Part I
Introducing Android
Chapter 2 Key Concepts
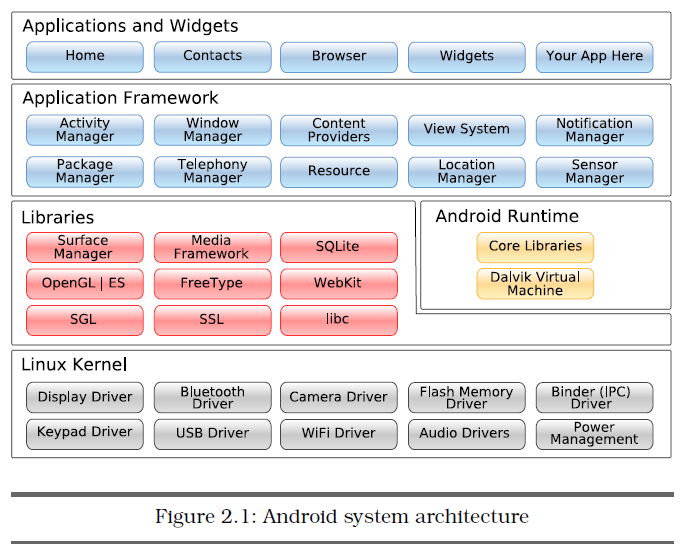
2.1 The Big Picture
◆. Linux Kernel
Internally, Android uses Linux for its memory management, process
management, networking, and other operating system services. The
Android phone user will never see Linux, and your programs will not make Linux calls directly. As a developer, though, you’ll need to be
aware it’s there. ◆. Native Libraries
The next layer above the kernel contains the Android native libraries.
These shared libraries are all written in C or C++, compiled for the
particular hardware architecture used by the phone, and preinstalled
by the phone vendor. Some of the most important native libraries include the following:
• Surface Manager: Android uses a compositing window manager
similar to Vista or Compiz, but it’s much simpler. Instead of draw- ing directly to the screen buffer, your drawing commands go into
off-screen bitmaps that are then combined with other bitmaps to
form the display the user sees. This lets the system create all
sorts of interesting effects such as see-through(透明的) windows and fancy(花俏的)
transitions. • 2D and 3D graphics: Two- and three-dimensional elements can be
combined in a single user interface with Android. The library will
use 3D hardware if the device has it or a fast software renderer(渲染) if
it doesn’t. • Media codecs(编解码器): Android can play video and record and play back
audio in a variety of formats including AAC, AVC (H.264), H.263,
MP3, and MPEG-4. • SQL database: Android includes the lightweight SQLite database
engine,the same database used in Firefox and the Apple iPhone.3
You can use this for persistent storage in your application. • Browser engine: For the fast display of HTML content, Android
uses the WebKit library. This is the same engine used in the
Google Chrome browser, Apple’s Safari browser, the Apple iPhone,
and Nokia’s S60 platform. These libraries are not applications that stand by themselves. They
exist only to be called by higher-level programs. ◆. Android Runtime
Also sitting on top of the kernel is the Android runtime, including the
Dalvik virtual machine and the core Java libraries. ◆. Application Framework
Sitting above the native libraries and runtime, you’ll find the Application
Framework layer. This layer provides the high-level building blocks
you will use to create your applications. The framework comes preinstalled
with Android, but you can also extend it with your own components
as needed. The most important parts of the framework are as follows:
• Activity Manager: This controls the life cycle of applications
and maintains a common “backstack” for user navigation.
• Content providers: These objects encapsulate data that needs to be
shared between applications, such as contacts. • Resource manager: Resources are anything that goes with (伴随)your
program that is not code. • Location manager: An Android phone always knows where it is.
• Notification manager: Events such as arriving messages, appointments,
proximity(接近的) alerts, alien invasions, and more can be presented
in an unobtrusive(不唐突的,不冒昧的) fashion to the user. ◆. Applications and Widgets
Applications are programs that can take over the whole screen and
interact with the user. On the other hand, widgets (which are sometimes
called gadgets), operate only in a small rectangle of the Home
screen application. 2.2 It’s Alive!
In Android, there is one foreground application, which typically takes
over the whole display except for the status line. When the user turns
on their phone, the first application they see is the Home application When the user runs an application, Android starts it and brings it to the
foreground. From that application, the user might invoke another application,
or another screen in the same application, and then another and
another. All these programs and screens are recorded on the application
stack by the system’s Activity Manager. At any time, the user can
press the Back button to return to the previous screen on the stack.
From the user’s point of view, it works a lot like the history in a web
browser. Pressing Back returns them to the previous page. ◆. Process != Application
Internally, each user interface screen is represented by an Activity class .
