声明本文只适合初学者,本人也是刚接触而已,经过一段时间的研究小有收获,特来分享下希望和大家互相交流学习。
首先配置我们的web.xml代码如下:
<filter>
<filter-name>shiroFilter</filter-name>
<filter-class>org.springframework.web.filter.DelegatingFilterProxy</filter-class>
</filter>
<filter-mapping>
<filter-name>shiroFilter</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
}
各部分代码功能上面注释已基本解释了,我要说的是,我们平时有可能比较喜欢使用currUser对象,但是貌似在这里没有办法得到了。其实不然,首先shiro给我们提供的Subject的会话可以满足我们的需求
Session session = subject.getSession();
Session session = subject.getSession(boolean create);
这些方法在概念上等同于HttpServletRequest API。第一个方法会返回Subject的现有会话,或者如果还没有会话,它会创建一个新的并将之返回。
第二个方法接受一个布尔参数,这个参数用于判定会话不存在时是否创建新会话。一旦获得Shiro的会话,你几乎可以像使用HttpSession一样使用它。Shiro团队觉得对于Java开发者,HttpSession API用起来太舒服了,所以我们保留了它的很多感觉。当然,最大的不同在于,你可以在任何应用中使用Shiro会话,不仅限于Web应用。因此你可以再验证登陆里写这样的一句话来完成我们的代码转换SecurityUtils.getSubject().getSession().setAttribute("currUser", user);注意在异常处理里需要移除此currUser。当然官方推荐使用Subject
最后就是我们的Controller了。在这里我介绍登陆和退出
@RequestMapping("/user/login")
@RequestMapping("/user/exit")
好了,这样基本算是完成任务了,接下来就是页面上的操作了。为此shiro还提供了相应的标签,在这里我就照搬官方的了,因为这个实在简单,大家一看就明白
引用<%@
验证当前用户是否为“访客”,即未认证(包含未记住)的用户
- <shiro:guest>
-
Hi there! Please <a href="login.jsp">Login</a> or <ahref="signup.jsp">Signup</a> today! - </shiro:guest>
user标签
认证通过或已记住的用户
- <shiro:user>
-
Welcome back John! Not John? Click <a href="login.jsp">here<a> to login. - </shiro:user>
authenticated标签
已认证通过的用户。不包含已记住的用户,这是与user标签的区别所在。
- <shiro:authenticated>
-
<a href="updateAccount.jsp">Update your </a>.contact information - </shiro:authenticated>
notAuthenticated标签
未认证通过用户,与authenticated标签相对应。与guest标签的区别是,该标签包含已记住用户。
- <shiro:notAuthenticated>
-
Please <a href="login.jsp">login</a> in order to update your credit card information. - </shiro:notAuthenticated>
principal 标签
输出当前用户信息,通常为登录帐号信息
- Hello,
<shiro:principal/>, how are you today?
hasRole标签
验证当前用户是否属于该角色
- <shiro:hasRole
name="administrator"> -
<a href="admin.jsp">Administer the </a>system - </shiro:hasRole>
lacksRole标签
与hasRole标签逻辑相反,当用户不属于该角色时验证通过
- <shiro:lacksRole
name="administrator"> -
Sorry, you are not allowed to administer the system. - </shiro:lacksRole>
hasAnyRole标签
验证当前用户是否属于以下任意一个角色。
- <shiro:hasAnyRoles
name="developer, project >manager, administrator" -
You are either a developer, project manager, or administrator. - </shiro:lacksRole>
hasPermission标签
验证当前用户是否拥有制定权限
- <shiro:hasPermission
name="user:create"> -
<a href="createUser.jsp">Create a </a>new User - </shiro:hasPermission>
lacksPermission标签
与hasPermission标签逻辑相反,当前用户没有制定权限时,验证通过
Xml代码
- <shiro:hasPermission
name="user:create"> -
<a href="createUser.jsp">Create a </a>new User - </shiro:hasPermission>
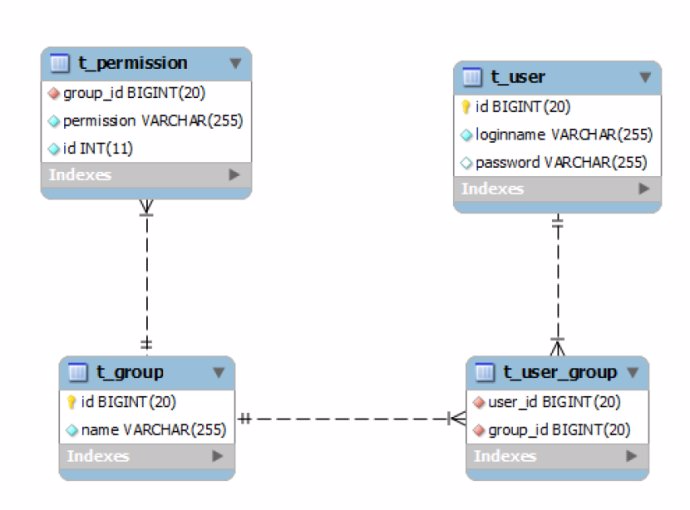
user == subject:用户,group(role):角色
permission:权限(拥有权限比较细的情况,一般只要user和group就满足要求了)
最后 提一下jar包,别弄错了。是shiro-all.jar。可以从官网下载http://shiro.apache.org/download.html