TypeScript函数类型
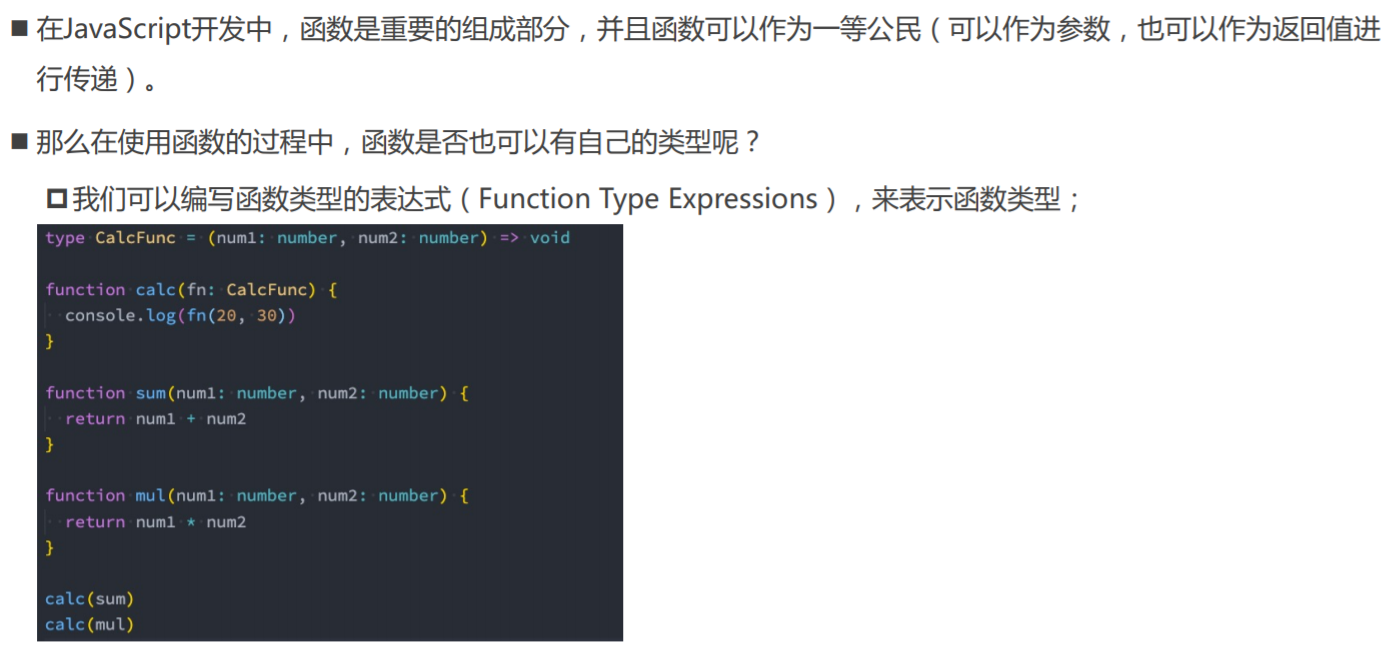
TypeScript函数类型解析
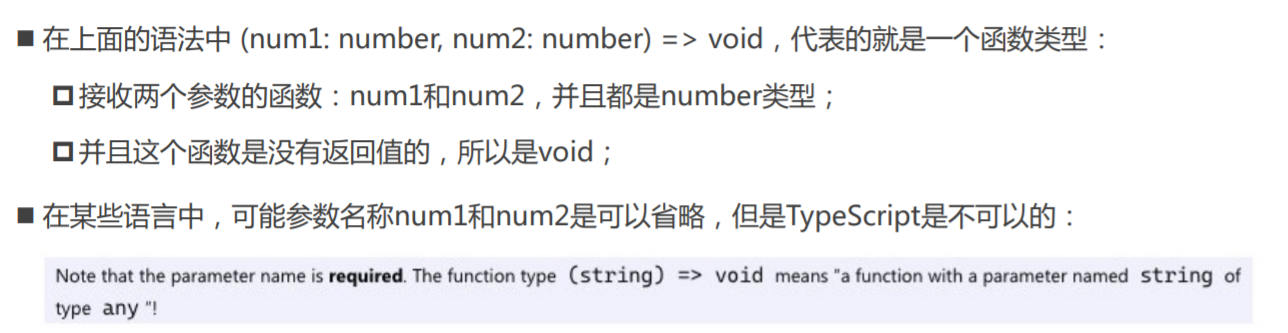
参数的可选类型
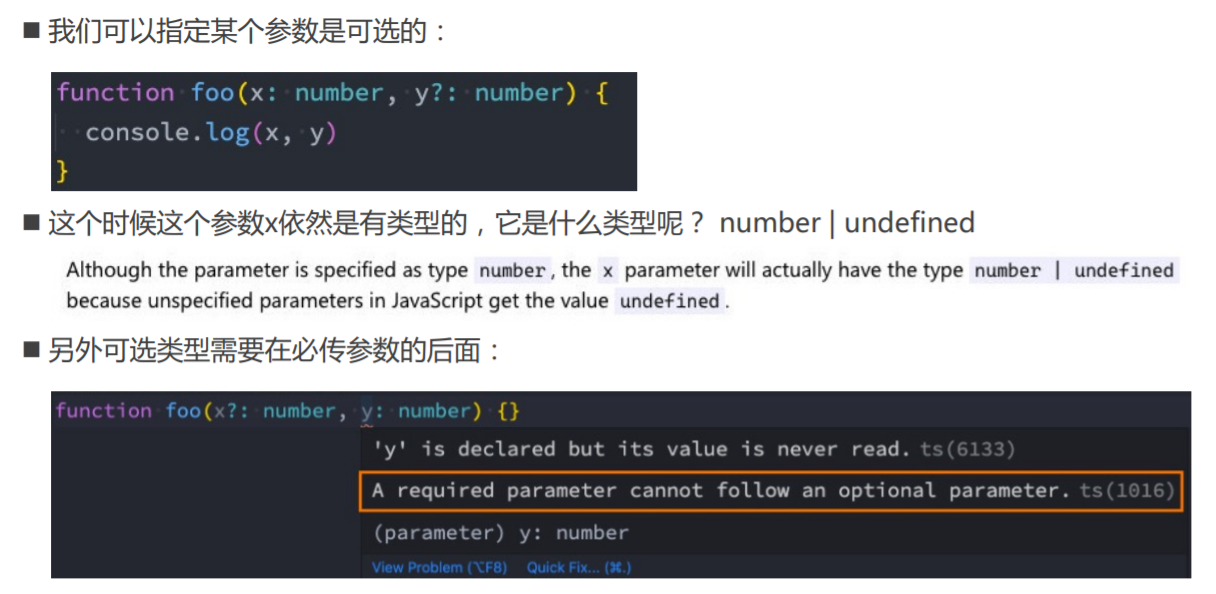
默认参数
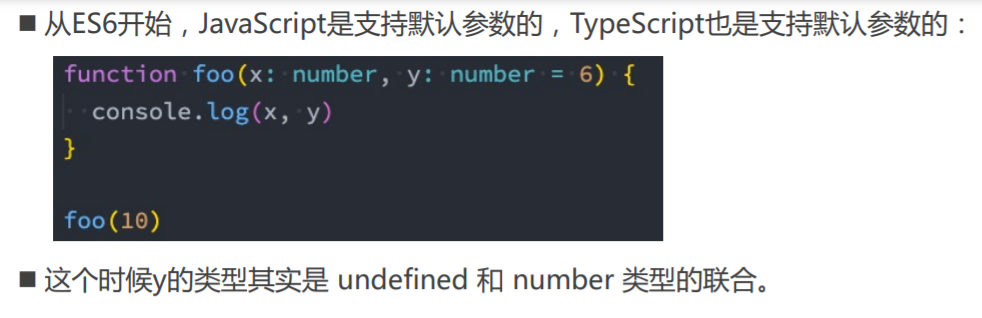
剩余参数
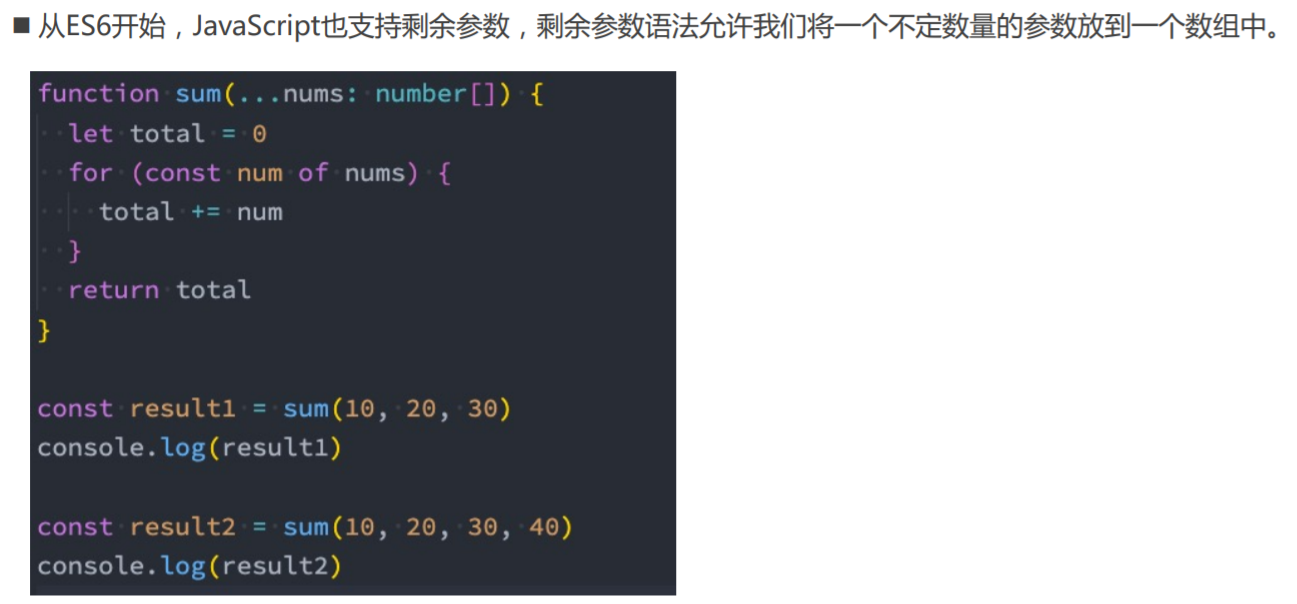
可推导的this类型
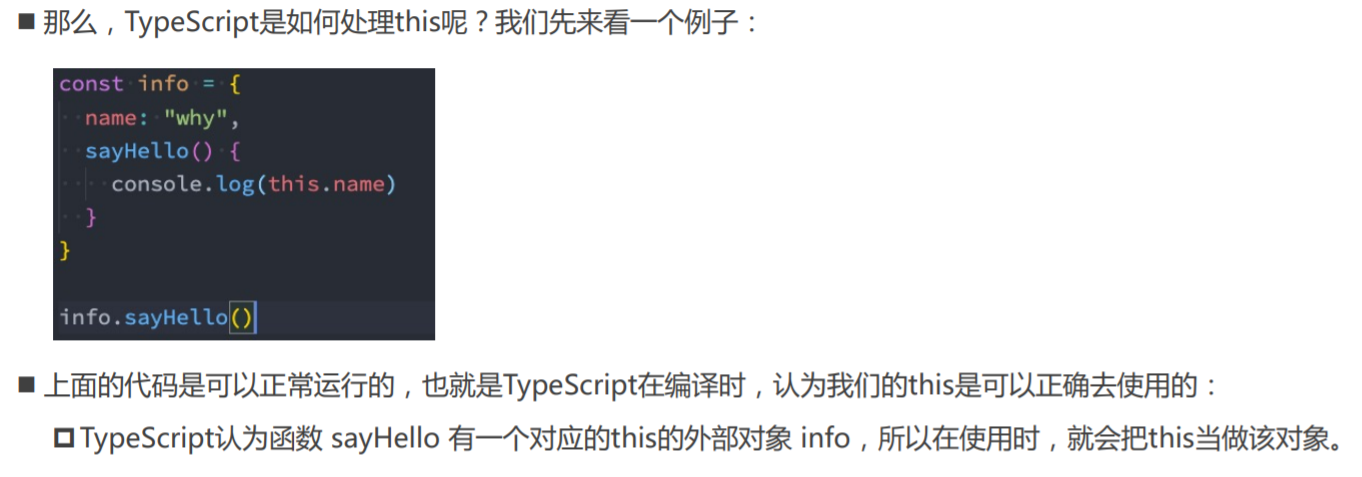
不确定的this类型
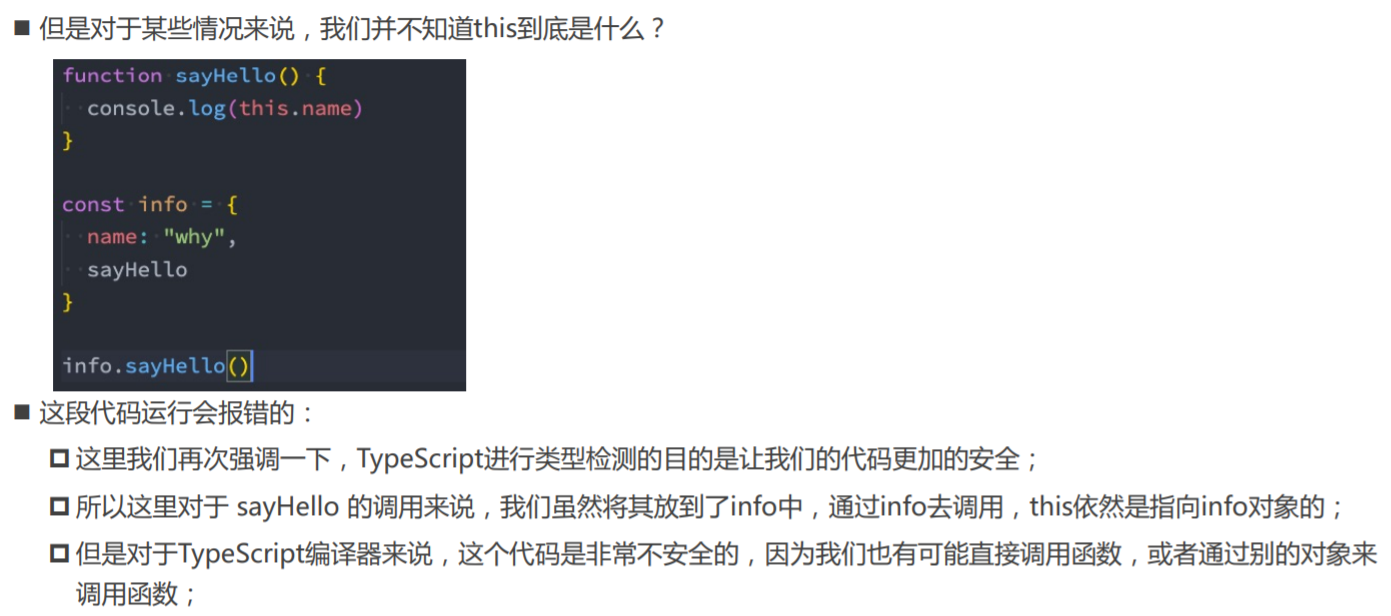
指定this的类型
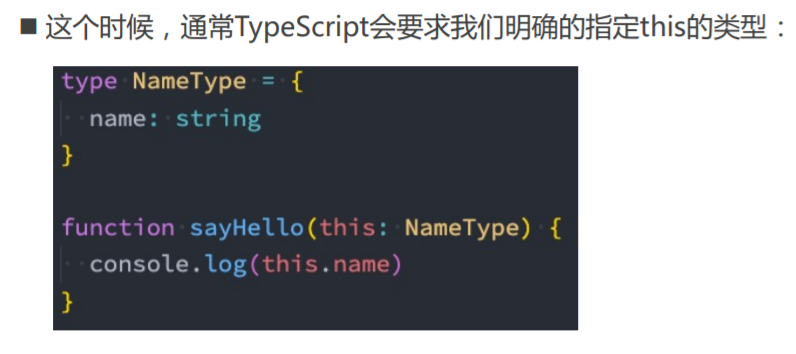
函数的重载
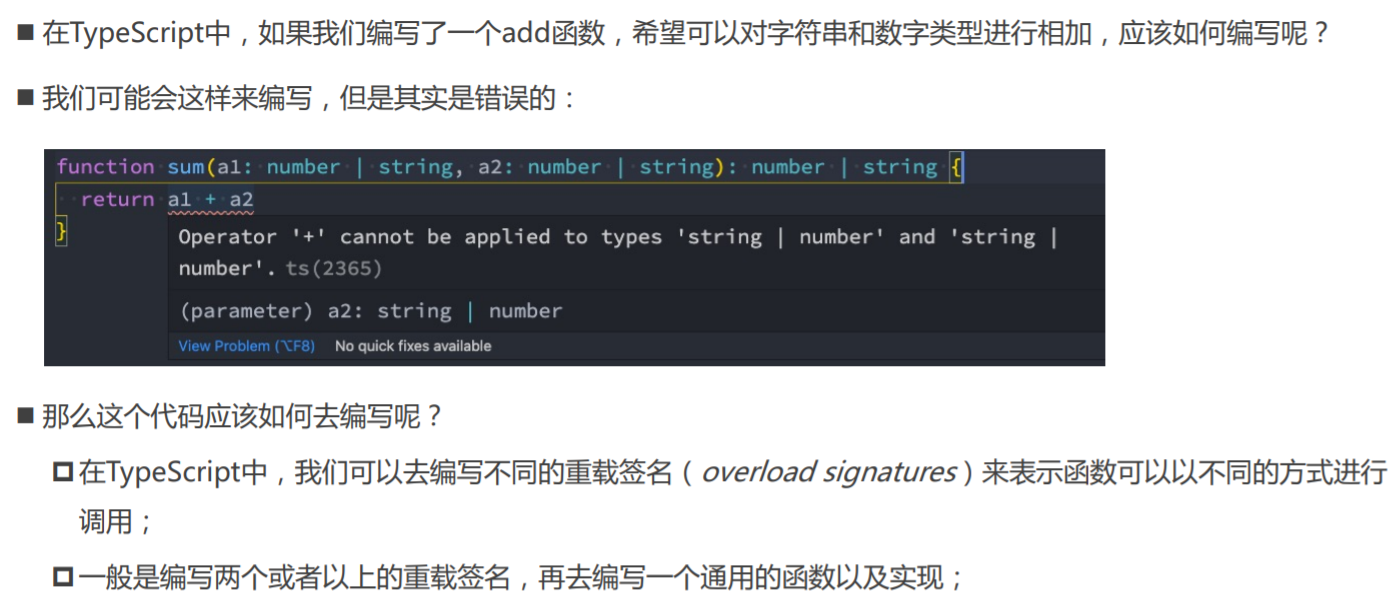
sum函数的重载
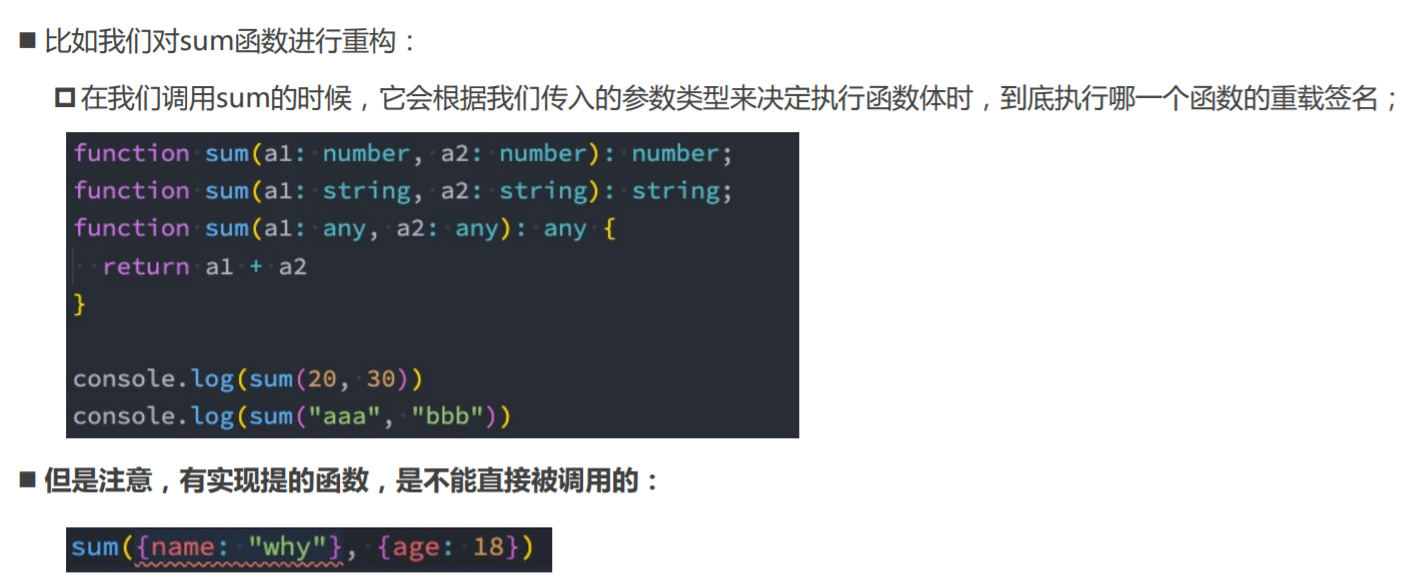
联合类型和重载
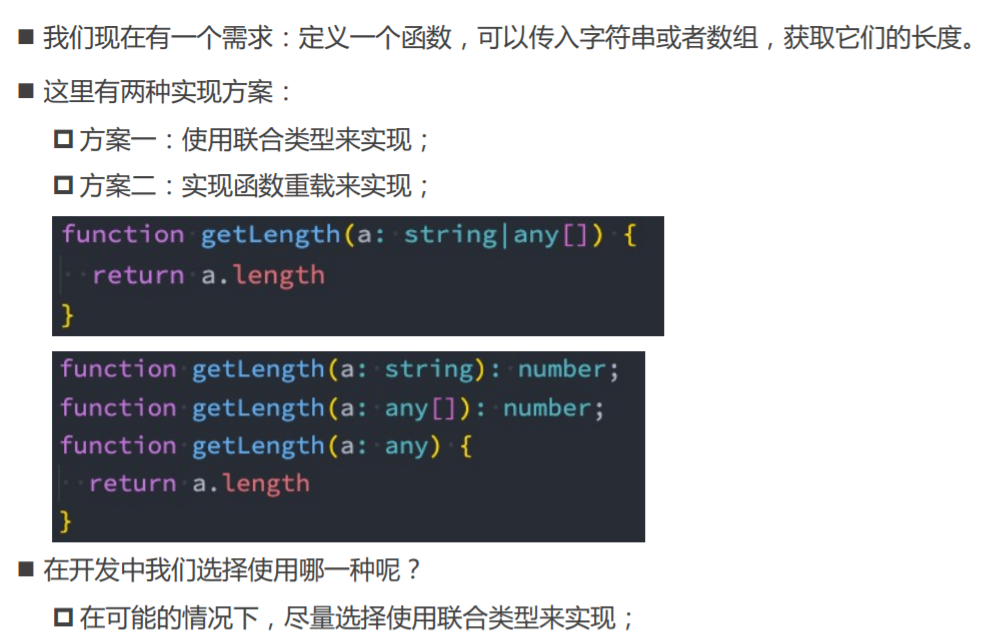
