1.spring cloud config是什么
官网:https://cloud.spring.io/spring-cloud-static/spring-cloud-config/2.2.1.RELEASE/reference/html/
Spring Cloud Config项目是一个解决分布式系统的配置管理方案。它包含了Client和Server两个部分,server提供配置文件的存储、以接口的形式将配置文件的内容提供出去,client通过接口获取数据、并依据此数据初始化自己的应用
2.使用
实际使用过程中,我们把配置文件放在git上面,创建一个config的server端,实时同步git上的配置文件。再把配置文件的内容以接口的形式提供除去。client去获取server的配置数据。
2.1在git上创建一个项目
里面放入配置文件,我这里有三个配置文件。注意文件的命名格式。
2.2创建一个模块作为server
2.2.1 依赖
<dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-config-server</artifactId> </dependency>
完整依赖
<dependencies> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-config-server</artifactId> </dependency> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-netflix-eureka-client</artifactId> </dependency> <dependency> <groupId>com.atguigu.springcloud</groupId> <artifactId>cloud-api-commons</artifactId> <version>${project.version}</version> </dependency> <!-- https://mvnrepository.com/artifact/org.springframework.boot/spring-boot-starter-web --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <!-- https://mvnrepository.com/artifact/org.springframework.boot/spring-boot-starter-web --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-actuator</artifactId> </dependency> <!-- https://mvnrepository.com/artifact/org.springframework.boot/spring-boot-devtools --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-devtools</artifactId> <scope>runtime</scope> <optional>true</optional> </dependency> <!-- https://mvnrepository.com/artifact/org.projectlombok/lombok --> <dependency> <groupId>org.projectlombok</groupId> <artifactId>lombok</artifactId> <optional>true</optional> </dependency> <!-- https://mvnrepository.com/artifact/org.springframework.boot/spring-boot-starter-test --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> </dependencies>
2.2.2配置文件
server: port: 3344 eureka: client: service-url: defaultZone: http://eureka7001.com:7001/eureka,http://eureka7002.com:7002/eureka #集群版 spring: application: name: config-server cloud: config: server: git: uri: https://gitee.com/xxx/sprincloud-config.git #git仓库路径 search-paths: - springcloud-config #搜索路径 username: xx password: xxx label: master
cloud.config.server.git:
uri:配置文件项目的git的地址
searc-paths:搜索路径,如下图
username:git用户名
password:git密码
label:分支,如下图
2.2.3主启动类
注解 @EnableConfigServer
package com.atguigu.springcloud; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.cloud.config.server.EnableConfigServer; import org.springframework.cloud.netflix.hystrix.EnableHystrix; @SpringBootApplication @EnableConfigServer public class ConfigCenterMain3344 { public static void main(String[] args) { SpringApplication.run(ConfigCenterMain3344.class,args); } }
2.2.4测试
访问三个配置文件,都访问到了
2.3创建一个client端-也就是需要使用server端配置内容的服务
2.3.1pom文件
<dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-config</artifactId> </dependency>
完整依赖
<dependencies> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-config</artifactId> </dependency> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-netflix-eureka-client</artifactId> </dependency> <dependency> <groupId>com.atguigu.springcloud</groupId> <artifactId>cloud-api-commons</artifactId> <version>${project.version}</version> </dependency> <!-- https://mvnrepository.com/artifact/org.springframework.boot/spring-boot-starter-web --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <!-- https://mvnrepository.com/artifact/org.springframework.boot/spring-boot-starter-web --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-actuator</artifactId> </dependency> <!-- https://mvnrepository.com/artifact/org.springframework.boot/spring-boot-devtools --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-devtools</artifactId> <scope>runtime</scope> <optional>true</optional> </dependency> <!-- https://mvnrepository.com/artifact/org.projectlombok/lombok --> <dependency> <groupId>org.projectlombok</groupId> <artifactId>lombok</artifactId> <optional>true</optional> </dependency> <!-- https://mvnrepository.com/artifact/org.springframework.boot/spring-boot-starter-test --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> </dependencies>
