root) { if (root === null) return 0; // 若根节点为null则当前节点深度为0 if (root.left === null && root.right === null) return 1; // 若左右都为null且当前节点不为null 那么当前节点深度为1 if (root.left === null) return minDepth(root.right) + 1; // 若左边为null 那么节点的最小深度取决于right的最小深度+1 if (root.right === null) return minDepth(root.left) + 1; return Math.min(minDepth(root.left), minDepth(root.right)) + 1; // 若两边都不为null 递归 };