01_函数的类型.ts
// 1.函数作为参数时, 在参数中如何编写类型
function foo() {}
type FooFnType = () => void
function bar(fn: FooFnType) {
fn()
}
bar(foo)
// 2.定义常量时, 编写函数的类型
// 【返回类型为void时,不报错,因为这意味着函数可以返回任何类型。】
type AddFnType = (num1: number, num2: number) => number
// 【形参任意】
const add: AddFnType = (a1: number, a2: number) => {
return a1 + a2
}
export {}
02_函数类型的案例.ts
function calc(n1: number, n2: number, fn: (num1: number, num2: number) => number) {
return fn(n1, n2)
}
const result1 = calc(20, 30, function (a1, a2) {
return a1 + a2
})
console.log(result1)
const result2 = calc(20, 30, function (a1, a2) {
return a1 * a2
})
console.log(result2)
// 我的改造
type calcFnType = (n1: number, n2: number) => number
function calc2(n1: number, n2: number, fn: calcFnType) {
return fn(n1, n2)
}
const sum = (a1: number, a2: number): number => a1 + a2
const mul = (a1: number, a2: number): number => a1 * a2
const res1 = calc2(10, 20, sum)
const res2 = calc2(10, 30, mul)
console.log(res1, res2)
04_参数的可选类型.ts
// 可选类型是必须写在必选类型的后面的
// y -> undefined | number
function foo(x: number, y?: number) {
console.log(x, y)
}
foo(20, 30)
foo(20)
export {}
