https://blog.csdn.net/shengmingqijiquan/article/details/52634640
一.ArrayList概述
- ArrayList 是一个数组队列,相当于动态数组。与Java中的数组相比,它的容量能动态增长。它继承于AbstractList,实现了List,RandomAccess[随机访问],Cloneable[可克隆], java.io.Serializable[序列化]这些接口。
- ArrayList 继承了AbstractList,实现了List。它是一个数组队列,提供了相关的添加、删除、修改、遍历等功能。
- ArrayList 实现了RandmoAccess接口,即提供了随机访问功能。RandmoAccess是java中用来被List实现,为List提供快速访问功能的。在ArrayList中,我们即可以通过元素的序号快速获取元素对象;这就是快速随机访问。稍后,我们会比较List的“快速随机访问”和“通过Iterator迭代器访问”的效率。
- ArrayList 实现了Cloneable接口,即覆盖了函数clone(),能被克隆。
- ArrayList 实现java.io.Serializable接口,这意味着ArrayList支持序列化,能通过序列化去传输。
- 和Vector不同,ArrayList中的操作不是线程安全的。所以,建议在单线程中才使用ArrayList,而在多线程中可以选择Vector或者CopyOnWriteArrayList。
二.ArrayList之API
1.ArrayList和Collection之间的关系
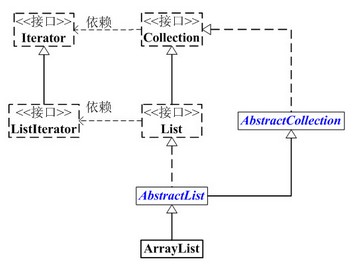
*实线代表直接继承的父类,虚线代表实现的接口;
2.ArrayList类的API
-
<span style="font-family:Microsoft YaHei;">// Collection中定义的API
-
boolean add(E object)//添加一个数组对象
-
boolean addAll(Collection<? extends E> collection)//添加一个包含Collection的对象
-
void clear()//清空
-
boolean contains(Object object)//包含
-
boolean containsAll(Collection<?> collection)
-
boolean equals(Object object)//判等
-
int hashCode()
-
boolean isEmpty()//判空
-
Iterator<E> iterator()
-
boolean remove(Object object)//删除
-
boolean removeAll(Collection<?> collection)
-
boolean retainAll(Collection<?> collection)
-
int size()
-
<T> T[] toArray(T[] array)
-
Object[] toArray()
-
// AbstractCollection中定义的API
-
void add(int location, E object)
-
boolean addAll(int location, Collection<? extends E> collection)
-
E get(int location)//获取某个元素值
-
int indexOf(Object object)
-
int lastIndexOf(Object object)
-
ListIterator<E> listIterator(int location)
-
ListIterator<E> listIterator()
-
E remove(int location)
-
E set(int location, E object)
-
List<E> subList(int start, int end)
-
// ArrayList新增的API
-
Object clone()//
-
void ensureCapacity(int minimumCapacity)//保证容量不小于元素个数
-
void trimToSize()
-
void removeRange(int fromIndex, int toIndex)
-
</span>
3.ArrayList的源码解析
-
<span style="font-family:Microsoft YaHei;">package java.util;
-
public class ArrayList<E> extends AbstractList<E>
-
implements List<E>, RandomAccess, Cloneable, java.io.Serializable
-
{
-
// 序列版本号
-
private static final long serialVersionUID = 8683452581122892189L;
-
// 保存ArrayList中数据的数组
-
private transient Object[] elementData;
-
// ArrayList中实际数据的数量
-
private int size;
-
// ArrayList带容量大小的构造函数。
-
public ArrayList(int initialCapacity) {
-
super();
-
if (initialCapacity < 0)
-
throw new IllegalArgumentException("Illegal Capacity: "+
-
initialCapacity);
-
// 新建一个数组
-
this.elementData = new Object[initialCapacity];
-
}
-
// ArrayList构造函数。默认容量是10。
-
public ArrayList() {
-
this(10);
-
}
-
// 创建一个包含collection的ArrayList
-
public ArrayList(Collection<? extends E> c) {
-
elementData = c.toArray();
-
size = elementData.length;
-
// c.toArray might (incorrectly) not return Object[] (see 6260652)
-
if (elementData.getClass() != Object[].class)
-
elementData = Arrays.copyOf(elementData, size, Object[].class);
-
}
-
// 将当前容量值设为 =实际元素个数
-
public void trimToSize() {
-
modCount++;
-
int oldCapacity = elementData.length;
-
if (size < oldCapacity) {
-
elementData = Arrays.copyOf(elementData, size);
-
}
-
}
-
// 确定ArrarList的容量。
-
// 若ArrayList的容量不足以容纳当前的全部元素,设置 新的容量=“(原始容量x3)/2 + 1”
-
public void ensureCapacity(int minCapacity) {
-
// 将“修改统计数”+1
-
modCount++;
-
int oldCapacity = elementData.length;
-
// 若当前容量不足以容纳当前的元素个数,设置 新的容量=“(原始容量x3)/2 + 1”
-
if (minCapacity > oldCapacity) {
-
Object oldData[] = elementData;
-
int newCapacity = (oldCapacity * 3)/2 + 1;
-
if (newCapacity < minCapacity)
-
newCapacity = minCapacity;
-
elementData = Arrays.copyOf(elementData, newCapacity);
-
}
-
}
-
// 添加元素e
-
public boolean add(E e) {
-
// 确定ArrayList的容量大小
-
ensureCapacity(size + 1); // Increments modCount!!
