Radar Installation
Time Limit: 1000MS | Memory Limit: 10000K | |
Total Submissions: 64472 | Accepted: 14497 |
Description
Assume the coasting is an infinite straight line. Land is in one side of coasting, sea in the other. Each small island is a point locating in the sea side. And any radar installation, locating on the coasting, can only cover d
distance, so an island in the sea can be covered by a radius installation, if the distance between them is at most d.
We use Cartesian coordinate system, defining the coasting is the x-axis. The sea side is above x-axis, and the land side below. Given the position of each island in the sea, and given the distance of the coverage of the radar installation, your task is to write a program to find the minimal number of radar installations to cover all the islands. Note that the position of an island is represented by its x-y coordinates.
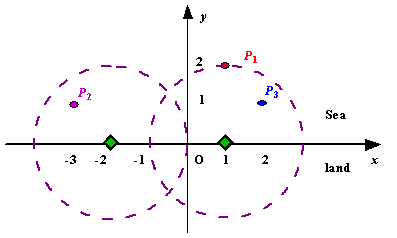
Figure A Sample Input of Radar Installations
We use Cartesian coordinate system, defining the coasting is the x-axis. The sea side is above x-axis, and the land side below. Given the position of each island in the sea, and given the distance of the coverage of the radar installation, your task is to write a program to find the minimal number of radar installations to cover all the islands. Note that the position of an island is represented by its x-y coordinates.
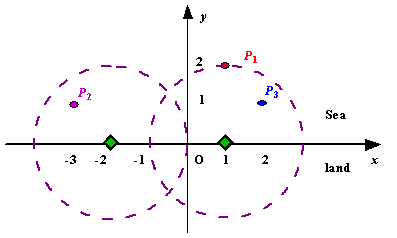
Figure A Sample Input of Radar Installations
Input
The input consists of several test cases. The first line of each case contains two integers n (1<=n<=1000) and d, where n is the number of islands in the sea and d is the distance of coverage of the radar installation. This is
followed by n lines each containing two integers representing the coordinate of the position of each island. Then a blank line follows to separate the cases.
The input is terminated by a line containing pair of zeros
The input is terminated by a line containing pair of zeros
Output
For each test case output one line consisting of the test case number followed by the minimal number of radar installations needed. "-1" installation means no solution for that case.
Sample Input
3 2 1 2 -3 1 2 1 1 2 0 2 0 0
Sample Output
Case 1: 2 Case 2: 1
Source
#include<iostream> #include<cstdio> #include<cstring> #include<cmath> #include<algorithm> using namespace std; struct node { double L,R; } p[1005]; int cmp(node p1,node p2) { return p1.L<p2.L; } int main() { int n,d,num=0; while(cin>>n>>d) { num++; if(n==0&&d==0) break; int flag=1; for(int i=0; i<n; i++) { int u,v; cin>>u>>v; if(flag==0) continue; if(d<v) //注意半径能够取负的,所以不能用d*d<v*v比較 { flag=0; } else { p[i].L=(double)u-sqrt((double)(d*d-v*v)); p[i].R=(double)u+sqrt((double)(d*d-v*v)); } } if(flag==0) { printf("Case %d: -1 ",num); continue; } sort(p,p+n,cmp); double x=p[0].R; int sum=1; for(int i=1; i<n; i++) { if(p[i].R<x) { x=p[i].R; } else if(x<p[i].L) { sum++; x=p[i].R; } } printf("Case %d: %d ",num,sum); } } /*把每一个岛屿来当做雷达的圆心。半径为d,做圆。与x轴会产生两个焦点L和R,这就是一个区间; 首先就是要把全部的区间找出来。然后x轴从左往右按L排序,再然后就是所谓的贪心 把那些互相重叠的区间去掉即可了区间也就是雷达;*/ /* 3 -3 1 2 -3 2 2 1 Case ... -1; */ //按R进行从左到右排序 #include<iostream> #include<cstdio> #include<cstring> #include<cmath> #include<algorithm> using namespace std; struct node { double L,R; } p[1001]; int cmp(node p1,node p2) { return p1.R<p2.R; } int main() { int n,d,num=0; while(cin>>n>>d) { num++; if(n==0&&d==0) break; int flag=0; for(int i=0; i<n; i++) { int u,v; cin>>u>>v; if(d<v) { flag=1; } else if(flag==0) { p[i].L=u-sqrt(d*d-v*v); p[i].R=sqrt(d*d-v*v)+u; } } if(flag) { printf("Case %d: -1 ",num); continue; } sort(p,p+n,cmp); double xR=p[0].R; double xL=p[0].L; int sum=1; for(int i=1; i<n; i++) { if(p[i].L<=xR) { } else if(p[i].L>xR) { xR=p[i].R; sum++; } } printf("Case %d: %d ",num,sum); } }