# 定时器
* * * * * cd /home/wwwroot/default/dexin/dragon && /usr/bin/php think order --option 1 >> /tmp/dexin_timer.log 2>&1
* * * * * cd /home/wwwroot/default/dexin/dragon && /usr/bin/php think order --option 2 >> /tmp/dexin_timer.log 2>&1
* * * * * cd /home/wwwroot/default/dexin/dragon && /usr/bin/php think order --option 3 >> /tmp/dexin_timer.log 2>&1
* * * * * cd /home/wwwroot/default/dexin/dragon && /usr/bin/php think order --option 4 >> /tmp/dexin_timer.log 2>&1
<?php
namespace appcroncommand;
use appcommonmodelOrderModel;
use thinkconsoleCommand;
use thinkconsoleInput;
use thinkconsoleinputArgument;
use thinkconsoleinputOption;
use thinkconsoleOutput;
use thinkDb;
class OrderTimer extends Command
{
protected function configure()
{
$this->setName('order')
->addOption('option', null, Option::VALUE_REQUIRED, 'Option todo')
->setDescription('Auto Deal Order');
}
protected function execute(Input $input, Output $output)
{
$option = $input->getOption('option');
if (!$option) {
return $output->writeln("Please Input Option");
}
switch ($option) {
case 1:
case 'cancel_not_payed_order':
$count = $this->cancel_not_payed_order();
return $output->writeln(date("Y-m-d H:i:s")." Deal Not Payed Order Num:".$count);
break;
case 2:
case 'clear_invalid_order':
$count = $this->clear_invalid_order();
return $output->writeln(date("Y-m-d H:i:s")." Deal Not Invalid Order Num:".$count);
break;
case 3:
case 'auto_done_order':
$count = $this->auto_done_order();
return $output->writeln(date("Y-m-d H:i:s")." Deal Done Order Num:".$count);
break;
case 4:
case 'auto_done_not_receive_order':
$count = $this->auto_done_not_receive_order();
return $output->writeln(date("Y-m-d H:i:s")." Deal Done Not Receive Order Num:".$count);
break;
default:
return $output->writeln("Invalid Option");
break;
}
}
// 清理未支付订单 订单为状态为0
public function clear_invalid_order() {
// 订单超过1个小时未生成,将清理
$order_list = Db::name('order')
->where('status',OrderModel::ORDER_ADD)
->where('add_time','lt',time()-60*60)
->select();
// 遍历清理
Db::startTrans();
$error = 0;
foreach ($order_list as $k => $v) {
// 删除订单商品数据
$r_del = Db::name('order_product')
->where('order_num',$v['order_num'])
->delete();
if (!$r_del && $r_del !== 0) {
$error ++ ;
}
// 删除订单
$r_del = Db::name('order')
->where('id',$v['id'])
->delete();
if (!$r_del && $r_del !== 0) {
$error ++ ;
}
}
if ($error == 0) {
Db::commit();
return count($order_list);
} else {
Db::rollback();
return -1;
}
}
// 清理未支付订单 订单为状态为1
public function cancel_not_payed_order() {
// 订单超过3个小时未支付,将取消
$order_list = Db::name('order')
->where('status',OrderModel::ORDER_CREATE)
->where('create_time','lt',time()-3*60*60)
->select();
// 遍历处理
Db::startTrans();
$error = 0;
foreach ($order_list as $k => $v) {
// 设置订单为取消
$update_data = [
'cancel_ok_time' => time(),
'status' => OrderModel::ORDER_CANCEL
];
$r_update = Db::name('order')
->where('id',$v['id'])
->data($update_data)
->update();
if (!$r_update && $r_update !== 0) {
$error ++ ;
}
}
if ($error == 0) {
Db::commit();
return count($order_list);
} else {
Db::rollback();
return -1;
}
}
// 确认收货订单自动完成 订单为状态为7
public function auto_done_order() {
// 确认收货状态订单,时间超过7天,将自动完成
$order_list = Db::name('order')
->where('status',OrderModel::ORDER_RECEIVE)
->where('receive_ok_time','lt',time()-7*24*60*60)
->select();
// 遍历处理
Db::startTrans();
$error = 0;
foreach ($order_list as $k => $v) {
// 设置订单完成
$update_data = [
'status' => OrderModel::ORDER_DONE
];
$r_update = Db::name('order')
->where('id',$v['id'])
->data($update_data)
->update();
if (!$r_update && $r_update !== 0) {
$error ++ ;
}
}
if ($error == 0) {
Db::commit();
return count($order_list);
} else {
Db::rollback();
return -1;
}
}
// 未收货订单,超过14天,将自动完成,订单状态为6
public function auto_done_not_receive_order() {
$order_list = Db::name('order')
->where('status',OrderModel::ORDER_SEND)
->where('send_time','lt',time()-14*24*60*60)
->select();
// 遍历处理
Db::startTrans();
$error = 0;
foreach ($order_list as $k => $v) {
// 设置订单完成
$update_data = [
'status' => OrderModel::ORDER_DONE
];
$r_update = Db::name('order')
->where('id',$v['id'])
->data($update_data)
->update();
if (!$r_update && $r_update !== 0) {
$error ++ ;
}
}
if ($error == 0) {
Db::commit();
return count($order_list);
} else {
Db::rollback();
return -1;
}
}
}
<?php
// +----------------------------------------------------------------------
// | ThinkPHP [ WE CAN DO IT JUST THINK ]
// +----------------------------------------------------------------------
// | Copyright (c) 2006-2016 http://thinkphp.cn All rights reserved.
// +----------------------------------------------------------------------
// | Licensed ( http://www.apache.org/licenses/LICENSE-2.0 )
// +----------------------------------------------------------------------
// | Author: yunwuxin <448901948@qq.com>
// +----------------------------------------------------------------------
return [
'appcroncommandOrderTimer',
'appcroncommandCommandTest',
];
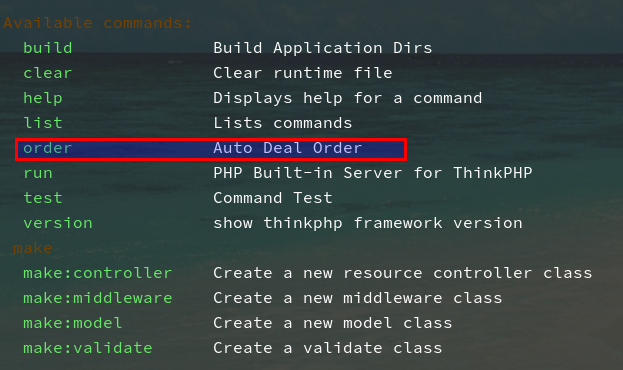
# tail -n 10 /tmp/dexin_timer.log
2018-09-19 14:04:01 Deal Done Not Receive Order Num:0
2018-09-19 14:04:01 Deal Done Order Num:0
2018-09-19 14:04:01 Deal Not Invalid Order Num:1
2018-09-19 14:04:01 Deal Not Payed Order Num:0