-
// 添加e到ArrayList中
-
elementData[size++] = e;
-
return true;
-
}
-
// 返回ArrayList的实际大小
-
public int size() {
-
return size;
-
}
-
// 返回ArrayList是否包含Object(o)
-
public boolean contains(Object o) {
-
return indexOf(o) >= 0;
-
}
-
// 返回ArrayList是否为空
-
public boolean isEmpty() {
-
return size == 0;
-
}
-
// 正向查找,返回元素的索引值
-
public int indexOf(Object o) {
-
if (o == null) {
-
for (int i = 0; i < size; i++)
-
if (elementData[i]==null)
-
return i;
-
} else {
-
for (int i = 0; i < size; i++)
-
if (o.equals(elementData[i]))
-
return i;
-
}
-
return -1;
-
}
-
// 反向查找,返回元素的索引值
-
public int lastIndexOf(Object o) {
-
if (o == null) {
-
for (int i = size-1; i >= 0; i--)
-
if (elementData[i]==null)
-
return i;
-
} else {
-
for (int i = size-1; i >= 0; i--)
-
if (o.equals(elementData[i]))
-
return i;
-
}
-
return -1;
-
}
-
// 反向查找(从数组末尾向开始查找),返回元素(o)的索引值
-
public int lastIndexOf(Object o) {
-
if (o == null) {
-
for (int i = size-1; i >= 0; i--)
-
if (elementData[i]==null)
-
return i;
-
} else {
-
for (int i = size-1; i >= 0; i--)
-
if (o.equals(elementData[i]))
-
return i;
-
}
-
return -1;
-
}
-
-
// 返回ArrayList的Object数组
-
public Object[] toArray() {
-
return Arrays.copyOf(elementData, size);
-
}
-
// 返回ArrayList的模板数组。所谓模板数组,即可以将T设为任意的数据类型
-
public <T> T[] toArray(T[] a) {
-
// 若数组a的大小 < ArrayList的元素个数;
-
// 则新建一个T[]数组,数组大小是“ArrayList的元素个数”,并将“ArrayList”全部拷贝到新数组中
-
if (a.length < size)
-
return (T[]) Arrays.copyOf(elementData, size, a.getClass());
-
// 若数组a的大小 >= ArrayList的元素个数;
-
// 则将ArrayList的全部元素都拷贝到数组a中。
-
System.arraycopy(elementData, 0, a, 0, size);
-
if (a.length > size)
-
a[size] = null;
-
return a;
-
}
-
// 获取index位置的元素值
-
public E get(int index) {
-
RangeCheck(index);
-
return (E) elementData[index];
-
}
-
// 设置index位置的值为element
-
public E set(int index, E element) {
-
RangeCheck(index);
-
E oldValue = (E) elementData[index];
-
elementData[index] = element;
-
return oldValue;
-
}
-
// 将e添加到ArrayList中
-
public boolean add(E e) {
-
ensureCapacity(size + 1); // Increments modCount!!