2.3.2配置文件
注意文件名称为:bootstrap.yml
server: port: 3355 eureka: client: service-url: defaultZone: http://eureka7001.com:7001/eureka,http://eureka7002.com:7002/eureka #集群版 spring: application: name: config-client cloud: config: label: master #分支名称 name: config #配置文件名称 profile: dev #读取配置文件的后缀名称 uri: http://localhost:3344 #这几个组合就是 http://localhost:3344/master/config-dev.yml
cloud.config:
label 分支名称
name 配置文件名称
profile 配置文件后缀名称
uri:server地址
这几个配置合起来就是: http://localhost:3344/master/config-dev.yml
2.3.3 主启动类
package com.atguigu.springcloud; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.cloud.netflix.eureka.EnableEurekaClient; @SpringBootApplication @EnableEurekaClient public class ConfigCenterMain3355 { public static void main(String[] args) { SpringApplication.run(ConfigCenterMain3355.class,args); } }
2.3.4 业务类
package com.atguigu.springcloud.controller; import com.atguigu.springcloud.entities.CommonResult; import com.atguigu.springcloud.entities.Payment; import lombok.extern.slf4j.Slf4j; import org.springframework.beans.factory.annotation.Value; import org.springframework.cloud.client.ServiceInstance; import org.springframework.cloud.client.discovery.DiscoveryClient; import org.springframework.cloud.context.config.annotation.RefreshScope; import org.springframework.web.bind.annotation.*; import javax.annotation.Resource; import java.util.List; import java.util.concurrent.TimeUnit; @RestController @Slf4j public class PaymentController { @Value("${config.info}") private String configInfo; //这里config.info是读取配置中心的配置 @GetMapping("/configInfo") public String getConfigInfo(){ return configInfo; } }
2.3.5测试
表示配置读取成功
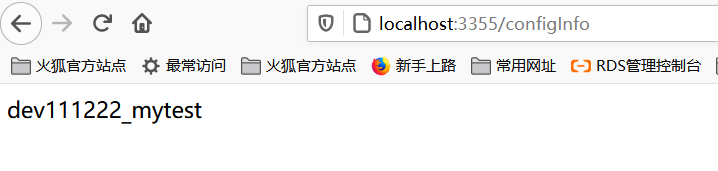
2.4若git上配置文件发生变化
2.4.1在git上修改config-dev的内容
2.4.2访问server
自动完成同步
2.4.3访问client
没有自动同步,只有重启client才完成同步
2.5自动同步配置
2.5.1在client加入依赖,加入actuator监控
前面已经添加了
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-actuator</artifactId> </dependency>
2.5.2配置文件
加入以下配置,暴露监控端点
management:
endpoints:
web:
exposure:
include: "*"
完整
server: port: 3355 eureka: client: service-url: defaultZone: http://eureka7001.com:7001/eureka,http://eureka7002.com:7002/eureka #集群版 spring: application: name: config-client cloud: config: label: master #分支名称 name: config #配置文件名称 profile: dev #读取配置文件的后缀名称 uri: http://localhost:3344 #这几个组合就是 http://localhost:3344/master/config-dev.yml #暴露监控端点 management: endpoints: web: exposure: include: "*"
2.5.3在业务类加入注解@RefreshScope - 刷新配置
package com.atguigu.springcloud.controller; import com.atguigu.springcloud.entities.CommonResult; import com.atguigu.springcloud.entities.Payment; import lombok.extern.slf4j.Slf4j; import org.springframework.beans.factory.annotation.Value; import org.springframework.cloud.client.ServiceInstance; import org.springframework.cloud.client.discovery.DiscoveryClient; import org.springframework.cloud.context.config.annotation.RefreshScope; import org.springframework.web.bind.annotation.*; import javax.annotation.Resource; import java.util.List; import java.util.concurrent.TimeUnit; @RestController @Slf4j @RefreshScope //刷新配置 public class PaymentController { @Value("${config.info}") private String configInfo; @GetMapping("/configInfo") public String getConfigInfo(){ return configInfo; } }
2.5.4在修改配置文件后,需向client发送一个POST请求
http://localhost:3355/actuator/refresh
2.5.5测试
修改配置文件
发送post请求
访问server,配置内容已更新
访问client,配置内容已更新
2.6还存在的缺陷
每次修改配置都需要向client发送post请求,如果存在多个client,就比较麻烦。这里就用到spring cloud bus。config和bus是相互配合的好搭档,一般都是一起使用。
3.使用spring cloud bus
3.1基本说明
Spring Cloud Bus 对自己的定位是 Spring Cloud 体系内的消息总线,使用 message broker 来连接分布式系统的所有节点。
bus有两种通知方式
方式1:
bus通知一个client,再有client把消息通知给其他client
方式2:
bus通知config的server端,再由server端通知client
方式二的架构显然更加合适,方式一不适合的原因如下:
