表单相关
固定的几个属性和事件
value: <input>,<textarea>;
checked: <input> typeof checkbox, radio;
selected: <option>;
onChange
: 作用于上面元素;
默认值
render: function() {
return <input type="text" defaultValue="Hello!" />;
}
获取事件触发
handleChange: function(event) {
this.setState({value: event.target.value.substr(0, 140)});
}
给select
定义默认值
//使用selected
<option value="A" selected={true}>Apple</option>
//利用state
var Select = React.createClass({
getInitialState: function() {
return {
value: 'B'
}
},
change: function(event){
this.setState({value: event.target.value});
},
render: function() {
return (
<select onChange={this.change} value={this.state.value} >
<option value="A" >Apple</option>
<option value="B">Banana</option>
<option value="C">Cranberry</option>
</select>
);
}
});
设置select
多选
<select multiple={true} value={['B', 'C']}>
</select>
组件的生命周期
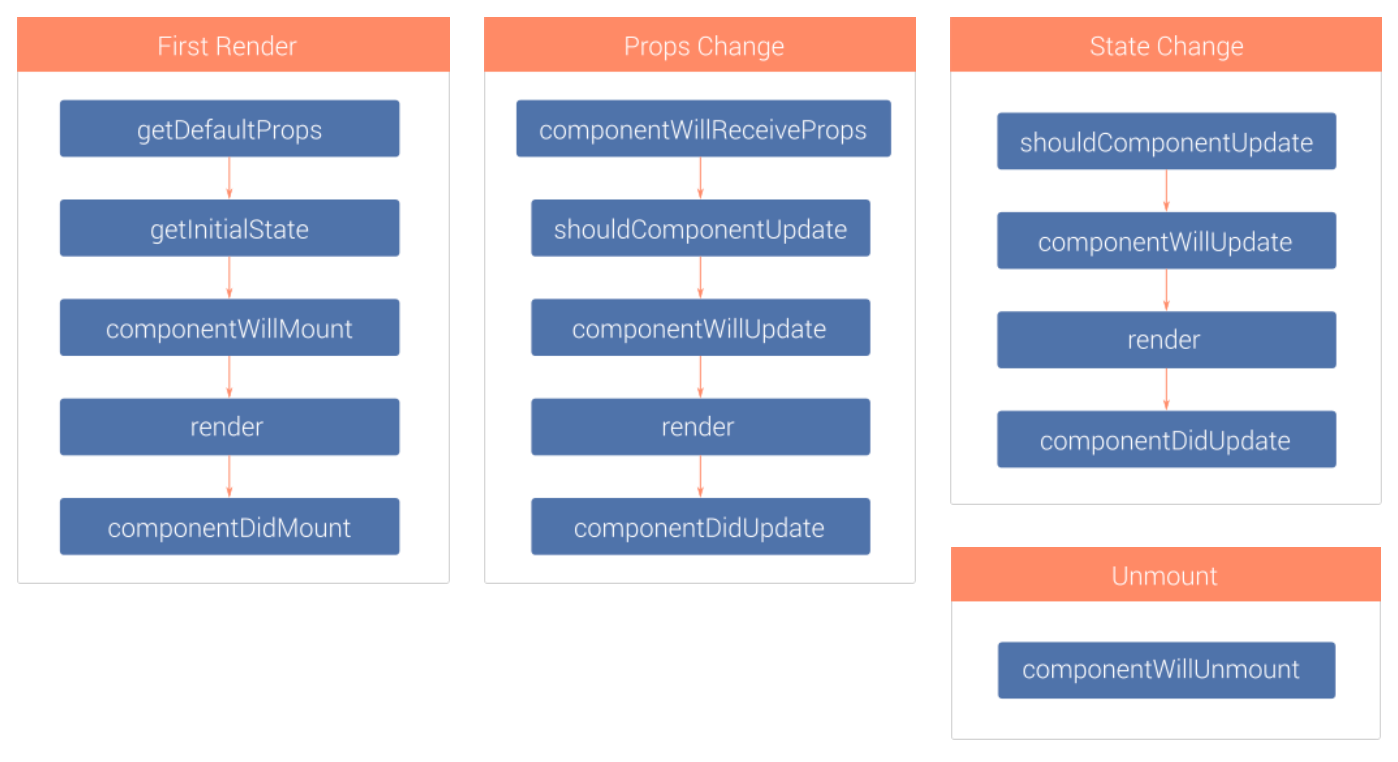
三个状态
Mounting
:已插入真实DOM
;
componentWillMount()
: -React.render
- 在
render
方法执行之前,此时不能获取dom
节点;
getInitialState();
-React.render
render
:-React.render
- 组件初始化渲染和
this.setState({}), this.render(), React.render
时会调用;
- 在修改dom节点的时候也不会调用;
- 注意在执行
this.setState()
时才会重新渲染;
componentDidMount();
-React.render
Updating
:正在被重新渲染;
componentWillReceiveProps(object nextProps)
:-this.setProps
shouldComponentUpdate(object nextProps, object nextState)
:-this.setProps, this.setState
- 组件判断是否重新渲染时调用;
- 如果有定义,必须返回布尔值以判断时候继续调用
update
方法;
componentWillUpdate(object nextProps, object nextState)
:-(this.setProps, this.setState && should !== false) || this. forceUpdate
componentDidUpdate(object prevProps, object prevState)
:-(this.setProps, this.setState && should !== false) || this. forceUpdate
Unmounting
:已移出真实DOM
;
componentWillUnmount();
-React.unmountComponentAtNode
//移除其他组件
mount: function () {
React.render(<Input />, document.getElementById('app'));
},
unmout: function () {
React.unmountComponentAtNode(document.getElementById('app'));
}
//移除自身
remove: function () {
React.unmountComponentAtNode(React.findDOMNode(this).parentNode);
}
强制重新渲染: this.forceUpdate()
混合
var SetIntervalMixin = {
componentWillMount: function() {
this.intervals = [];
},
setInterval: function() {
this.intervals.push(setInterval.apply(null, arguments));
},
componentWillUnmount: function() {
this.intervals.map(clearInterval);
}
};
var TickTock = React.createClass({
mixins: [SetIntervalMixin],
.......
}
Contexts