# -*- coding: utf-8 -*-
# @Time:
# @Auther: kongweixin
# @File:
"""
#举例:针对需求 比大小
def func(x,y):
if x>y:
return x
else:
return y
x=input("输入比较的第一个值:")
y=input("输入比较的第二个值:")
res=func(x,y)
print(res)
# 这种写法是比较纯粹的 (以基础水平来说 这种很简单写法是比较正常的)
# 但是接下来我们学一种新的简单方法进行写代码!!
"""
"""
#####################################################
########## #############
########## 1.0__三元表达式----总结 #############
########## #############
#####################################################
三元表达式
三元表达式是python为我们提供的一种简化代码的解决方案,
语法如下:
res = 条件成立时返回的值 if 条件 else 条件不成立时返回的值
针对上述场景用三元表达式
x=input("输入比较的第一个值:")
y=input("输入比较的第二个值:")
res =x if x>y else y
print(res)
# 应用举例:
def func():
if 1>3:
return 1
else:
return 3
print(func())
# 用三元表达式编写、
def func():
return 1 if 1>3 else 3
print(func())
# 没有什么难点, 敲一下就差不多了
"""
#####################################################
########## #############
########## 2.0__生成式----总结 #############
########## #############
#####################################################
"""
2.1 列表生成式
列表生成式是python为我们提供的一种简化代码的解决方案,
用来快速生成列表,语法如下:
[expression for item1 in iterable1 if condition1
for item2 in iterable2 if condition2
...
for itemN in iterableN if conditionN
]
# 把_dsb为结尾 提取出来
l = ['alex_dsb', 'lxx_dsb', 'wxx_dsb', "xxq_dsb", 'egon']
new_l=[]
for name in l:
if name.endswith("dsb"): #注意 是endswith 很多同学写成endwith
new_l.append(name)
print(new_l)
# 利用列表生成式
l = ['alex_dsb', 'lxx_dsb', 'wxx_dsb', "xxq_dsb", 'egon']
new_l=[name for name in l if name.endswith("dsb")]
print(new_l)
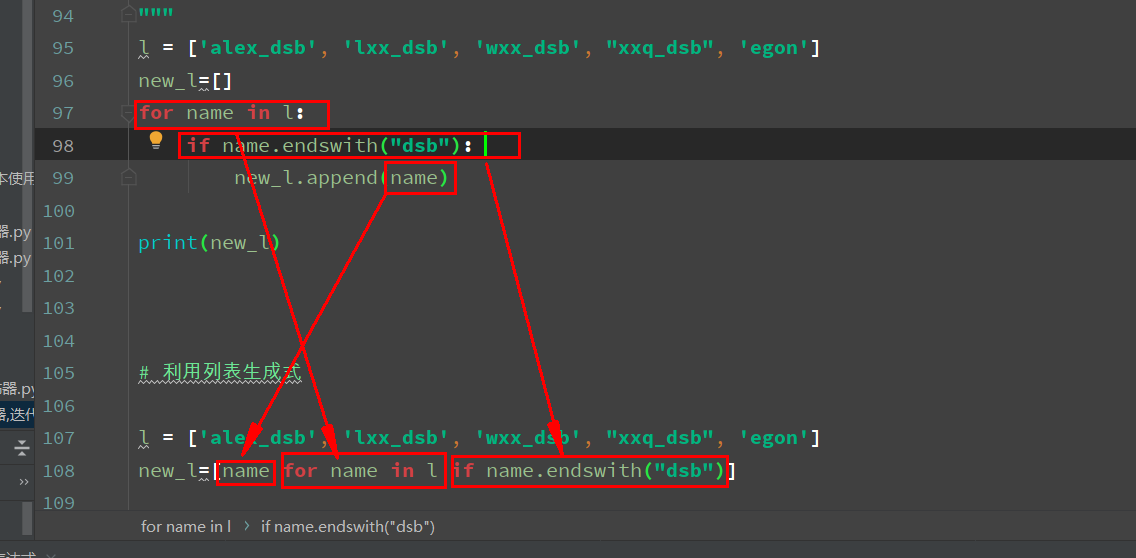
# 利用列表生成式
l = ['alex_dsb', 'lxx_dsb', 'wxx_dsb', "xxq_dsb", 'egon']
# 把_dsb为结尾 提取出来
new_l=[name for name in l if name.endswith("dsb")]
print(new_l)
# 把所有的小写字母转换成大写
new_l=[name.upper() for name in l ]
print(new_l)
# 把所有的名字去掉后缀_dsb
new_l=[name.strip("_dsb") for name in l ]
new_l = [name.replace("_dsb","")for name in l]
print(new_l)
2.2 字典生成式
keys=['name','age','gender']
dic ={key:123 for key in keys}
print(dic)
items=[('name','egon'),('age',18),('gender','male')]
res={k:v for k,v in items if k != 'gender'}
print(res)
# 补充:
# for k,v in items 是一种解压方法 举例说明:
# l,k=[1,2]
# print(l) #输出结果: 1
# print(k) #输出结果: 2
2.3、集合生成式
keys=['name','age','gender']
set1={key for key in keys}
print(set1,type(set1))
4、生成器表达式
创建一个生成器对象有两种方式,
一种是调用带yield关键字的函数,
另一种就是生成器表达式,与列表生成式的语法格式相同,只需要将[]换成(),
即:对比列表生成式返回的是一个列表,生成器表达式返回的是一个生成器对象
举例:1
g=(i for i in range(10) if i > 3)
!!!!!!!!!!!强调!!!!!!!!!!!!!!!
此刻g内部一个值也没有
print(g,type(g))
向值的话 需要
print(next(g))
print(next(g))
print(next(g))
print(next(g))
print(next(g))
print(next(g))
print(next(g))
举例:2
res1=[x*x for x in range(3)]
print(res1)
res2=(x*x for x in range(3))
print(res2)
print(next(res2))
print(next(res2))
print(next(res2))
print(next(res2)) # StopIteration
输出结果:
[0, 1, 4]
<generator object <genexpr> at 0x0000027DD6AC4748>
5.0文件进行生成器
如果我们要读取一个大文件的字节数,应该基于生成器表达式的方式完成
with open('笔记.txt', mode='rt', encoding='utf-8') as f:
# 方式一:将文件传到内存中让然后进行统计字符总数
res=0
for line in f:
res+=len(line)
print(res)
# 方式二:利用sum()的函数方式
# res=sum([1,2,3,4,5,6])
# print(res)
res=sum([len(line) for line in f])
print(res)
# 方式三 :效率最高
# res = sum((len(line) for line in f))
# 上述可以简写为如下形式
res = sum(len(line) for line in f)
print(res)
或者是 这里要注意encoding的字符编码 和mode的模式 t模式或者b模式是不一样的
with open('笔记.txt','rt', encoding='utf-8') as f:
nums=(len(line) for line in f)
total_size=sum(nums)
print(total_size)
"""