打破了微服务的职责单一性,因为微服务本身是业务模块,它本不应该承担配置刷新职责
破坏了微服务各节点的对等性
有一定的局限性。例如,微服务在迁移时,它的网络地址常常会发生变化,此时如果想要做到自动刷新,那就会增加更多的修改
所以我们采取方式二
3.1环境准备
spring cloud bus不需具备Rabbitmq或者kafka
RabbitMq安装:https://www.cnblogs.com/jthr/p/13786746.html
3.2修改cloud-config-center-3344
3.2.1 添加依赖
<dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-bus-amqp</artifactId> </dependency>
完整
<dependencies> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-config-server</artifactId> </dependency> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-bus-amqp</artifactId> </dependency> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-netflix-eureka-client</artifactId> </dependency> <dependency> <groupId>com.atguigu.springcloud</groupId> <artifactId>cloud-api-commons</artifactId> <version>${project.version}</version> </dependency> <!-- https://mvnrepository.com/artifact/org.springframework.boot/spring-boot-starter-web --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <!-- https://mvnrepository.com/artifact/org.springframework.boot/spring-boot-starter-web --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-actuator</artifactId> </dependency> <!-- https://mvnrepository.com/artifact/org.springframework.boot/spring-boot-devtools --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-devtools</artifactId> <scope>runtime</scope> <optional>true</optional> </dependency> <!-- https://mvnrepository.com/artifact/org.projectlombok/lombok --> <dependency> <groupId>org.projectlombok</groupId> <artifactId>lombok</artifactId> <optional>true</optional> </dependency> <!-- https://mvnrepository.com/artifact/org.springframework.boot/spring-boot-starter-test --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> </dependencies>
3.2.2配置文件添加
rabbitmq: host: localhost #rabbitmq地址 port: 5672 #端口 username: guest #账号 password: guest #密码 management: #暴露bus刷新的端点 endpoints: web: exposure: include: bus-refresh
完整
server: port: 3344 eureka: client: service-url: defaultZone: http://eureka7001.com:7001/eureka,http://eureka7002.com:7002/eureka #集群版 spring: application: name: config-server cloud: config: server: git: uri: https://gitee.com/xxx/sprincloud-config.git #git仓库路径 search-paths: - springcloud-config #搜索路径 username: xxx password: xxx label: master rabbitmq: host: localhost #rabbitmq地址 port: 5672 #端口 username: guest #账号 password: guest #密码 management: #暴露bus刷新的端点 endpoints: web: exposure: include: bus-refresh
3.3修改cloud-config-client-3355
3.3.1添加依赖
<dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-bus-amqp</artifactId> </dependency>
完整
<dependencies> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-config</artifactId> </dependency> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-bus-amqp</artifactId> </dependency> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-bus-amqp</artifactId> </dependency> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-netflix-eureka-client</artifactId> </dependency> <dependency> <groupId>com.atguigu.springcloud</groupId> <artifactId>cloud-api-commons</artifactId> <version>${project.version}</version> </dependency> <!-- https://mvnrepository.com/artifact/org.springframework.boot/spring-boot-starter-web --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <!-- https://mvnrepository.com/artifact/org.springframework.boot/spring-boot-starter-web --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-actuator</artifactId> </dependency> <!-- https://mvnrepository.com/artifact/org.springframework.boot/spring-boot-devtools --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-devtools</artifactId> <scope>runtime</scope> <optional>true</optional> </dependency> <!-- https://mvnrepository.com/artifact/org.projectlombok/lombok --> <dependency> <groupId>org.projectlombok</groupId> <artifactId>lombok</artifactId> <optional>true</optional> </dependency> <!-- https://mvnrepository.com/artifact/org.springframework.boot/spring-boot-starter-test --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> </dependencies>