-
elementData[size++] = e;
-
return true;
-
}
-
// 将e添加到ArrayList的指定位置
-
public void add(int index, E element) {
-
if (index > size || index < 0)
-
throw new IndexOutOfBoundsException(
-
"Index: "+index+", Size: "+size);
-
ensureCapacity(size+1); // Increments modCount!!
-
System.arraycopy(elementData, index, elementData, index + 1,
-
size - index);
-
elementData[index] = element;
-
size++;
-
}
-
// 删除ArrayList指定位置的元素
-
public E remove(int index) {
-
RangeCheck(index);
-
modCount++;
-
E oldValue = (E) elementData[index];
-
int numMoved = size - index - 1;
-
if (numMoved > 0)
-
System.arraycopy(elementData, index+1, elementData, index,
-
numMoved);
-
elementData[--size] = null; // Let gc do its work
-
return oldValue;
-
}
-
// 删除ArrayList的指定元素
-
public boolean remove(Object o) {
-
if (o == null) {
-
for (int index = 0; index < size; index++)
-
if (elementData[index] == null) {
-
fastRemove(index);
-
return true;
-
}
-
} else {
-
for (int index = 0; index < size; index++)
-
if (o.equals(elementData[index])) {
-
fastRemove(index);
-
return true;
-
}
-
}
-
return false;
-
}
-
// 快速删除第index个元素
-
private void fastRemove(int index) {
-
modCount++;
-
int numMoved = size - index - 1;
-
// 从"index+1"开始,用后面的元素替换前面的元素。
-
if (numMoved > 0)
-
System.arraycopy(elementData, index+1, elementData, index,
-
numMoved);
-
// 将最后一个元素设为null
-
elementData[--size] = null; // Let gc do its work
-
}
-
// 删除元素
-
public boolean remove(Object o) {
-
if (o == null) {
-
for (int index = 0; index < size; index++)
-
if (elementData[index] == null) {
-
fastRemove(index);
-
return true;
-
}
-
} else {
-
// 便利ArrayList,找到“元素o”,则删除,并返回true。
-
for (int index = 0; index < size; index++)
-
if (o.equals(elementData[index])) {
-
fastRemove(index);
-
return true;
-
}
-
}
-
return false;
-
}
-
// 清空ArrayList,将全部的元素设为null
-
public void clear() {
-
modCount++;
-
for (int i = 0; i < size; i++)
-
elementData[i] = null;
-
size = 0;
-
}
-
// 将集合c追加到ArrayList中
-
public boolean addAll(Collection<? extends E> c) {
-
Object[] a = c.toArray();
-
int numNew = a.length;
-
ensureCapacity(size + numNew); // Increments modCount
-
System.arraycopy(a, 0, elementData, size, numNew);
-
size += numNew;
-
return numNew != 0;
-
}
-
// 从index位置开始,将集合c添加到ArrayList
-
public boolean addAll(int index, Collection<? extends E> c) {
-
if (index > size || index < 0)
-
throw new IndexOutOfBoundsException(
-
"Index: " + index + ", Size: " + size);
-
Object[] a = c.toArray();
-
int numNew = a.length;
-
ensureCapacity(size + numNew); // Increments modCount
-
int numMoved = size - index;
-
if (numMoved > 0)
-
System.arraycopy(elementData, index, elementData, index + numNew,
-
numMoved);
-
System.arraycopy(a, 0, elementData, index, numNew);
-
size += numNew;
-
return numNew != 0;
-
}
-
// 删除fromIndex到toIndex之间的全部元素。
-
protected void removeRange(int fromIndex, int toIndex) {
-
modCount++;
-
int numMoved = size - toIndex;
-
System.arraycopy(elementData, toIndex, elementData, fromIndex,
-
numMoved);
-
// Let gc do its work
-
int newSize = size - (toIndex-fromIndex);
-
while (size != newSize)
-
elementData[--size] = null;
-
}
-
private void RangeCheck(int index) {
-
if (index >= size)
-
throw new IndexOutOfBoundsException(
-
"Index: "+index+", Size: "+size);
-
}
-
// 克隆函数
-
public Object clone() {
-
try {
-
ArrayList<E> v = (ArrayList<E>) super.clone();
-