05_参数的默认值.ts
// 参数顺序:必传参数 - 有默认值的参数 - 可选参数
function foo(y: number, x: number = 20) {
console.log(x, y)
}
foo(30)
06_函数的剩余参数.ts
// function sum(num1: number, num2: number) {
// return num1 + num2
// }
function sum(initalNum: number, ...nums: number[]) {
let total = initalNum
for (const num of nums) {
total += num
}
return total
}
console.log(sum(20, 30))
console.log(sum(20, 30, 40))
console.log(sum(20, 30, 40, 50))
export {}
07_this的默认推导.ts
// this是可以被推导出来 info对象(TypeScript推导出来)
const info = {
name: 'why',
eating() {
console.log(this.name + ' eating')
},
}
info.eating()
export {}
08_this的不明确类型.ts
type ThisType = { name: string }
// 【这个this参数要放在第一位。】
function eating(this: ThisType, message: string) {
console.log(this.name + ' eating', message)
}
const info = {
name: 'why',
eating: eating,
}
// 隐式绑定
info.eating('哈哈哈')
// 显示绑定
eating.call({ name: 'kobe' }, '呵呵呵')
eating.apply({ name: 'james' }, ['嘿嘿嘿'])
export {}
09_函数的重载(联合类型).ts
/**
* 通过联合类型有两个缺点:
* 1.进行很多的逻辑判断(类型缩小)
* 2.返回值的类型依然是不能确定
*/
// 【联合类型不能使用操作符+,a1、a2表示联合类型,而不是取值为number或string。】
function add(a1: number | string, a2: number | string) {
