Lazy Running
Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 524288/524288 K (Java/Others)
Total Submission(s): 101 Accepted Submission(s): 40
Problem Description
In HDU, you have to run along the campus for 24 times, or you will fail in PE. According to the rule, you must keep your speed, and your running distance should not be less thanK meters.
There are 4 checkpoints in the campus, indexed as p1,p2,p3 and p4. Every time you pass a checkpoint, you should swipe your card, then the distance between this checkpoint and the last checkpoint you passed will be added to your total distance.
The system regards these 4 checkpoints as a circle. When you are at checkpoint pi, you can just run to pi−1 or pi+1(p1 is also next to p4). You can run more distance between two adjacent checkpoints, but only the distance saved at the system will be counted.
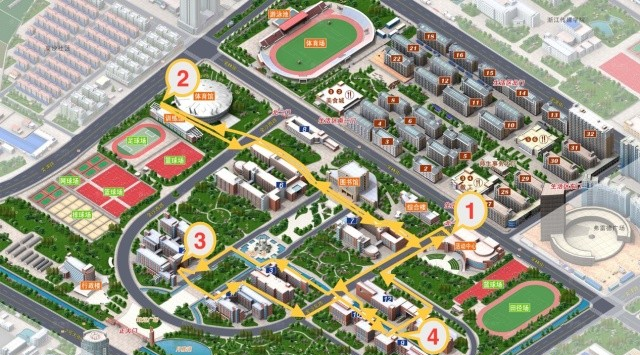
Checkpoint p2 is the nearest to the dormitory, Little Q always starts and ends running at this checkpoint. Please write a program to help Little Q find the shortest path whose total distance is not less thanK.
Input
The first line of the input contains an integer T(1≤T≤15), denoting the number of test cases.
In each test case, there are 5 integers K,d1,2,d2,3,d3,4,d4,1(1≤K≤1018,1≤d≤30000), denoting the required distance and the distance between every two adjacent checkpoints.
Output
For each test case, print a single line containing an integer, denoting the minimum distance.
Sample Input
Sample Output
Source
2017 Multi-University Training Contest - Team 4
比赛的时候就是蠢……
当时只剩下十几分钟的时候看到这题的……其实很简单的一道最短路,前提是想到了……
题目要求是只有四个点,然后连边成正方形,问从2号点出发,再回到2号点且走过的总距离大于K的最短的路径是多少。对于这个,我们考虑如果存在一条合法路径,那么我再走2*w也一定是合法路径,其中w表示与2相连的某条边的长度。即回到2之后再出去再回来,这样的路径一定合法。那么,如果走了某条路径回到了2,然后总距离不够的话,我们只需要加上几个2*w使得最后结果大于等于K即可。
那么如何使这个结果最小呢?我们考虑设置一个数组d[x][p]表示从2出发最后到达x点且费用对2*w取模结果为p时的最小花费。这样子原本的一个点就可以拆成2*w个点,这样跑一遍dijkstra即可求出d数组。之后,对于每一个对2*w取模后的数值,我们都可以计算把它补到大于K且距离K最近的花费,再在这些花费中取一个最小的即可。那么,这样子考虑为什么可以包含全部的而且最优的解呢?因为我们最后补的是2*w或者它的倍数,然后我把对2*w取模后的所有取值的最小花费都计算了一次,这样子得出来的最小花费一定包含所有的情况,即2*w的所有剩余系都被包括了,所以可以保证正确性。具体代码如下:
转载于http://blog.csdn.net/yasola/article/details/76684704;
#include <iostream> #include <algorithm> #include <cstdio> #include <cstring> #include <cstdlib> #include <cmath> #include <ctime> #include <vector> #include <queue> #include <stack> #include <deque> #include <string> #include <map> #include <set> #include <list> using namespace std; #define INF 0x3f3f3f3f3f3f3f3f #define LL long long #define fi first #define se second #define mem(a,b) memset((a),(b),sizeof(a)) const int MAXN=4+3; const int MAXM=60000+3; struct Node { int p; LL dis; Node(int p, LL d):p(p), dis(d){} }; LL K, G[MAXN][MAXN], m, ans; bool vis[MAXN][MAXM]; LL dist[MAXN][MAXM];//从1开始到达i,模m等于j的最短路 void spfa(int s) { queue<Node> que; mem(vis, 0); mem(dist, 0x3f); que.push(Node(1, 0)); dist[1][0]=0; vis[1][0]=true; while(!que.empty()) { int u=que.front().p; LL now_dis=que.front().dis; vis[u][now_dis%m]=false; que.pop(); for(int i=-1;i<2;i+=2) { int v=(u+i+4)%4; LL next_dis=now_dis+G[u][v], next_m=next_dis%m; if(v==s)//形成环,更行答案 { if(next_dis<K) ans=min(ans, next_dis+((K-next_dis-1)/m+1)*m); else ans=min(ans, next_dis); } if(dist[v][next_m]>next_dis) { dist[v][next_m]=next_dis; if(!vis[v][next_m]) { que.push(Node(v, next_dis)); vis[v][next_m]=true; } } } } } int main() { int T_T; scanf("%d", &T_T); while(T_T--) { scanf("%lld", &K); for(int i=0;i<4;++i) { scanf("%lld", &G[i][(i+1)%4]); G[(i+1)%4][i]=G[i][(i+1)%4]; } m=2*min(G[1][0], G[1][2]);//最小环 ans=((K-1)/m+1)*m;//只使用最短的回路 spfa(1); printf("%lld ", ans); } return 0; }