// 将当前ArrayList的全部元素拷贝到v中
-
v.elementData = Arrays.copyOf(elementData, size);
-
v.modCount = 0;
-
return v;
-
} catch (CloneNotSupportedException e) {
-
// this shouldn't happen, since we are Cloneable
-
throw new InternalError();
-
}
-
}
-
// java.io.Serializable的写入函数
-
// 将ArrayList的“容量,所有的元素值”都写入到输出流中
-
private void writeObject(java.io.ObjectOutputStream s)
-
throws java.io.IOException{
-
// Write out element count, and any hidden stuff
-
int expectedModCount = modCount;
-
s.defaultWriteObject();
-
// 写入“数组的容量”
-
s.writeInt(elementData.length);
-
// 写入“数组的每一个元素”
-
for (int i=0; i<size; i++)
-
s.writeObject(elementData[i]);
-
if (modCount != expectedModCount) {
-
throw new ConcurrentModificationException();
-
}
-
}
-
// java.io.Serializable的读取函数:根据写入方式读出
-
// 先将ArrayList的“容量”读出,然后将“所有的元素值”读出
-
private void readObject(java.io.ObjectInputStream s)
-
throws java.io.IOException, ClassNotFoundException {
-
// Read in size, and any hidden stuff
-
s.defaultReadObject();
-
// 从输入流中读取ArrayList的“容量”
-
int arrayLength = s.readInt();
-
Object[] a = elementData = new Object[arrayLength];
-
// 从输入流中将“所有的元素值”读出
-
for (int i=0; i<size; i++)
-
a[i] = s.readObject();
-
}
-
}
-
</span>
重点总结:
- ArrayList 实际上是通过一个数组去保存数据的。当我们构造ArrayList时;若使用默认构造函数,则ArrayList的默认容量大小是10。
- 当ArrayList容量不足以容纳全部元素时,ArrayList会重新设置容量:新的容量=“(原始容量x3)/2 + 1”。
- ArrayList的克隆函数,即是将全部元素克隆到一个数组中。
- ArrayList实现java.io.Serializable的方式。当写入到输出流时,先写入“容量”,再依次写入“每一个元素”;当读出输入流时,先读取“容量”,再依次读取“每一个元素”。
三.ArrayList的使用实例
1.ArrayList的添加
将字符a添加到list当中
-
<span style="font-family:Microsoft YaHei;">package com.ArrayList;
-
-
import java.util.ArrayList;
-
-
/**
-
* @author shengmingqijiquan
-
* @since 2016.09.23
-
*
-
* */
-
public class MyArrayList{
-
public static void main(String[] args) {
-
ArrayList<String> list = new ArrayList<String>();
-
list.add("a");//按照顺序依次添加。将a添加到list中
-
System.out.println(list+" ");
-
list.add(1,"b");//在第1个元素后面添加E,ArrayList中必须有足够多的数据,例如ArrayList中没有任何数据,这个时候使用arraylist.add(1, "E");就会出现java.lang.IndexOutOfBoundsException异常。
-
System.out.println(list+" ");
-
ArrayList<String> list1 = new ArrayList<String>();
-
list1.addAll(list);//将list中的全部数据添加到list1中
-
System.out.println(list1+" ");
-
list1.addAll(1,list);//将一个ArrayList中的所有数据添加到另外一个ArraList中的第1个元素之后
-
System.out.println(list1+" ");
-
-
}
-
-
}
-
</span>
运行结果:
2.ArrayList的删除
-
<span style="font-family:Microsoft YaHei;">package com.ArrayList;
-
-
import java.util.ArrayList;
-
/**
-
* @author shengmingqijiquan
-
* @since 2016.09.23
-
*
-
* */
-
public class ArrrayListRemove {
-
public static void main(String[] args) {
-
ArrayList<String> list = new ArrayList<String>();
-
list.add("a");
-
list.add("b");
-
list.add("c");
-
list.add("d");
-
list.add("e");
-
System.out.println(list+" ");
-
-
//1.按照内容删除单个数据.注意:对于int,String,char这样的原始类型数据是可以删除的,但是对于复杂对象,例如自己编写的User类、Person类对象,需要重写equals方法,负责remove方法无法匹配删除。
-
list.remove("a");//将list中的数据"d"删除
-
System.out.println("删除单个数据a:"+list);
-
-
//2.按照集合同时删除多个数据
-
ArrayList<String> list1 = new ArrayList<String>();
-
list1.add("a");
-
list1.add("b");
-
list.removeAll(list1);//按照list1的数据删除list
-
System.out.println("删除多个数据后,清空之前 "+list);
-
-
//3.清空ArrayList
-
list.clear();
-
System.out.println("清空之后 "+list);
-
-
}
-
}
-
</span>
3.ArrayList的修改
-
<span style="font-family:Microsoft YaHei;">package com.ArrayList;
-
-
import java.util.ArrayList;
-
/**
-