Each activity has its own life
cycle. An application is one or more activities plus a Linux process to
contain them. That sounds pretty straightforward, doesn’t it? But don’t
get comfortable yet; I’m about to throw you a curve(曲线) ball. In Android, an application can be “alive” even if its process has been
killed. Put another way(= in other words), the activity life cycle is not tied to the process
life cycle. Processes are just disposable(任意处理的) containers for activities. This is
probably different from every other system you’re familiar with, so let’s
take a closer look before moving on. ◆. Life Cycles of the Rich and Famous
During its lifetime, each activity of an Android program can be in one
of several states, 
• onCreate(Bundle): This is called when the activity first starts up.
You can use it to perform one-time initialization such as creating the user interface. onCreate( ) takes one parameter that is either
null or some state information previously saved by the onSaveInstanceState(
) method. • onStart( ): This indicates the activity is about to be displayed to the
user. • onResume( ): This is called when your activity can start interacting
with the user. This is a good place to start animations and music. • onPause( ): This runs when the activity is about to go into the background,
usually because another activity has been launched in
front of it. This is where you should save your program’s persistent
state, such as a database record being edited. • onStop( ): This is called when your activity is no longer visible to
the user and it won’t be needed for a while. If memory is tight,
onStop( ) may never be called (the system may simply terminate
your process). • onRestart( ): If this method is called, it indicates your activity is
being redisplayed to the user from a stopped state. • onDestroy( ): This is called right before your activity is destroyed. If
memory is tight, onDestroy( ) may never be called (the system may
simply terminate your process). • onSaveInstanceState(Bundle): Android will call this method to allow
the activity to save per-instance state, such as a cursor position
within a text field. Usually you won’t need to override it because
the default implementation saves the state for all your user interface
controls automatically. • onRestoreInstanceState(Bundle): This is called when the activity is
being reinitialized from a state previously saved by the onSave-
InstanceState( ) method. The default implementation restores the
state of your user interface. Activities that are not running in the foreground may be stopped, or
the Linux process that houses them may be killed at any time in order
to make room for new activities. This will be a common occurrence,
so it’s important that your application be designed from the beginning
with this in mind. In some cases, the onPause( ) method may be the last
method called in your activity, so that’s where you should save any data
you want to keep around for next time. 2.3 Building Blocks(积木)
A few objects are defined in the Android SDK that every developer needs
to be familiar with. The most important ones are activities, intents,
services, and content providers. You’ll see several examples of them in
the rest of the book, so I’d like to briefly introduce them now. ◆.Activities
each activity is responsible for
saving its own state so that it can be restored later as part of the
application life cycle. ◆.Intents
An intent is a mechanism for describing a specific action, such as “pick
a photo,” “phone home,” or “open the pod bay doors.” In Android, just
about everything goes through intents, so you have plenty of opportunities
to replace or reuse components. ◆.Services
A service is a task that runs in the background without the user’s direct
interaction, similar to a Unix daemon. A service is a task that runs in the background without the user’s direct
interaction, similar to a Unix daemon. For example, consider a music
player. The music may be started by an activity, but you want it to keep
playing even when the user has moved on to a different program. So, the
code that does the actual playing should be in a service. Later, another
activity may bind to that service and tell it to switch tracks or stop playing.
Android comes with many services built in, along with convenient
APIs to access them. ◆.Content Providers
A content provider is a set of data wrapped up in a custom API to read
and write it. This is the best way to share global data between applications.
For example, Google provides a content provider for contacts.
All the information there—names, addresses, phone numbers, and so
forth—can be shared by any application that wants to use it. 2.4 Using Resources
A resource is a localized text string, bitmap, or other small piece of
noncode information that your program needs. At build time all your
resources get compiled into your application. This is useful for internationalization
and for supporting multiple device types 2.5 Safe and Secure
As mentioned earlier, every application runs in its own Linux process.
The hardware forbids one process from accessing another process’s memory. Furthermore, every application is assigned a specific user ID.
Any files it creates cannot be read or written by other applications. In addition, access to certain critical operations are restricted, and you
must specifically ask for permission to use them in a file named Android-
Manifest.xml. When the application is installed, the Package Manager
either grants or doesn’t grant the permissions based on certificates
and, if necessary, user prompts. Here are some of the most common
permissions you will need: • INTERNET: Access the Internet.
• READ_CONTACTS: Read (but don’t write) the user’s contacts data.
• WRITE_CONTACTS: Write (but don’t read) the user’s contacts data.
• RECEIVE_SMS: Monitor incoming SMS (text) messages.
• ACCESS_COARSE_LOCATION: Use a coarse(粗糙的) location provider such as
cell towers or wifi.
• ACCESS_FINE_LOCATION: Use a more accurate location provider such
as GPS. Android can even restrict access to entire parts of the system. Using
XML tags in AndroidManifest.xml, you can restrict who can start an activity,
start or bind to a service, broadcast intents to a receiver, or access
the data in a content provider. This kind of control is beyond the scope
of this book, but if you want to learn more, read the online help for the
Android security model. (android apps 的SOP:
1. 现在res中创建界面:layout/main.xml--主界面,layout-land/main.xml 横屏时候的主界面)
2. 创建对应的Activity,并注册layout
3. 在AndroidManifest.xml中注册相关的Activity和权限。