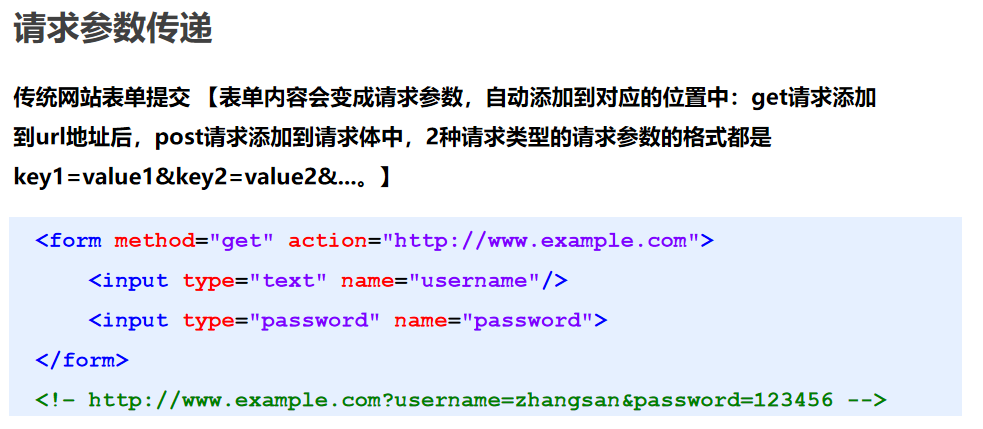
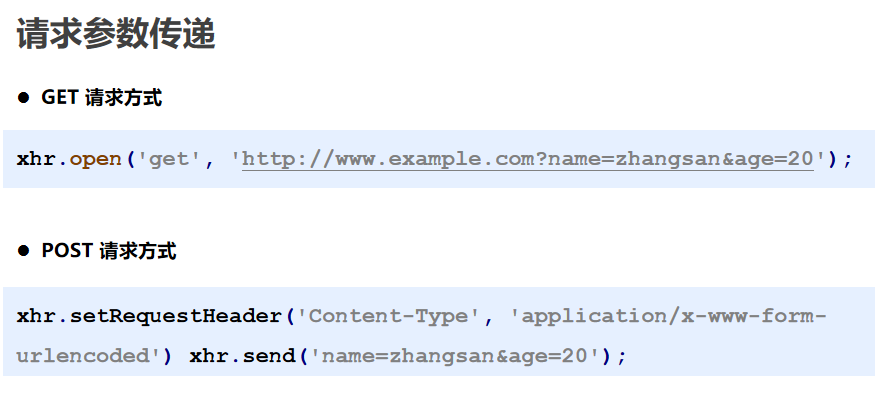
03.传递get请求参数.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
</head>
<body>
<p>
<input type="text" id="username">
</p>
<p>
<input type="text" id="age">
</p>
<p>
<!-- 这里不是使用传统的表单提交,不需要submit提交按钮 -->
<input type="button" value="提交" id="btn">
</p>
<script type="text/javascript">
var btn = document.getElementById('btn'); // 获取按钮元素
var username = document.getElementById('username'); // 获取姓名文本框
var age = document.getElementById('age'); // 获取年龄文本框
// 为按钮添加点击事件
btn.onclick = function() {
var xhr = new XMLHttpRequest(); // 创建ajax对象
var nameValue = username.value;
var ageValue = age.value;
var params = 'username=' + nameValue + '&age=' + ageValue; // 拼接请求参数
xhr.open('get', 'http://localhost:3000/get?' + params); // 配置ajax对象
xhr.send(); // 发送请求
// 获取服务器端响应的数据
xhr.onload = function() {
console.log(xhr.responseText)
}
}
// 补充写法
document.getElementById('btn').onclick = () => {
let unameValue = document.querySelector('#username').value
let ageValue = document.querySelector('#age').value
let xhr = new XMLHttpRequest()
xhr.open('get', `http://localhost:3000/get?uname=${unameValue}&age=${ageValue}`)
xhr.send()
xhr.onload = function() {
console.log(xhr.responseText) // {"uname":"111","age":"222"}
}
}
</script>
</body>
</html>
04.传递post请求参数.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
</head>
<body>
<p>
<input type="text" id="username">
</p>
<p>
<input type="text" id="age">
</p>
<p>
<input type="button" value="提交" id="btn">
</p>
<script type="text/javascript">
// 表单提交,可以在Headers的 Form Data 中看提交数据
// 获取按钮元素
var btn = document.getElementById('btn');
// 获取姓名文本框
var username = document.getElementById('username');
// 获取年龄文本框
var age = document.getElementById('age');
// 为按钮添加点击事件
btn.onclick = function() {
// 创建ajax对象
var xhr = new XMLHttpRequest();
// 获取用户在文本框中输入的值
var nameValue = username.value;
var ageValue = age.value;
// 拼接请求参数
var params = 'username=' + nameValue + '&age=' + ageValue;
// 配置ajax对象
xhr.open('post', 'http://localhost:3000/post');
// 设置请求参数格式的类型(post请求必须要设置) 【如果参数是key1=value1&key2=value2这种类型,则 Content-Type 的 值 就是 'application/x-www-form-urlencoded'】
xhr.setRequestHeader('Content-Type', 'application/x-www-form-urlencoded');
// 发送请求
xhr.send(params);
// 获取服务器端响应的数据
xhr.onload = function() {
console.log(xhr.responseText)
}
}
// 补充
document.getElementById('btn').onclick = () => {
let unameValue = document.querySelector('#username').value
let ageValue = document.querySelector('#age').value
let xhr = new XMLHttpRequest()
xhr.open('post', `http://localhost:3000/post`)
xhr.setRequestHeader('Content-Type', 'application/x-www-form-urlencoded')
xhr.send(`uname=${unameValue}&age=${ageValue}`)
xhr.onload = function() {
console.log(xhr.responseText)
}
}
</script>
</body>
</html>