* @author shengmingqijiquan
-
* @date 2016.09.23
-
* */
-
public class ArrayListSet {
-
-
public static void main(String[] args) {
-
ArrayList<String> list = new ArrayList<>();
-
list.add("a");
-
list.add("b");
-
list.add("c");
-
list.add("d");
-
list.add("e");
-
System.out.println("修改前"+list);
-
list.set(1,"f");
-
list.set(2,"g");
-
System.out.println("修改后"+list);
-
}
-
-
}
-
</span>
4.ArrayList的查询
-
<span style="font-family:Microsoft YaHei;">package com.ArrayList;
-
-
import java.util.ArrayList;
-
/**
-
* @author shengmingqijiquan
-
* @date 2016.09.23
-
* */
-
public class ArrayListGet {
-
-
public static void main(String[] args) {
-
ArrayList<String> list = new ArrayList<>();
-
list.add("a");
-
list.add("b");
-
list.add("c");
-
list.add("d");
-
list.add("e");
-
System.out.println("初始化数组:"+list);
-
String ele = list.get(1);
-
System.out.println("查询到的元素ele:"+ele);
-
-
}
-
-
}
-
</span>
5.ArrayList的遍历
ArrayList支持3种遍历方式
- 第一种,通过迭代器遍历。即通过Iterator去遍历
-
<span style="font-family:Microsoft YaHei;">Integer value = null;
-
Iterator iter = list.iterator();
-
while (iter.hasNext()) {
-
value = (Integer)iter.next();
-
}
-
</span>
- 第二种,随机访问,通过索引值去遍历
-
<span style="font-family:Microsoft YaHei;">Integer value = null;
-
int size = list.size();
-
for (int i=0; i<size; i++) {
-
value = (Integer)list.get(i);
-
}
-
</span>
- 第三种,for循环遍历
-
<span style="font-family:Microsoft YaHei;">Integer value = null;
-
for (Integer integ:list) {
-
value = integ;
-
}</span>
比较这三种遍历方式的效率
-
<span style="font-family:Microsoft YaHei;">import java.util.*;
-
import java.util.concurrent.*;
-
/*
-
* @title ArrayList遍历方式和效率的测试程序。
-
*
-
* @author shengmingqijiquan
-
*/
-
public class ArrayListRandomAccessTest {
-
public static void main(String[] args) {
-
List list = new ArrayList();
-
for (int i=0; i<100000; i++)
-
list.add(i);
-
//isRandomAccessSupported(list);
-
iteratorThroughRandomAccess(list) ;
-
iteratorThroughIterator(list) ;
-
iteratorThroughFor2(list) ;
-
-
}
-
private static void isRandomAccessSupported(List list) {
-
if (list instanceof RandomAccess) {
-
System.out.println("RandomAccess implemented!");
-
} else {
-
System.out.println("RandomAccess not implemented!");
-
}
-
}
-
public static void iteratorThroughRandomAccess(List list) {
-
long startTime;
-
long endTime;
-
startTime = System.currentTimeMillis();
-
for (int i=0; i<list.size(); i++) {
-
list.get(i);
-
}
-
endTime = System.currentTimeMillis();
-
long interval = endTime - startTime;
-
System.out.println("iteratorThroughRandomAccess:" + interval+" ms");
-
}
-
public static void iteratorThroughIterator(List list) {
-
long startTime;
-
long endTime;
-
startTime = System.currentTimeMillis();
-
for(Iterator iter = list.iterator(); iter.hasNext(); ) {
-
iter.next();
-
}
-
endTime = System.currentTimeMillis();
-
long interval = endTime - startTime;
-
System.out.println("iteratorThroughIterator:" + interval+" ms");
-
}
-
public static void iteratorThroughFor2(List list) {
-
long startTime;
-
long endTime;
-
startTime = System.currentTimeMillis();
-
for(Object obj:list)
-
-
endTime = System.currentTimeMillis();
-
long interval = endTime - startTime;
-
System.out.println("iteratorThroughFor2:" + interval+" ms");
-
}
-
}
-
</span>
iteratorThroughRandomAccess:3 ms
iteratorThroughIterator:8 ms
iteratorThroughFor2:5 ms
由此可见,遍历ArrayList时,使用随机访问(即,通过索引序号访问)效率最高,而使用迭代器的效率最低!