if (typeof a1 === 'number' && typeof a2 === 'number') {
return a1 + a2
} else if (typeof a1 === 'string' && typeof a2 === 'string') {
return a1 + a2
}
// return a1 + a2;
}
add(10, 20)
10_函数的重载(函数重载).ts
// 函数的重载: 函数的名称相同, 但是参数不同的若干个函数, 就是函数的重载
function add(num1: number, num2: number): number // 没函数体
function add(num1: string, num2: string): string
// 【函数的声明和实现是分开的。】
function add(num1: any, num2: any): any {
if (typeof num1 === 'string' && typeof num2 === 'string') {
return num1.length + num2.length
}
return num1 + num2
}
const result = add(20, 30)
const result2 = add('abc', 'cba')
console.log(result)
console.log(result2)
// 在函数的重载中, 实现函数是不能直接被调用的
// add({name: "why"}, {age: 18})
export {}
11_函数的重载练习.ts
// 实现方式一: 联合类型
function getLength(args: string | any[]) {
return args.length
}
console.log(getLength('abc'))
console.log(getLength([123, 321, 123]))
// 实现方式二: 函数的重载
// function getLength(args: string): number;
// function getLength(args: any[]): number;
// function getLength(args: any): number {
// return args.length
// }
// console.log(getLength("abc"))
// console.log(getLength([123, 321, 123]))