3.3.2配置文件添加
rabbitmq: host: localhost port: 5672 username: guest password: guest
完整
server: port: 3355 eureka: client: service-url: defaultZone: http://eureka7001.com:7001/eureka,http://eureka7002.com:7002/eureka #集群版 spring: application: name: config-client cloud: config: label: master #分支名称 name: config #配置文件名称 profile: dev #读取配置文件的后缀名称 uri: http://localhost:3344 #这几个组合就是 http://localhost:3344/master/config-dev.yml rabbitmq: host: localhost port: 5672 username: guest password: guest #暴露监控端点 management: endpoints: web: exposure: include: "*"
3.4新建cloud-config-client-3366
前面有了cloud-config-client-3355,这里新建一个3366,这样子就有两个client,演示效果更好。
3366和3355内容除了端口号,其它一模一样
3.4.1新建
3.4.2pom文件依赖
<dependencies> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-config</artifactId> </dependency> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-bus-amqp</artifactId> </dependency> <dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-netflix-eureka-client</artifactId> </dependency> <dependency> <groupId>com.atguigu.springcloud</groupId> <artifactId>cloud-api-commons</artifactId> <version>${project.version}</version> </dependency> <!-- https://mvnrepository.com/artifact/org.springframework.boot/spring-boot-starter-web --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <!-- https://mvnrepository.com/artifact/org.springframework.boot/spring-boot-starter-web --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-actuator</artifactId> </dependency> <!-- https://mvnrepository.com/artifact/org.springframework.boot/spring-boot-devtools --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-devtools</artifactId> <scope>runtime</scope> <optional>true</optional> </dependency> <!-- https://mvnrepository.com/artifact/org.projectlombok/lombok --> <dependency> <groupId>org.projectlombok</groupId> <artifactId>lombok</artifactId> <optional>true</optional> </dependency> <!-- https://mvnrepository.com/artifact/org.springframework.boot/spring-boot-starter-test --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> </dependencies>
3.4.3配置文件
名字:bootstrap.yml
server: port: 3366 eureka: client: service-url: defaultZone: http://eureka7001.com:7001/eureka,http://eureka7002.com:7002/eureka #集群版 spring: application: name: config-client cloud: config: label: master #分支名称 name: config #配置文件名称 profile: dev #读取配置文件的后缀名称 uri: http://localhost:3344 #这几个组合就是 http://localhost:3344/master/config-dev.yml rabbitmq: host: localhost port: 5672 username: guest password: guest #暴露监控端点 management: endpoints: web: exposure: include: "*"
3.4.4主启动类
package com.atguigu.springcloud; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.cloud.netflix.eureka.EnableEurekaClient; @SpringBootApplication @EnableEurekaClient public class ConfigCenterMain3366 { public static void main(String[] args) { SpringApplication.run(ConfigCenterMain3366.class,args); } }
3.4.5业务类
package com.atguigu.springcloud.controller; import lombok.extern.slf4j.Slf4j; import org.springframework.beans.factory.annotation.Value; import org.springframework.cloud.context.config.annotation.RefreshScope; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RestController; @RestController @Slf4j @RefreshScope //刷新配置 public class PaymentController { @Value("${config.info}") private String configInfo; @GetMapping("/configInfo") public String getConfigInfo(){ return configInfo; } }
3.5测试
启动项目
3.5.1访问
访问server3344
访问3355
访问3366
3.5.3修改git上的配置文件
3.5.4发送POST请求
http://localhost:3344/actuator/bus-refresh
3.5.5再次访问
配置内容已经自动更新
3.5.6说明
上面的测试说明,在修改git上配置文件后,只需要发送一次POST请求到server,所有的client会更新配置文件内容。这样子就不用去对每个client端发送POST请求了。
3.6差异化通知
如果我们只需要部分client更新而不是全部client更新,该怎么做,只需要对发送的POST请求进行修改
3.5.1修改git上配置文件
3.5.2发送POST请求
http://localhost:3344/actuator/bus-refresh/config-client:3355
config-client:微服务名称 3355:端口号
3.5.3再次访问
server3344和client3355都更新了,client3366没有更新