头文件部分
1 /*
2 版权信息:狼
3 文件名称:String.h
4 文件标识:
5 摘 要:对于上版本简易的String进行优化跟进。
6 改进
7 1.(将小块内存问题与大块分别对待)小内存块每个对象都有,当内存需求大于定义大小时利用动态分配
8 2.实现大块内存的写时拷贝功能,提高效率,优化空间利用
9 3.类似new[]实现机制:将动态内存块大小信息保存为隐藏“头”
10
11 当前版本:1.2
12 修 改 者:狼
13 完成日期:2015-12-12
14
15 取代版本:1.1
16 原 作 者:狼
17 完成日期:2015-12-11
18 */
19 #ifndef _STRING_H
20 #define _STRING_H
21 #include<iostream>
22 using namespace ::std;
23
24 namespace COW
25 {
26 class String
27 {
28 friend void Check_Interface(String &S);
29 friend ostream& operator << (ostream&os, String &Str);
30
31 public:
32 String(const char* str = "");
33 String(String& Str);
34 String& operator=(const String& Str);
35 ~String();
36
37 private:
38 void decrease();//减少引用计数。
39 void increase();//增加引用计数。
40
41 private:
42 enum{BufSize = 16}; //利用枚举进行数组初始化
43 char *_str; //内容块,“头”代替capacity
44 char _buff[BufSize]; //小内存块处理。
45 };
46 ostream& operator << (ostream&os, String &Str);
47 }
48 #endif
///////////////实现文件
#include"String.h"
#pragma warning (disable:4351 4996)
using namespace COW;
String::String(const char *Str)
:_str(NULL)
, _buff()
{
//采用动态分配
if (strlen(Str) >= BufSize)
{
_str = new char[strlen(Str) + 5];
*((int*)_str) = 1;
strcpy(_str + 4, Str);
}
else//栈内存储
{
strcpy(_buff, Str);
}
}
String::String(String& Str)
:_str(NULL)
, _buff()
{
// 1.如果是小块内存。浅拷贝
if (Str._str == NULL)
{
strcpy(_buff,Str._buff);
}
else
{
_str = Str._str;
this->increase();
}
}
String& String::operator=(const String& Str)
{
//都是动态 且指向一处
if (_str == Str._str&&_str != NULL)
return *this;
//两个都是小内存时
if (Str._str == NULL&&_str==NULL)
{
strcpy(_buff, Str._buff);
}
//如果*this 是小内存(不存在内存变更,不用处理buff),Str 动态。
else if (_str==NULL&&Str._str!=NULL)
{
_str = Str._str;
this->increase();
}
//*this 动态,Str小内存。减少*this._str计数。。.更改buff
else if(_str!=NULL&&Str._str==NULL)
{
this->decrease();
_str = NULL;
strcpy(_buff,Str._buff);
}
//两个都是动态分配 但不同
else
{
this->decrease();
_str = Str._str;
this->increase();
}
return *this;
}
void String::increase()
{
*(int*)_str += 1;
}
void String::decrease()
{
if (_str != NULL)
{
//////问题2,,每次decrease之后必须变更_str..为NULL或者指向新块
if ((*(int*)_str) - 1 != 0)
{
(*(int*)_str) -= 1;
}
else
{
delete[]_str;
_str = NULL;
}
}
}
String::~String()
{
this->decrease();
_str = NULL;
}
///////问题1...命名空间冲突时
ostream& COW::operator << (ostream&os, String &Str)
{
if (Str._str)
{
os << (Str._str + 4) << " "<< (*(int*)Str._str);
}
else
os << Str._buff;
return os;
}
//////friend 友员函数不能跨越命名空间访问私有成员????????????
//void Check_Interface(COW::String &S)
//{
// //char COW::String::*ch = S._str;
// char *ch = S._str;
// cout << endl;
//}
/////////////测试模块
#include"String.h"
using namespace COW;
void Test_COPY_EPU()
{
String S1("hellow world!");
String S2("hellow world");
String S3;
S3 = S2;
String S4;
S4 = S1;
//少对多,少对少
cout << "小内存赋值" << S3 << endl;
cout << "小内存赋值" << S4 << endl;
//多对多,多对少
String S5("change world");
S5 = S1;
cout << S5 << endl;
S5 = S2;
cout << S5 << endl;
//多多且等
String S6 = S1;
S6 = S1;
cout << S6 << endl;
}
void main()
{
Test_COPY_EPU();
}
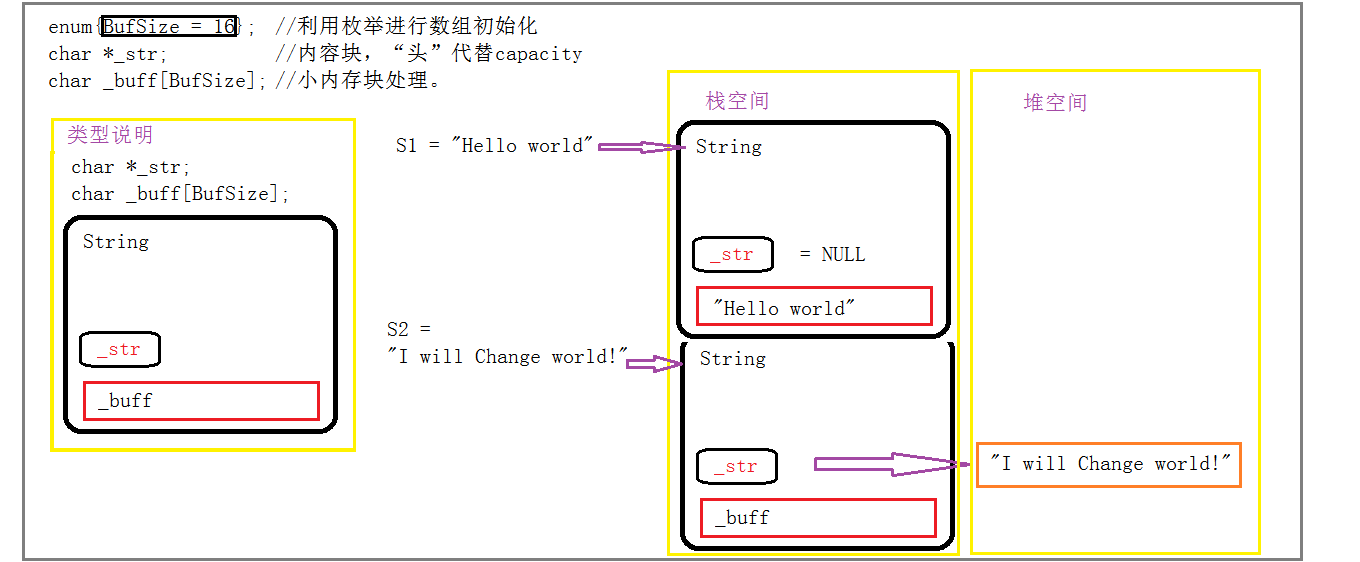