1. 在根模块下导入
import { ReactiveFormsModule } from '@angular/forms';
2.ts 文件
import { Component, OnInit } from '@angular/core';
import { Router } from '@angular/router';
import { FormGroup, FormBuilder ,FormArray } from '@angular/forms';
@Component({
selector: 'app-news',
templateUrl: './news.component.html',
styleUrls: ['./news.component.css']
})
export class NewsComponent {
constructor(private router: Router, private formBuilder: FormBuilder) { }
public fg:FormGroup =this.formBuilder.group(
{
// 创建数组对象
arrayList:this.formBuilder.array([])
}
);
/**
* 获取数组对象
*/
get arrayList()
{
return this.fg.get('arrayList') as FormArray;
}
/**
* 创建一行组件
*/
createRow(){
return this.formBuilder.group({
firstName:[''],
age:[''],
profession:[''],
});
}
/**
* 增加一行事件
*/
addBtn(){
this.arrayList.push(this.createRow());
}
/**
* 删除一行事件
*/
delBtn(i:number){
this.arrayList.removeAt(i);
}
}
3.HTML
<form [formGroup]="fg">
<table class="table table-bordered">
<tr><td>姓名</td><td>年龄</td><td>职业</td><td></td></tr>
<ng-container formArrayName='arrayList'>
<ng-container *ngFor="let row of arrayList.controls;let i =index">
<tr>
<ng-container [formGroup]="row">
<td>
<input type="text" class='form-control' formControlName="firstName">
</td>
<td>
<input type="text"class='form-control' formControlName="age">
</td>
<td>
<input type="text" class='form-control' formControlName="profession">
</td>
<td>
<button class='form-control' (click)="delBtn(i)">删除</button>
</td>
</ng-container>
</tr>
</ng-container>
</ng-container>
</table>
</form>
<button (click)="addBtn()">添加</button>
4.引入bootstrap
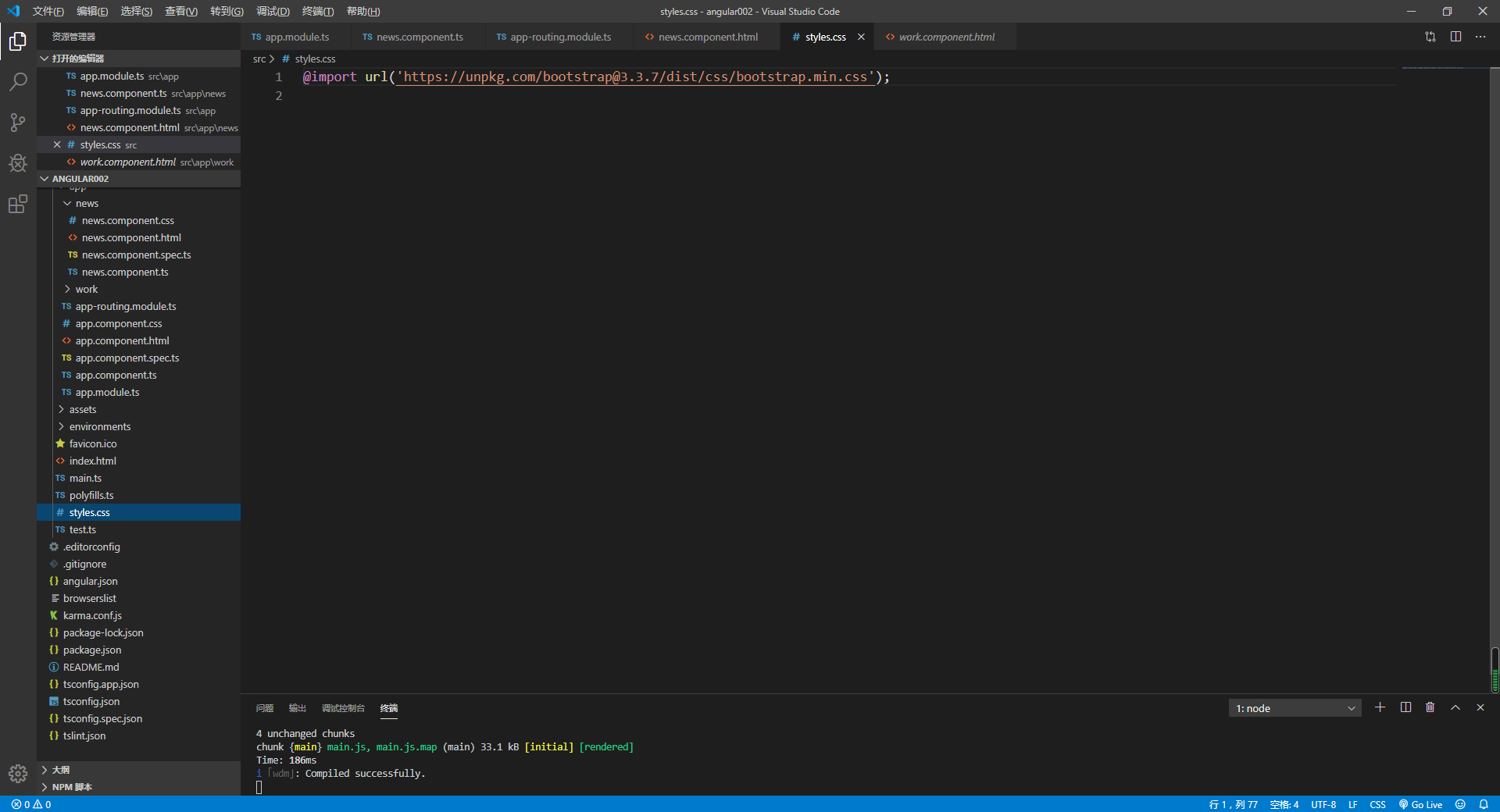