6.ArrayList之toArray()补充
当我们调用ArrayList中的 toArray(),可能遇到过抛出“java.lang.ClassCastException”异常的情况。下面我们说说这是怎么回事。
ArrayList提供了2个toArray()函数:Object[] toArray()<T> T[] toArray(T[] contents)。调用 toArray() 函数会抛出“java.lang.ClassCastException”异常,但是调用 toArray(T[] contents) 能正常返回 T[]。
toArray() 会抛出异常是因为 toArray() 返回的是 Object[] 数组,将 Object[] 转换为其它类型(如如,将Object[]转换为的Integer[])则会抛出“java.lang.ClassCastException”异常,因为Java不支持向下转型。具体的可以参考前面ArrayList.java的源码介绍部分的toArray()。
解决该问题的办法是调用 <T> T[] toArray(T[] contents) , 而不是 Object[] toArray()。
ArrayList提供了2个toArray()函数:Object[] toArray()<T> T[] toArray(T[] contents)。调用 toArray() 函数会抛出“java.lang.ClassCastException”异常,但是调用 toArray(T[] contents) 能正常返回 T[]。
toArray() 会抛出异常是因为 toArray() 返回的是 Object[] 数组,将 Object[] 转换为其它类型(如如,将Object[]转换为的Integer[])则会抛出“java.lang.ClassCastException”异常,因为Java不支持向下转型。具体的可以参考前面ArrayList.java的源码介绍部分的toArray()。
解决该问题的办法是调用 <T> T[] toArray(T[] contents) , 而不是 Object[] toArray()。
调用 toArray(T[] contents) 返回T[]的可以通过以下几种方式实现。
-
<span style="font-family:Microsoft YaHei;">// toArray(T[] contents)调用方式一
-
public static Integer[] vectorToArray1(ArrayList<Integer> v) {
-
Integer[] newText = new Integer[v.size()];
-
v.toArray(newText);
-
return newText;
-
}
-
// toArray(T[] contents)调用方式二。最常用!
-
public static Integer[] vectorToArray2(ArrayList<Integer> v) {
-
Integer[] newText = (Integer[])v.toArray(new Integer[0]);
-
return newText;
-
}
-
// toArray(T[] contents)调用方式三
-
public static Integer[] vectorToArray3(ArrayList<Integer> v) {
-
Integer[] newText = new Integer[v.size()];
-
Integer[] newStrings = (Integer[])v.toArray(newText);
-
return newStrings;
-
}</span>
7.ArrayList综合实例
import java.util.*;
-
<span style="font-family:Microsoft YaHei;">/*
-
* @title ArrayList常用API的测试程序
-
* @author shengmingqijiquan
-
* @email 1127641712@qq.com
-
*/
-
public class ArrayListTest {
-
public static void main(String[] args) {
-
-
// 创建ArrayList
-
ArrayList list = new ArrayList();
-
// 将“”
-
list.add("1");
-
list.add("2");
-
list.add("3");
-
list.add("4");
-
// 将下面的元素添加到第1个位置
-
list.add(0, "5");
-
// 获取第1个元素
-
System.out.println("the first element is: "+ list.get(0));
-
// 删除“3”
-
list.remove("3");
-
// 获取ArrayList的大小
-
System.out.println("Arraylist size=: "+ list.size());
-
// 判断list中是否包含"3"
-
System.out.println("ArrayList contains 3 is: "+ list.contains(3));
-
// 设置第2个元素为10
-
list.set(1, "10");
-
// 通过Iterator遍历ArrayList
-
for(Iterator iter = list.iterator(); iter.hasNext(); ) {
-
System.out.println("next is: "+ iter.next());
-
}
-
// 将ArrayList转换为数组
-
String[] arr = (String[])list.toArray(new String[0]);
-
for (String str:arr)
-
System.out.println("str: "+ str);
-
// 清空ArrayList
-
list.clear();
-
// 判断ArrayList是否为空
-
System.out.println("ArrayList is empty: "+ list.isEmpty());
-
}
-
}
-
</span>